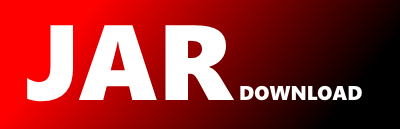
pl.touk.tscreload.Reloadable Maven / Gradle / Ivy
/*
* Copyright 2015 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package pl.touk.tscreload;
import javaslang.*;
import lombok.extern.slf4j.Slf4j;
import pl.touk.tscreload.impl.*;
import java.util.Optional;
@Slf4j
public abstract class Reloadable extends Observable {
private T current;
protected Reloadable(T current) {
this.current = current;
}
public Reloadable map(Function1 f) {
return map((t, prev) -> f.apply(t));
}
public Reloadable map(Function2, U> f) {
Reloadable1 child = new Reloadable1<>(currentValue(), f);
addWeakObserver(child);
return child;
}
protected synchronized void updateCurrentValue(Function1, T> transform) {
try {
T newValue = transform.apply(Optional.of(current));
if (!current.equals(newValue)) {
current = newValue;
notifyObservers(newValue);
}
} catch (Exception ex) {
log.error("Exception while computing reloaded value. Propagation of changes for child nodes will be discarded.", ex);
}
}
public synchronized T currentValue() {
return current;
}
public static Reloadable compose(Reloadable r1,
Reloadable r2,
Function2 f) {
return compose(r1, r2, (p1, p2, prev) -> f.apply(p1, p2));
}
public static Reloadable compose(Reloadable r1,
Reloadable r2,
Function3, U> f) {
Reloadable2 reloadable = new Reloadable2<>(
r1.currentValue(),
r2.currentValue(),
f);
r1.addWeakObserver(reloadable.observer1);
r2.addWeakObserver(reloadable.observer2);
return reloadable;
}
public static Reloadable compose(Reloadable r1,
Reloadable r2,
Reloadable r3,
Function3 f) {
return compose(r1, r2, r3, (p1, p2, p3, prev) -> f.apply(p1, p2, p3));
}
public static Reloadable compose(Reloadable r1,
Reloadable r2,
Reloadable r3,
Function4, U> f) {
Reloadable3 reloadable = new Reloadable3<>(
r1.currentValue(),
r2.currentValue(),
r3.currentValue(),
f);
r1.addWeakObserver(reloadable.observer1);
r2.addWeakObserver(reloadable.observer2);
r3.addWeakObserver(reloadable.observer3);
return reloadable;
}
public static Reloadable compose(Reloadable r1,
Reloadable r2,
Reloadable r3,
Reloadable r4,
Function4 f) {
return compose(r1, r2, r3, r4, (p1, p2, p3, p4, prev) -> f.apply(p1, p2, p3, p4));
}
public static Reloadable compose(Reloadable r1,
Reloadable r2,
Reloadable r3,
Reloadable r4,
Function5, U> f) {
Reloadable4 reloadable = new Reloadable4<>(
r1.currentValue(),
r2.currentValue(),
r3.currentValue(),
r4.currentValue(),
f);
r1.addWeakObserver(reloadable.observer1);
r2.addWeakObserver(reloadable.observer2);
r3.addWeakObserver(reloadable.observer3);
r4.addWeakObserver(reloadable.observer4);
return reloadable;
}
public static Reloadable compose(Reloadable r1,
Reloadable r2,
Reloadable r3,
Reloadable r4,
Reloadable r5,
Function5 f) {
return compose(r1, r2, r3, r4, r5, (p1, p2, p3, p4, p5, prev) -> f.apply(p1, p2, p3, p4, p5));
}
public static Reloadable compose(Reloadable r1,
Reloadable r2,
Reloadable r3,
Reloadable r4,
Reloadable r5,
Function6, U> f) {
Reloadable5 reloadable = new Reloadable5<>(
r1.currentValue(),
r2.currentValue(),
r3.currentValue(),
r4.currentValue(),
r5.currentValue(),
f);
r1.addWeakObserver(reloadable.observer1);
r2.addWeakObserver(reloadable.observer2);
r3.addWeakObserver(reloadable.observer3);
r4.addWeakObserver(reloadable.observer4);
r5.addWeakObserver(reloadable.observer5);
return reloadable;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy