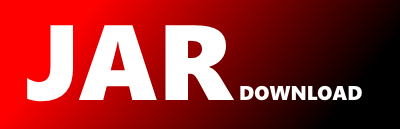
pro.foundev.cassandra.commons.migrations.Migration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cassandra-commons-migrations Show documentation
Show all versions of cassandra-commons-migrations Show documentation
Versioned Cassandra Schema Migrations For Sane Development
The newest version!
/*
* Copyright 2015 Ryan Svihla
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package pro.foundev.cassandra.commons.migrations;
import com.datastax.driver.core.Statement;
import com.datastax.driver.mapping.annotations.*;
import java.util.List;
/**
* The actual object responsible for migration
*/
@Table(name="migrations")
public class Migration {
@PartitionKey
@Column(name="migration_set")
private Integer migrationSet;
@ClusteringColumn
private Long version;
private String name;
private String cql;
@Transient
private List cqlToRun;
public String getCql() {
return cql;
}
public void setCql(String cql) {
this.cql = cql;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Long getVersion() {
return version;
}
public void setVersion(Long version) {
this.version = version;
}
public Integer getMigrationSet() {
return migrationSet;
}
public void setMigrationSet(Integer migrationSet) {
this.migrationSet = migrationSet;
}
public void setCqlToRun(List cqlToRun) {
StringBuffer buffer = new StringBuffer();
cqlToRun.forEach(s->buffer.append(s.toString()+"\n"));
this.setCql(buffer.toString());
this.cqlToRun = cqlToRun;
}
public List getCqlToRun() {
return cqlToRun;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy