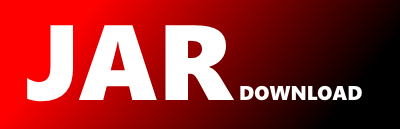
pro.foundev.cassandra.commons.migrations.script_parser.ScriptParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cassandra-commons-migrations Show documentation
Show all versions of cassandra-commons-migrations Show documentation
Versioned Cassandra Schema Migrations For Sane Development
The newest version!
/*
* Copyright 2015 Ryan Svihla
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package pro.foundev.cassandra.commons.migrations.script_parser;
import com.datastax.driver.core.SimpleStatement;
import com.datastax.driver.core.Statement;
import java.io.BufferedReader;
import java.io.IOException;
import java.nio.charset.Charset;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.ArrayList;
import java.util.List;
/**
* Generates a list of statements from a file
*/
public class ScriptParser {
private boolean isDoubleSlashComment(char newChar, char lastChar){
return newChar=='/' && lastChar=='/';
}
private boolean isDoubleDashComment(char newChar, char lastChar){
return newChar=='-' && lastChar=='-';
}
private boolean isMultiLineStart(char newChar, char lastChar){
return newChar=='*'&&lastChar=='/';
}
private boolean isMultilineEnd(char newChar, char lastChar){
return newChar=='/'&&lastChar=='*';
}
public List parse(Path file) {
List statements = new ArrayList<>();
StringBuffer cqlStatementBuffer = new StringBuffer();
//FIXME: detect charset
Charset charset = Charset.forName("UTF-8");
try (BufferedReader reader = Files.newBufferedReader(file, charset)) {
int c;
char lastChar = '\0';
boolean commentOn = false;
boolean multiLineCommentOn = false;
while ((c = reader.read()) != -1) {
char converted = (char)c;
if(isMultiLineStart(converted, lastChar)){
multiLineCommentOn = true;
cqlStatementBuffer = new StringBuffer();
}else if(isMultilineEnd(converted, lastChar)){
multiLineCommentOn = false;
}else {
if (isDoubleDashComment(converted, lastChar) || isDoubleSlashComment(converted, lastChar)) {
commentOn = true;
cqlStatementBuffer = new StringBuffer();//need to blank out previously stored -
} else if (commentOn && converted == '\n') {
commentOn = false;
}
lastChar = converted;
if (!commentOn && !multiLineCommentOn) {
cqlStatementBuffer.append(converted);
if (converted == ';') {
statements.add(new SimpleStatement(cqlStatementBuffer.toString()));
cqlStatementBuffer = new StringBuffer();
}
}
}
}
if(statements.size()==0&&cqlStatementBuffer.length()>0){
statements.add(new SimpleStatement(cqlStatementBuffer.toString()));
}
} catch (IOException x) {
throw new RuntimeException(x);
}
return statements;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy