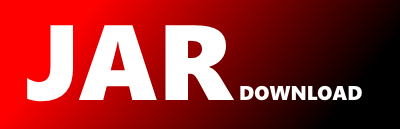
pro.taskana.common.rest.TaskanaEngineController Maven / Gradle / Ivy
package pro.taskana.common.rest;
import java.util.List;
import java.util.Map;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.hateoas.config.EnableHypermediaSupport;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import pro.taskana.TaskanaEngineConfiguration;
import pro.taskana.common.api.TaskanaEngine;
import pro.taskana.common.api.TaskanaRole;
import pro.taskana.common.internal.security.CurrentUserContext;
import pro.taskana.common.rest.models.TaskanaUserInfoRepresentationModel;
import pro.taskana.common.rest.models.VersionRepresentationModel;
/** Controller for TaskanaEngine related tasks. */
@RestController
@EnableHypermediaSupport(type = EnableHypermediaSupport.HypermediaType.HAL)
public class TaskanaEngineController {
private static final Logger LOGGER = LoggerFactory.getLogger(TaskanaEngineController.class);
private final TaskanaEngineConfiguration taskanaEngineConfiguration;
private final TaskanaEngine taskanaEngine;
@Value("${version:Local build}")
private String version;
TaskanaEngineController(
TaskanaEngineConfiguration taskanaEngineConfiguration, TaskanaEngine taskanaEngine) {
this.taskanaEngineConfiguration = taskanaEngineConfiguration;
this.taskanaEngine = taskanaEngine;
}
@GetMapping(path = Mapping.URL_DOMAIN)
public ResponseEntity> getDomains() {
ResponseEntity> response =
ResponseEntity.ok(taskanaEngineConfiguration.getDomains());
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("Exit from getDomains(), returning {}", response);
}
return response;
}
@GetMapping(path = Mapping.URL_CLASSIFICATION_CATEGORIES)
public ResponseEntity> getClassificationCategories(String type) {
LOGGER.debug("Entry to getClassificationCategories(type = {})", type);
ResponseEntity> response;
if (type != null) {
response =
ResponseEntity.ok(taskanaEngineConfiguration.getClassificationCategoriesByType(type));
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("Exit from getClassificationCategories(), returning {}", response);
}
return response;
}
response = ResponseEntity.ok(taskanaEngineConfiguration.getAllClassificationCategories());
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("Exit from getClassificationCategories(), returning {}", response);
}
return response;
}
@GetMapping(path = Mapping.URL_CLASSIFICATION_TYPES)
public ResponseEntity> getClassificationTypes() {
ResponseEntity> response =
ResponseEntity.ok(taskanaEngineConfiguration.getClassificationTypes());
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("Exit from getClassificationTypes(), returning {}", response);
}
return response;
}
@GetMapping(path = Mapping.URL_CLASSIFICATION_CATEGORIES_BY_TYPES)
public ResponseEntity
© 2015 - 2025 Weber Informatics LLC | Privacy Policy