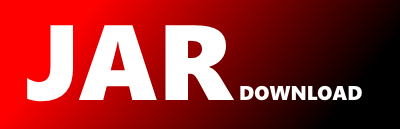
pro.taskana.task.rest.TaskCommentController Maven / Gradle / Ivy
package pro.taskana.task.rest;
import java.util.List;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.hateoas.config.EnableHypermediaSupport;
import org.springframework.hateoas.config.EnableHypermediaSupport.HypermediaType;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.web.bind.annotation.DeleteMapping;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.PutMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
import pro.taskana.common.api.exceptions.ConcurrencyException;
import pro.taskana.common.api.exceptions.InvalidArgumentException;
import pro.taskana.common.api.exceptions.NotAuthorizedException;
import pro.taskana.common.rest.Mapping;
import pro.taskana.common.rest.models.TaskanaPagedModel;
import pro.taskana.task.api.TaskService;
import pro.taskana.task.api.exceptions.TaskCommentNotFoundException;
import pro.taskana.task.api.exceptions.TaskNotFoundException;
import pro.taskana.task.api.models.TaskComment;
import pro.taskana.task.rest.assembler.TaskCommentRepresentationModelAssembler;
import pro.taskana.task.rest.models.TaskCommentRepresentationModel;
/** Controller for all {@link TaskComment} related endpoints. */
@RestController
@EnableHypermediaSupport(type = HypermediaType.HAL)
public class TaskCommentController {
private static final Logger LOGGER = LoggerFactory.getLogger(TaskCommentController.class);
private TaskService taskService;
private TaskCommentRepresentationModelAssembler taskCommentRepresentationModelAssembler;
TaskCommentController(
TaskService taskService,
TaskCommentRepresentationModelAssembler taskCommentRepresentationModelAssembler) {
this.taskService = taskService;
this.taskCommentRepresentationModelAssembler = taskCommentRepresentationModelAssembler;
}
@GetMapping(path = Mapping.URL_TASK_COMMENT)
@Transactional(readOnly = true, rollbackFor = Exception.class)
public ResponseEntity getTaskComment(
@PathVariable String taskCommentId)
throws NotAuthorizedException, TaskNotFoundException, TaskCommentNotFoundException,
InvalidArgumentException {
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("Entry to getTaskComment(taskCommentId= {})", taskCommentId);
}
TaskComment taskComment = taskService.getTaskComment(taskCommentId);
TaskCommentRepresentationModel taskCommentRepresentationModel =
taskCommentRepresentationModelAssembler.toModel(taskComment);
ResponseEntity response =
ResponseEntity.ok(taskCommentRepresentationModel);
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("Exit from getTaskComment(), returning {}", response);
}
return response;
}
@GetMapping(path = Mapping.URL_TASK_GET_POST_COMMENTS)
@Transactional(readOnly = true, rollbackFor = Exception.class)
public ResponseEntity> getTaskComments(
@PathVariable String taskId) throws NotAuthorizedException, TaskNotFoundException {
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("Entry to getTaskComments(taskId= {})", taskId);
}
List taskComments = taskService.getTaskComments(taskId);
TaskanaPagedModel taskCommentListResource =
taskCommentRepresentationModelAssembler.toPageModel(taskComments, null);
ResponseEntity> response =
ResponseEntity.ok(taskCommentListResource);
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("Exit from getTaskComments(), returning {}", response);
}
return response;
}
@DeleteMapping(path = Mapping.URL_TASK_COMMENT)
@Transactional(readOnly = true, rollbackFor = Exception.class)
public ResponseEntity deleteTaskComment(
@PathVariable String taskCommentId)
throws NotAuthorizedException, TaskNotFoundException, TaskCommentNotFoundException,
InvalidArgumentException {
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("Entry to deleteTaskComment(taskCommentId= {})", taskCommentId);
}
taskService.deleteTaskComment(taskCommentId);
ResponseEntity result = ResponseEntity.noContent().build();
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("Exit from deleteTaskComment(), returning {}", result);
}
return result;
}
@PutMapping(path = Mapping.URL_TASK_COMMENT)
@Transactional(readOnly = true, rollbackFor = Exception.class)
public ResponseEntity updateTaskComment(
@PathVariable String taskCommentId,
@RequestBody TaskCommentRepresentationModel taskCommentRepresentationModel)
throws NotAuthorizedException, TaskNotFoundException, TaskCommentNotFoundException,
InvalidArgumentException, ConcurrencyException {
if (LOGGER.isDebugEnabled()) {
LOGGER.debug(
"Entry to updateTaskComment(taskCommentId= {}, taskCommentResource= {})",
taskCommentId,
taskCommentRepresentationModel);
}
ResponseEntity result = null;
if ((taskCommentId.equals(taskCommentRepresentationModel.getTaskCommentId()))) {
TaskComment taskComment =
taskCommentRepresentationModelAssembler.toEntityModel(taskCommentRepresentationModel);
taskComment = taskService.updateTaskComment(taskComment);
result = ResponseEntity.ok(taskCommentRepresentationModelAssembler.toModel(taskComment));
} else {
throw new InvalidArgumentException(
String.format(
"TaskCommentId ('%s') is not identical with the id"
+ " of the object in the payload which should be updated",
taskCommentId));
}
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("Exit from updateTaskComment(), returning {}", result);
}
return result;
}
@PostMapping(path = Mapping.URL_TASK_GET_POST_COMMENTS)
@Transactional(rollbackFor = Exception.class)
public ResponseEntity createTaskComment(
@PathVariable String taskId,
@RequestBody TaskCommentRepresentationModel taskCommentRepresentationModel)
throws NotAuthorizedException, InvalidArgumentException, TaskNotFoundException {
if (LOGGER.isDebugEnabled()) {
LOGGER.debug(
"Entry to createTaskComment(taskId= {}, taskCommentResource= {})",
taskId,
taskCommentRepresentationModel);
}
taskCommentRepresentationModel.setTaskId(taskId);
TaskComment taskCommentFromResource =
taskCommentRepresentationModelAssembler.toEntityModel(taskCommentRepresentationModel);
TaskComment createdTaskComment = taskService.createTaskComment(taskCommentFromResource);
ResponseEntity result;
result =
ResponseEntity.status(HttpStatus.CREATED)
.body(taskCommentRepresentationModelAssembler.toModel(createdTaskComment));
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("Exit from createTaskComment(), returning {}", result);
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy