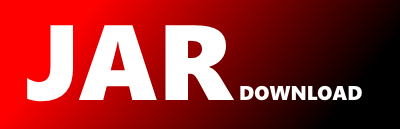
pro.zackpollard.telegrambot.api.menu.button.AbstractButtonBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jtelegram-botapi Show documentation
Show all versions of jtelegram-botapi Show documentation
The Java Telegram Bot API is a wrapper for the Telegram HTTP Bot API
package pro.zackpollard.telegrambot.api.menu.button;
import pro.zackpollard.telegrambot.api.menu.AbstractInlineMenuBuilder;
import pro.zackpollard.telegrambot.api.menu.InlineMenu;
import pro.zackpollard.telegrambot.api.menu.InlineMenuRowBuilder;
import pro.zackpollard.telegrambot.api.menu.button.callback.ButtonCallback;
/**
* Abstract methods used across all button creation
* @param implementing type
* @param menu builder type
* @author Mazen Kotb
*/
public abstract class AbstractButtonBuilder {
protected final InlineMenuRowBuilder parent;
protected final int index;
protected String text;
protected ButtonCallback callback;
protected AbstractButtonBuilder(InlineMenuRowBuilder parent, int index) {
this.parent = parent;
this.index = index;
}
protected AbstractButtonBuilder(InlineMenuRowBuilder parent, int index, String text) {
this.parent = parent;
this.index = index;
this.text = text;
}
protected abstract T instance();
/**
* Build the current builder
* @return parent row builder
*/
public abstract InlineMenuRowBuilder build();
/**
* Set the text of the current button. Required call unless provided at init
* @param text Text to be set
* @return this
*/
public T text(String text) {
this.text = text;
return instance();
}
/**
* Set the button callback of this builder.
* @param callback Callback to be set
* @return this
*/
public T buttonCallback(ButtonCallback callback) {
this.callback = callback;
return instance();
}
/**
* Build the current button, delegate to parent's newRow method
* @return new row builder
* @see InlineMenuRowBuilder#newRow()
*/
public InlineMenuRowBuilder newRow() {
build();
return parent.newRow();
}
/**
* Build the current button, delegate to parent's build method
* @return current menu builder
* @see InlineMenuRowBuilder#build()
*/
public E buildRow() {
build();
return parent.build();
}
/**
* Build the button, build the parent's row, build the menu
* @return built menu
* @see InlineMenuRowBuilder#build()
* @see AbstractInlineMenuBuilder#buildMenu()
*/
public InlineMenu buildMenu() {
build();
return parent.build().buildMenu();
}
protected InlineMenuButton processButton(InlineMenuButton button) {
return button.setCallback(callback);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy