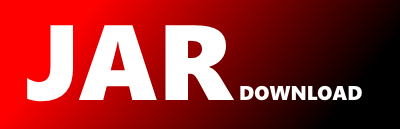
commonMain.LfLong.kt Maven / Gradle / Ivy
@file:JvmName("LfLong")
package pt.lightweightform.lfkotlin
import kotlin.jvm.JvmName
import kotlin.math.absoluteValue
/** Maximum value supported by [LfLong] (equals `Number.MAX_SAFE_INTEGER` in JS). */
public const val LF_LONG_MAX_VALUE: Long = 9007199254740991
/** Minimum value supported by [LfLong] (equals `Number.MIN_SAFE_INTEGER` in JS). */
public const val LF_LONG_MIN_VALUE: Long = -LF_LONG_MAX_VALUE
/**
* A long that can be used from common code which is represented by a JS number on the JS side
* (instead of Kotlin's own class) and as a [Long] on the JVM. Use `toLong()` to use this long from
* common code. This common long only accepts values in the range [LF_LONG_MIN_VALUE] to
* [LF_LONG_MAX_VALUE] in order to be compatible with JS numbers.
*/
public expect class LfLong : Comparable
/** [Long] as an LF long. */
public fun Long.toLfLong(): LfLong =
if (this.absoluteValue <= LF_LONG_MAX_VALUE) this.toLfLongImpl()
else
error(
"Cannot convert 'Long' to 'LfLong': absolute value is greater than '$LF_LONG_MAX_VALUE'"
)
/** LF long as a [Long]. */
public fun LfLong.toLong(): Long =
this.toLongImpl().also { long ->
if (long.absoluteValue > LF_LONG_MAX_VALUE) {
error(
"'LfLong' is in an invalid state: absolute value greater than '$LF_LONG_MAX_VALUE'"
)
}
}
// Implementations
internal expect fun Long.toLfLongImpl(): LfLong
internal expect fun LfLong.toLongImpl(): Long
// Other conversions:
public fun LfLong.toByte(): Byte = this.toLong().toByte()
public fun LfLong.toShort(): Short = this.toLong().toShort()
public fun LfLong.toInt(): Int = this.toLong().toInt()
public fun LfLong.toFloat(): Float = this.toLong().toFloat()
public fun LfLong.toDouble(): Double = this.toLong().toDouble()
public fun Number.toLfLong(): LfLong = this.toLong().toLfLong()
// Comparators:
public operator fun LfLong.compareTo(value: Byte): Int = this.toLong().compareTo(value)
public operator fun LfLong.compareTo(value: Short): Int = this.toLong().compareTo(value)
public operator fun LfLong.compareTo(value: Int): Int = this.toLong().compareTo(value)
public operator fun LfLong.compareTo(value: Long): Int = this.toLong().compareTo(value)
public operator fun LfLong.compareTo(value: Float): Int = this.toLong().compareTo(value)
public operator fun LfLong.compareTo(value: Double): Int = this.toLong().compareTo(value)
public operator fun Number.compareTo(value: LfLong): Int = this.toDouble().compareTo(value.toLong())
© 2015 - 2025 Weber Informatics LLC | Privacy Policy