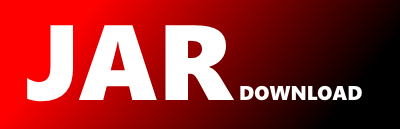
jsMain.Context.kt Maven / Gradle / Ivy
package pt.lightweightform.lfkotlin
import pt.lightweightform.lfkotlin.internal.jsToKtValue
/**
* `Storage` from `@lightweightform/storage` (parts relevant to `lf-kotlin`, i.e. parts that do not
* write).
*/
public external class Storage {
public val currentPath: Path
public val currentPathAsArray: Array
public val currentId: dynamic
public val validationMode: String
public fun pathInfo(relativePath: Path, options: dynamic): Array
public fun validatePath(relativePath: Path)
public fun resolvePath(relativePath: Path): Path
public fun resolvePathToArray(relativePath: Path): Array
public fun id(relativePath: Path): dynamic
public fun hasPath(relativePath: Path): Boolean
public fun relativeStorage(relativePath: Path): Storage
public fun schema(relativePath: Path): Schema<*>
public fun isComputed(relativePath: Path): Boolean
public fun isClientOnly(relativePath: Path): Boolean
public fun isNullable(relativePath: Path): Boolean
public fun isRemovable(relativePath: Path): Boolean
public fun has(relativePath: Path): Boolean
public fun get(relativePath: Path): dynamic
public fun getAsJS(relativePath: Path): dynamic
public fun getState(relativePath: Path): dynamic
public fun hasStateProperty(relativePath: Path): Boolean
public fun getStateProperty(relativePath: Path, prop: String): dynamic
public fun statePropertyStatus(relativePath: Path, prop: String): String
public fun typeIsValid(
relativePath: Path,
value: dynamic,
issuesMap: dynamic,
options: dynamic
): Boolean
public fun validationIssues(relativePath: Path): dynamic
public fun hasIssues(relativePath: Path): dynamic
public fun validationStatus(relativePath: Path): String
public fun localValidationIssues(relativePath: Path): dynamic
public fun childrenIds(relativePath: Path): Array
public fun size(relativePath: Path): Double
}
/** A context backed by the LF storage. */
public actual class Context(public val storage: Storage) {
public actual val currentPath: Path = storage.currentPath
public actual fun relativeContext(relativePath: Path): Context =
Context(storage.relativeStorage(relativePath))
@Suppress("UNCHECKED_CAST_TO_EXTERNAL_INTERFACE", "UNCHECKED_CAST")
public actual fun get(relativePath: Path): T {
val partInfo = storage.pathInfo(relativePath, js("{ fetchValues: true }")).last()
return jsToKtValue(partInfo.schema as Schema, partInfo.value) as T
}
@Suppress("UNCHECKED_CAST")
public actual fun getStateProperty(relativePath: Path, prop: String): T =
storage.getStateProperty(relativePath, prop) as T
}
/**
* A "storage validation": a validation in the format expected by the [Storage] (a function that
* receives a relative [Storage] instance as context and returns either an array of validation
* issues, or a promise to such an array).
*/
public typealias StorageContextFn = (ctx: Storage) -> Any?
© 2015 - 2025 Weber Informatics LLC | Privacy Policy