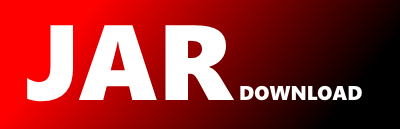
redis.clients.jedis.search.aggr.AggregationResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jedis Show documentation
Show all versions of jedis Show documentation
Jedis is a blazingly small and sane Redis java client.
package redis.clients.jedis.search.aggr;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import redis.clients.jedis.exceptions.JedisDataException;
import redis.clients.jedis.util.SafeEncoder;
public class AggregationResult {
/**
* @deprecated Use {@link AggregationResult#getTotalResults()}.
*/
@Deprecated
public final long totalResults;
private final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy