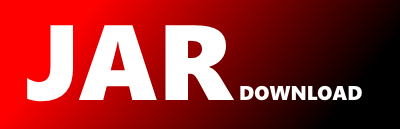
ren.crux.jadb.base.ActivityManagerBase Maven / Gradle / Ivy
/*
*
* Copyright 2018 The Crux Authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package ren.crux.jadb.base;
import lombok.NonNull;
import org.apache.commons.exec.CommandLine;
import ren.crux.jadb.Consts;
import ren.crux.jadb.model.Intent;
import ren.crux.jadb.model.Target;
import ren.crux.jadb.util.CommandLineTools;
import static ren.crux.jadb.Consts.Command.Shell.ActivityManager.*;
import static ren.crux.jadb.Consts.Option.ActivityManager.*;
/**
* @author wangzhihui
*/
public class ActivityManagerBase {
private final ShellBase base;
public ActivityManagerBase(ShellBase base) {
this.base = base;
}
private CommandLine create(Target target) {
return base.create(target)
.addArgument(Consts.Command.Shell.ACTIVITY_MANAGER);
}
/**
* Force stop everything associated with package (the app's package name).
*
* @param target
* @param packageName
* @return
* @throws Exception
*/
public String forceStop(Target target, @NonNull String packageName) throws Exception {
CommandLine command = create(target)
.addArgument(FORCE_STOP)
.addArgument(packageName);
return CommandLineTools.exec(command);
}
public String forceStop(@NonNull String packageName) throws Exception {
return forceStop(null, packageName);
}
/**
* Kill all processes associated with package (the app's package name).
*
* This command kills only processes that are safe to kill and that will not impact the user experience.
*
* @param target
* @param packageName
* @return
* @throws Exception
*/
public String kill(Target target, @NonNull String packageName) throws Exception {
CommandLine command = create(target)
.addArgument(KILL)
.addArgument(packageName);
return CommandLineTools.exec(command);
}
public String kill(@NonNull String packageName) throws Exception {
return kill(null, packageName);
}
/**
* Kill all background processes.
*
* @param target
* @return
* @throws Exception
*/
public String killAll(Target target) throws Exception {
CommandLine command = create(target)
.addArgument(KILL_ALL);
return CommandLineTools.exec(command);
}
public String killAll() throws Exception {
return killAll(null);
}
/**
* Start an Activity specified by intent.
*
* @param target
* @param wait
* @param repeat
* @param stop
* @param intent
* @return
* @throws Exception
*/
public String startActivity(Target target, boolean debug, boolean wait, int repeat, boolean stop, @NonNull Intent intent) throws Exception {
CommandLine command = create(target)
.addArgument(START);
if (debug) {
command.addArgument(DEBUG);
}
if (wait) {
command.addArgument(WAIT);
}
if (repeat > 0) {
command.addArgument(REPEAT).addArgument(String.valueOf(repeat));
}
if (stop) {
command.addArgument(STOP);
}
return CommandLineTools.exec(intent.write(command));
}
public String startActivity(boolean debug, boolean wait, int repeat, boolean stop, @NonNull Intent intent) throws Exception {
return startActivity(null, debug, wait, repeat, stop, intent);
}
/**
* Start the Service specified by intent.
*
* @param target
* @param intent
* @return
* @throws Exception
*/
public String startService(Target target, @NonNull Intent intent) throws Exception {
CommandLine command = create(target)
.addArgument(START_SERVICE);
return CommandLineTools.exec(intent.write(command));
}
public String startService(@NonNull Intent intent) throws Exception {
return startService(null, intent);
}
/**
* Issue a broadcast intent.
*
* @param target
* @param intent
* @return
* @throws Exception
*/
public String broadcast(Target target, @NonNull Intent intent) throws Exception {
CommandLine command = create(target)
.addArgument(BROADCAST);
return CommandLineTools.exec(intent.write(command));
}
public String broadcast(@NonNull Intent intent) throws Exception {
return broadcast(null, intent);
}
/**
* Print the given intent specification as a URI.
*
* @param intent
* @return
* @throws Exception
*/
public String toUri(@NonNull Intent intent) throws Exception {
CommandLine command = create(null)
.addArgument(TO_URI);
return CommandLineTools.exec(intent.write(command));
}
/**
* Print the given intent specification as an intent: URI.
*
* @param intent
* @return
* @throws Exception
*/
public String toIntentUri(@NonNull Intent intent) throws Exception {
CommandLine command = create(null)
.addArgument(TO_INTENT_URI);
return CommandLineTools.exec(intent.write(command));
}
}