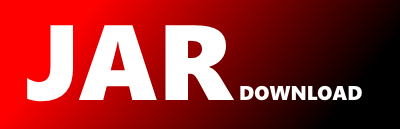
ren.crux.jadb.ActivityManager Maven / Gradle / Ivy
/*
*
* Copyright 2018 The Crux Authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package ren.crux.jadb;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import ren.crux.jadb.base.ActivityManagerBase;
import ren.crux.jadb.base.ShellBase;
import ren.crux.jadb.model.Intent;
import ren.crux.jadb.model.Target;
import ren.crux.jadb.util.OutputUtil;
import java.util.List;
import static ren.crux.jadb.Consts.Output.*;
/**
* @author wangzhihui
*/
@Slf4j
public class ActivityManager {
protected final ActivityManagerBase base;
public ActivityManager(ShellBase base) {
this.base = new ActivityManagerBase(base);
}
/**
* Force stop everything associated with package (the app's package name).
*
* @param target
* @param packageName
* @return
* @throws Exception
*/
public boolean forceStop(Target target, String packageName) throws Exception {
String output = base.forceStop(target, packageName);
return StringUtils.isBlank(output);
}
public boolean forceStop(String packageName) throws Exception {
return forceStop(null, packageName);
}
/**
* Kill all processes associated with package (the app's package name).
*
* This command kills only processes that are safe to kill and that will not impact the user experience.
*
* @param target
* @param packageName
* @return
* @throws Exception
*/
public boolean kill(Target target, String packageName) throws Exception {
String output = base.kill(target, packageName);
return StringUtils.isBlank(output);
}
public boolean kill(String packageName) throws Exception {
return kill(null, packageName);
}
/**
* Kill all background processes.
*
* @param target
* @return
* @throws Exception
*/
public boolean killAll(Target target) throws Exception {
String output = base.killAll(target);
return StringUtils.isBlank(output);
}
public boolean killAll() throws Exception {
return killAll(null);
}
/**
* Start an Activity specified by intent.
*
* @param target
* @param wait
* @param repeat
* @param stop
* @param intent
* @return
* @throws Exception
*/
public boolean startActivity(Target target, boolean debug, boolean wait, int repeat, boolean stop, Intent intent) throws Exception {
String output = base.startActivity(target, debug, wait, repeat, stop, intent);
List lines = OutputUtil.readLines(output);
if (!lines.isEmpty()) {
if (stop) {
if (!StringUtils.startsWith(lines.get(0), STOPPING_KEY_WORD)) {
return false;
}
lines.remove(0);
}
if (!lines.isEmpty()) {
if (!StringUtils.startsWith(lines.get(0), STARTING_KEY_WORD)) {
return false;
}
lines.remove(0);
if (wait) {
if (!lines.isEmpty() && !StringUtils.equals(lines.get(0), STATUS_OK_KEY_WORD)) {
return false;
}
}
if (repeat > 1) {
int startCount = 0;
int stopCount = 0;
for (String line : lines) {
if (StringUtils.startsWith(line, STARTING_KEY_WORD)) {
startCount++;
} else if (StringUtils.startsWith(line, STOPPING_KEY_WORD)) {
stopCount++;
}
}
return startCount == repeat - 1 && (!stop || stopCount == repeat - 1);
}
}
return true;
}
return false;
}
public boolean startActivity(boolean debug, boolean wait, int repeat, boolean stop, Intent intent) throws Exception {
return startActivity(null, debug, wait, repeat, stop, intent);
}
/**
* Start the Service specified by intent.
*
* @param target
* @param intent
* @return
* @throws Exception
*/
public boolean startService(Target target, Intent intent) throws Exception {
String output = base.startService(target, intent);
return StringUtils.startsWith(output, Consts.Output.STARTING_SERVICE_KEY_WORD);
}
public boolean startService(Intent intent) throws Exception {
return startService(null, intent);
}
/**
* Issue a broadcast intent.
*
* @param target
* @param intent
* @return
* @throws Exception
*/
public boolean broadcast(Target target, Intent intent) throws Exception {
String output = base.broadcast(target, intent);
return StringUtils.startsWith(output, Consts.Output.BROADCASTING_KEY_WORD);
}
public boolean broadcast(Intent intent) throws Exception {
return broadcast(null, intent);
}
/**
* Print the given intent specification as a URI.
*
* @param intent
* @return
* @throws Exception
*/
public String toUri(Intent intent) throws Exception {
return base.toUri(intent);
}
/**
* Print the given intent specification as an intent: URI.
*
* @param intent
* @return
* @throws Exception
*/
public String toIntentUri(Intent intent) throws Exception {
return base.toIntentUri(intent);
}
}