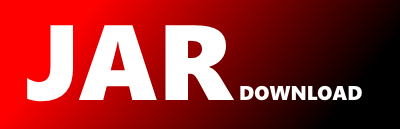
ren.crux.jadb.AndroidDebugBridge Maven / Gradle / Ivy
/*
*
* Copyright 2018 The Crux Authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package ren.crux.jadb;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import ren.crux.jadb.base.AndroidDebugBridgeBase;
import ren.crux.jadb.model.Device;
import ren.crux.jadb.model.DeviceState;
import ren.crux.jadb.model.Target;
import ren.crux.jadb.util.OutputUtil;
import java.util.ArrayList;
import java.util.List;
/**
* @author wangzhihui
*/
@Slf4j
public class AndroidDebugBridge {
protected AndroidDebugBridgeBase base;
public Shell shell;
public AndroidDebugBridge() {
this.base = new AndroidDebugBridgeBase();
shell = new Shell(base);
}
/**
* Print a list of all devices.
*
* @return
* @throws Exception
*/
public List devices() throws Exception {
List devices = new ArrayList<>();
String output = base.devices();
output = OutputUtil.requiredNonBlank(output);
int index = output.indexOf(Consts.Output.DEVICES_KEY_WORD);
if (index >= 0) {
String body = output.substring(index + Consts.Output.DEVICES_KEY_WORD.length());
List rows = OutputUtil.readLines(body);
for (String row : rows) {
log.debug("row : {}", row);
String[] cells = StringUtils.split(row, " ");
if (cells.length >= 2) {
Device device = new Device();
device.setSerialNumber(cells[0]);
device.setEmulator(StringUtils.startsWith(cells[0], "emulator-"));
device.setState(DeviceState.valueOf(cells[1]));
for (int i = 1; i < cells.length; i++) {
String cell = cells[i];
String[] pair = cell.split(":", 2);
if (pair.length == 2) {
switch (pair[0]) {
case "usb":
device.setUsb(pair[1]);
break;
case "product":
device.setProduct(pair[1]);
break;
case "model":
device.setModel(pair[1]);
break;
case "device":
device.setDevice(pair[1]);
break;
case "transport_id":
if (StringUtils.isNumeric(pair[1])) {
device.setTransportId(Integer.valueOf(pair[1]));
}
break;
default:
break;
}
}
}
devices.add(device);
}
}
} else {
throw new Exception("Invalid output : " + output);
}
return devices;
}
/**
* Connect to a device over TCP/IP.
*
* If you do not specify a port, then the default port, 5555, is used.
*
* @param serialNumber
* @return
* @throws Exception
*/
public boolean connect(String serialNumber) throws Exception {
String output = base.connect(serialNumber);
output = OutputUtil.requiredNonBlank(output);
return output.equals(Consts.Output.CONNECT_KEY_WORD + serialNumber) || output.equals(Consts.Output.CONNECT_ALREADY_KEY_WORD + serialNumber);
}
/**
* Disconnect from the specified TCP/IP device running on the specified port.
*
* If you do not specify a host or a port, then all devices are disconnected from all TCP/IP ports.
* If you specify a host, but not a port, the default port, 5555, is used.
*
* @param serialNumber
* @return
* @throws Exception
*/
public boolean disconnect(String serialNumber) throws Exception {
String output = base.disconnect(serialNumber);
output = OutputUtil.requiredNonBlank(output);
return output.equals(Consts.Output.DISCONNECT_KEY_WORD + serialNumber);
}
/**
* Installs a package (specified by path) to the system.
*
* @param target
* @param path
* @param grantAllPermissions
* @param replace
* @return
* @throws Exception
*/
public boolean install(Target target, String path, boolean grantAllPermissions, boolean replace) throws Exception {
String output = base.install(target, path, grantAllPermissions, replace);
output = OutputUtil.requiredNonBlank(output);
return output.equals(Consts.Output.SUCC_KEY_WORD);
}
public boolean install(String path, boolean grantAllPermissions, boolean replace) throws Exception {
return install(null, path, grantAllPermissions, replace);
}
/**
* Remove this app package from the device.
*
* @param target
* @param packageName
* @param keep
* @return
* @throws Exception
*/
public boolean uninstall(Target target, String packageName, boolean keep) throws Exception {
String output = base.uninstall(target, packageName, keep);
output = OutputUtil.requiredNonBlank(output);
return output.equals(Consts.Output.SUCC_KEY_WORD);
}
public boolean uninstall(String packageName, boolean keep) throws Exception {
return uninstall(null, packageName, keep);
}
/**
* Restart the adb server listening on TCP at the specified port.
*
* @param target
* @param port
* @return
* @throws Exception
*/
public boolean tcpip(Target target, int port) throws Exception {
String output = base.tcpip(target, port);
output = OutputUtil.requiredNonBlank(output);
return output.equals(Consts.Output.TCPIP_KEY_WORD + port);
}
public boolean tcpip(int port) throws Exception {
return tcpip(null, port);
}
}