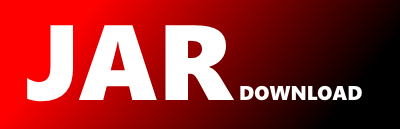
ro.fortsoft.genericdata.dao.GenericDAO Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of generic-data-dao Show documentation
Show all versions of generic-data-dao Show documentation
DAO module for generic-data
The newest version!
package ro.fortsoft.genericdata.dao;
import java.io.Serializable;
import java.util.List;
import ro.fortsoft.genericdata.utils.exception.AppException;
import ro.fortsoft.genericdata.utils.query.Pair;
import ro.fortsoft.genericdata.utils.query.QueryParameter;
public interface GenericDAO {
void saveOrUpdate(T obj) throws AppException;
T get(Class objClass, Serializable id) throws AppException;
T add(T obj) throws AppException;
List addList(List list) throws AppException;
void update(T obj) throws AppException;
void updateList(List list) throws AppException;
void delete(T obj) throws AppException;
void delete(Class clazz, Serializable id) throws AppException;
void deleteBulk(Class clazz) throws AppException;
void deleteBulk(Class clazz, QueryParameter qp) throws AppException;
void deleteBulk(Class clazz, T filter) throws AppException;
void deleteBulk(Class clazz, QueryParameter qp, T filter) throws AppException;
T getUnique(Class clazz) throws AppException;
T getUnique(Class clazz, QueryParameter qp) throws AppException;
T getUnique(Class clazz, T filter) throws AppException;
T getUnique(Class clazz, QueryParameter qp, T filter) throws AppException;
T getUniqueCached(Class clazz) throws AppException;
T getUniqueCached(Class clazz, QueryParameter qp) throws AppException;
T getUniqueCached(Class clazz, T filter) throws AppException;
T getUniqueCached(Class clazz, QueryParameter qp, T filter) throws AppException;
List getList(Class clazz) throws AppException;
Long getCount(Class clazz) throws AppException;
List getList(Class clazz, QueryParameter qp) throws AppException;
Long getCount(Class clazz, QueryParameter qp) throws AppException;
List getList(Class clazz, T filter) throws AppException;
Long getCount(Class clazz, T filter) throws AppException;
List getList(Class clazz, QueryParameter qp, T filter) throws AppException;
Long getCount(Class clazz, QueryParameter qp, T filter) throws AppException;
List getListCached(Class clazz) throws AppException;
Long getCountCached(Class clazz) throws AppException;
List getListCached(Class clazz, QueryParameter qp) throws AppException;
Long getCountCached(Class clazz, QueryParameter qp) throws AppException;
List getListCached(Class clazz, T filter) throws AppException;
Long getCountCached(Class clazz, T filter) throws AppException;
List getListCached(Class clazz, QueryParameter qp, T filter) throws AppException;
Long getCountCached(Class clazz, QueryParameter qp, T filter) throws AppException;
List> getLiteList(Class clazz, String idPropName, String displayPropName)
throws AppException;
List> getLiteList(Class clazz, String idPropName, String displayPropName,
QueryParameter qp) throws AppException;
List> getLiteList(Class clazz, String idPropName, String displayPropName,
QueryParameter qp, T filter) throws AppException;
List> getLiteListCached(Class clazz, String idPropName, String displayPropName)
throws AppException;
List> getLiteListCached(Class clazz, String idPropName, String displayPropName,
QueryParameter qp) throws AppException;
List> getLiteListCached(Class clazz, String idPropName, String displayPropName,
QueryParameter qp, T filter) throws AppException;
List getPropertyValues(Class clazz, QueryParameter qp, T filter, String propName, Class returnClass)
throws AppException;
List getPropertyValuesDistinct(Class clazz, QueryParameter qp, T filter, String propName,
Class returnClass) throws AppException;
V getPropertyValue(Class clazz, Serializable id, String propName, Class returnClass)
throws AppException;
List getPropertyValuesCached(Class clazz, QueryParameter qp, T filter, String propName,
Class returnClass) throws AppException;
List getPropertyValuesCachedDistinct(Class clazz, QueryParameter qp, T filter, String propName,
Class returnClass) throws AppException;
V getPropertyValueCached(Class clazz, Serializable id, String propName, Class returnClass)
throws AppException;
void flushSession();
void evict(T obj);
void evictWithRefresh(T obj);
void refresh(T obj);
void clearSession();
T merge(T obj);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy