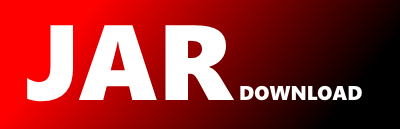
org.netbeans.modules.css.parser.css.jjt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of css-parser Show documentation
Show all versions of css-parser Show documentation
A CSS Parser extract from the NetBeans CSS modules
The newest version!
options {
TRACK_TOKENS = true;
IGNORE_CASE = true;
STATIC = false;
// UNICODE_INPUT = false;
USER_CHAR_STREAM = true;
}
PARSER_BEGIN(CssParser)
package org.netbeans.modules.css.parser;
import java.util.*;
public class CssParser {
private ArrayList errors = new ArrayList();
public CssParser() {
this((CharStream) null);
// change manager to our patched one:
token_source = new PatchedCssParserTokenManager(null);
}
public List errors() {
return errors;
}
}
PARSER_END(CssParser)
//TOKEN_MGR_DECLS :
TOKEN :
{
< S: ( " "|"\t"|"\r"|"\n"|"\f" )+ >
}
MORE :
{
< "/*" > : IN_COMMENT
}
MORE :
{
< ~[] >
}
TOKEN :
{
< COMMENT: "*/" > : DEFAULT
}
MORE:
{
< "expression(" > : MS_EXPRESSION
}
TOKEN :
{
//this is very simple identification of the end of the expression. The closing par. may be
//in a string or there might be some brackets in expressions inside.
< MSE : ")" > : DEFAULT
}
MORE :
{
< ~[] > : MS_EXPRESSION
}
TOKEN :
{
< LBRACE: "{" >
| < RBRACE: "}" >
| < COMMA: "," >
| < DOT: "." >
| < SEMICOLON: ";" >
| < COLON: ":" >
| < ASTERISK: "*" >
| < SLASH: "/" >
| < PLUS: "+" >
| < MINUS: "-" >
| < EQUALS: "=" >
| < GT: ">" >
| < LSQUARE: "[" >
| < RSQUARE: "]" >
}
TOKEN :
{
< HASH: "#" >
}
TOKEN :
{
< STRING: | >
| < RROUND: ")" >
}
TOKEN :
{
< #URL: ["!","#","$","%","&","*"-"~"] | | >
| < URI: "url(" ( )* ( | ( )* ) ( )* >
}
TOKEN : {
< GENERATED: "@@@" >
}
TOKEN :
{
< CDO: "" >
| < INCLUDES: "~=" >
| < CSS3_BEGINS: "^=" >
| < CSS3_ENDS: "$=" >
| < CSS3_CONTAINS: "*=" >
| < DASHMATCH: "|=" >
| < IMPORT_SYM: "@import" >
| < PAGE_SYM: "@page" >
| < MEDIA_SYM: "@media" >
| < FONT_FACE_SYM: "@font-face" >
| < CHARSET_SYM: "@charset" >
| < ATKEYWORD: "@" >
| < IMPORTANT_SYM: "!" ( )? "important" >
| < INHERIT: "inherit" >
| < EMS: "em" >
| < EXS: "ex" >
| < LENGTH_PX: "px" >
| < LENGTH_CM: "cm" >
| < LENGTH_MM: "mm" >
| < LENGTH_IN: "in" >
| < LENGTH_PT: "pt" >
| < LENGTH_PC: "pc" >
| < ANGLE_DEG: "deg" >
| < ANGLE_RAD: "rad" >
| < ANGLE_GRAD: "grad" >
| < TIME_MS: "ms" >
| < TIME_S: "s" >
| < FREQ_HZ: "Hz" >
| < FREQ_KHZ: "kHz" >
| < DIMEN: >
| < PERCENTAGE: "%" >
| < NUMBER: >
| < RGB: "rgb(" >
| < FUNCTION: "(" >
| < IDENT: ()+ | (( "-" )? ( )*) >
| < #NAME: ( )+ >
| < NUM: ( ["0"-"9"] )+ | ( ["0"-"9"] )* "." ( ["0"-"9"] )+ >
| < UNICODERANGE: "U+" ( | ( "-" ) ) >
| < #RANGE: | ( ()? | ( ()? | ( ()? | ( ()? | ( ()? | ) ) ) ) ) >
| < #Q16: "?" | "??" | "???" | "????" | "?????" | "??????" >
| < #Q15: "?" | "??" | "???" | "????" | "?????" >
| < #Q14: "?" | "??" | "???" | "????" >
| < #Q13: "?" | "??" | "???" >
| < #Q12: "?" | "??" >
| < #Q11: "?" >
| < #NMSTART: ["_"] | ["a"-"z"] | | >
| < #NMCHAR: ["_"] | ["a"-"z","0"-"9","-"] | | >
| < #STRING1: "\"" ( ["\t"," ","!","#","$","%","&","("-"~"] | "\\" | "\'" | | )* "\"" >
| < #STRING2: "\'" ( ["\t"," ","!","#","$","%","&","("-"~"] | "\\" | "\"" | | )* "\'" >
| < #NONASCII: ["\u0080"-"\uFFFF"] >
| < #ESCAPE: | ( "\\" [" "-"~","\u0080"-"\uFFFF"] ) >
| < #NL: "\n" | "\r\n" | "\r" | "\f" >
| < #UNICODE: "\\" ( " " | "\t" | "\r" | "\n" | "\f" )? >
| < #HNUM: | | | | | >
| < #H: ["0"-"9","a"-"f"] >
}
<*> TOKEN:
{
< UNKNOWN: ~[] >
{
//System.err.println("Illegal character : " + image.toString());
}
}
SimpleNode styleSheet() :{} {
styleSheetRuleList()
{ return jjtThis; }
}
void styleSheetRuleList() :{} {
( charsetRule() )?
( ( | ) | | )*
( importRule() ( ( | ) | | )* )*
( ( { return; } | rule() ) ( ( | ) | | )* )*
}
void rule() :{} {
styleRule() | mediaRule() | pageRule() | fontFaceRule() | unknownRule() | error_skip_to_whitespace()
}
//
// This is used by ASTStyleSheet.insertRule to parse a single rule
//
void styleSheetRuleSingle() :{} {
( charsetRule() | importRule() | styleRule() | mediaRule() | pageRule() | fontFaceRule() | unknownRule() )
}
void charsetRule() : {} {
( ( | ) )*
( ( | ) )*
}
void unknownRule() :{} {
skip()
}
void importRule() : {} {
( ( | ) )*
(
| ) ( ( | ) )*
( mediaList() )?
}
void mediaRule() : {} {
try {
( ( | ) )*
mediaList()
( ( | ) )*
( mediaRuleList() )?
} catch(ParseException e) {
reportError(e);
error_skipblock();
}
}
void mediaList() :{} {
medium()
( ( ( | ) )* medium() )*
}
void mediaRuleList() : {} {
( ( styleRule() | pageRule() | unknownRule() ) ( ( | ) )* )+
}
void mediaRuleSingle() :
{
}
{
( styleRule() | pageRule() | unknownRule() )
}
void medium() :
{
}
{
( | ) ( ( | ) )*
}
void pageRule() :
{
Token t = null;
String s = null;
boolean start = false;
}
{
( ( | ) )*
( LOOKAHEAD(2) ( t = ( ( | ) )* ) |
( pseudoPage() ( ( | ) )* ) |
( pseudoPage() ( ( | ) )* ) )?
( ( | ) )*
( declaration() )?
( ( ( | ) )* ( declaration() )? )*
}
//
// pseudoPage
// : ':' IDENT
// ;
//
void pseudoPage() :
{
}
{
}
//
// font_face
// : FONT_FACE_SYM S*
// '{' S* declaration [ ';' S* declaration ]* '}' S*
// ;
//
void fontFaceRule() :
{
}
{
( ( | ) )*
( ( | ) )*
( declaration() )?
( ( ( | ) )* ( declaration() )? )*
}
//
// operator
// : '/' S* | ',' S* |
// ;
//
void operator() :
{
}
{
( ( | ) )* | ( ( | ) )*
}
//
// combinator
// : '+' S* | '>' S* |
// ;
//
void combinator() :
{
}
{
( ( ( | ) )*
| ( ( | ) )*
| ( | ) ( ( | ) ( ( | ) )* )? )
}
//
// unary_operator
// : '-' | '+'
// ;
//
void unaryOperator() :
{
}
{
( | )
}
//
// property
// : IDENT S*
// ;
//
void property() :
{
}
{
( | | ) ( ( | ) )*
}
//
// ruleset
// : selector [ ',' S* selector ]*
// '{' S* declaration [ ';' S* declaration ]* '}' S*
// ;
//
//TODO marek: resolve the error reporting and recovery in styleRule()
void styleRule() :
{
}
{
try {
selectorList()
( ( | ) )*
( declaration() )?
( ( ( | ) )* ( declaration() )? )*
} catch(ParseException e) {
reportError(e);
error_skipblock();
}
}
void selectorList() :
{
}
{
try {
selector()
( ( ( | ) )* selector() )*
} catch (ParseException e) {
reportError(e);
}
}
//
// selector
// : simple_selector [ combinator simple_selector ]*
// ;
//
void selector() :
{
}
{
try {
simpleSelector()
( LOOKAHEAD(2) combinator() simpleSelector() )* ( ( | ) )*
} catch (ParseException e) {
reportError(e);
skipSelector();
}
}
//
// simple_selector
// : element_name? [ HASH | class | attrib | pseudo ]* S*
// ;
//
void simpleSelector() :
{
}
{
(
( elementName()
( hash()
| _class()
| attrib()
| pseudo()
)*
)
|
(
( hash()
| _class()
| attrib()
| pseudo()
)+
)
)
}
//
// class
// : '.' IDENT
// ;
//
void _class() :
{
}
{
( | )
}
//
// element_name
// : IDENT | '*'
// ;
//
void elementName() :
{
}
{
| |
}
//
// attrib
// : '[' S* IDENT S* [ [ '=' | INCLUDES | DASHMATCH ] S*
// [ IDENT | STRING ] S* ]? ']'
// ;
//
void attrib() :
{
}
{
( ( | ) )*
( ) ( ( | ) )*
(
(
|
|
|
|
|
)
( ( | ) )*
(
( )
|
)
( ( | ) )*
)?
}
//
// pseudo
// : ':' [ IDENT | FUNCTION S* IDENT S* ')' ]
// ;
//
void pseudo() :
{
}
{
(
( | )
|
(
( ( | ) )*
( (()? ) | ) ( ( | ) )*
)
)
}
void hash() :
{
}
{
}
void styleDeclaration() :
{
}
{