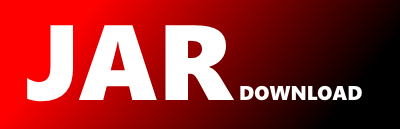
ru.curs.celesta.syscursors.CalllogCursor Maven / Gradle / Ivy
package ru.curs.celesta.syscursors;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Date;
import java.util.Iterator;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Objects;
import java.util.Set;
import java.util.function.Consumer;
import javax.annotation.Generated;
import ru.curs.celesta.CallContext;
import ru.curs.celesta.ICelesta;
import ru.curs.celesta.dbutils.BasicCursor;
import ru.curs.celesta.dbutils.CelestaGenerated;
import ru.curs.celesta.dbutils.Cursor;
import ru.curs.celesta.dbutils.CursorIterator;
import ru.curs.celesta.event.TriggerType;
import ru.curs.celesta.score.ColumnMeta;
import ru.curs.celesta.score.Table;
@Generated(
value = "ru.curs.celesta.plugin.maven.CursorGenerator",
date = "2023-02-12T00:37:44.201"
)
@CelestaGenerated
public class CalllogCursor extends Cursor implements Iterable {
private static final String GRAIN_NAME = "celesta";
private static final String OBJECT_NAME = "calllog";
public static final String TABLE_NAME = OBJECT_NAME;
public final CalllogCursor.Columns COLUMNS;
private Integer entryno;
private String sessionid;
private String userid;
private String procname;
private Date starttime;
private Integer duration;
{
this.COLUMNS = new CalllogCursor.Columns(callContext().getCelesta());
}
public CalllogCursor(CallContext context) {
super(context);
}
public CalllogCursor(CallContext context, ColumnMeta>... columns) {
super(context, columns);
}
@Deprecated
public CalllogCursor(CallContext context, Set fields) {
super(context, fields);
}
public Integer getEntryno() {
return this.entryno;
}
public CalllogCursor setEntryno(Integer entryno) {
this.entryno = entryno;
return this;
}
public String getSessionid() {
return this.sessionid;
}
public CalllogCursor setSessionid(String sessionid) {
this.sessionid = sessionid;
return this;
}
public String getUserid() {
return this.userid;
}
public CalllogCursor setUserid(String userid) {
this.userid = userid;
return this;
}
public String getProcname() {
return this.procname;
}
public CalllogCursor setProcname(String procname) {
this.procname = procname;
return this;
}
public Date getStarttime() {
return this.starttime;
}
public CalllogCursor setStarttime(Date starttime) {
this.starttime = starttime;
return this;
}
public Integer getDuration() {
return this.duration;
}
public CalllogCursor setDuration(Integer duration) {
this.duration = duration;
return this;
}
@Override
protected Object _getFieldValue(String name) {
switch (name) {
case "entryno": return this.entryno;
case "sessionid": return this.sessionid;
case "userid": return this.userid;
case "procname": return this.procname;
case "starttime": return this.starttime;
case "duration": return this.duration;
default: return null;
}
}
@Override
protected void _setFieldValue(String name, Object value) {
switch (name) {
case "entryno": {
this.entryno = (Integer) value;
break;
}
case "sessionid": {
this.sessionid = (String) value;
break;
}
case "userid": {
this.userid = (String) value;
break;
}
case "procname": {
this.procname = (String) value;
break;
}
case "starttime": {
this.starttime = (Date) value;
break;
}
case "duration": {
this.duration = (Integer) value;
break;
}
default:;
}
}
@Override
protected Object[] _currentKeyValues() {
return new Object[] {entryno};
}
public boolean tryGet(Integer entryno) {
return tryGetByValuesArray(entryno);
}
public void get(Integer entryno) {
getByValuesArray(entryno);
}
@Override
protected void _parseResultInternal(ResultSet rs) throws SQLException {
if (this.inRec("entryno")) {
this.entryno = rs.getInt("entryno");
if (rs.wasNull()) {
this.entryno = null;
}
}
if (this.inRec("sessionid")) {
this.sessionid = rs.getString("sessionid");
if (rs.wasNull()) {
this.sessionid = null;
}
}
if (this.inRec("userid")) {
this.userid = rs.getString("userid");
if (rs.wasNull()) {
this.userid = null;
}
}
if (this.inRec("procname")) {
this.procname = rs.getString("procname");
if (rs.wasNull()) {
this.procname = null;
}
}
if (this.inRec("starttime")) {
this.starttime = rs.getTimestamp("starttime");
if (rs.wasNull()) {
this.starttime = null;
}
}
if (this.inRec("duration")) {
this.duration = rs.getInt("duration");
if (rs.wasNull()) {
this.duration = null;
}
}
}
@Override
public void _clearBuffer(boolean withKeys) {
if (withKeys) {
this.entryno = null;
}
this.sessionid = null;
this.userid = null;
this.procname = null;
this.starttime = null;
this.duration = null;
}
@Override
public Object[] _currentValues() {
return new Object[] {entryno, sessionid, userid, procname, starttime, duration};
}
@Override
protected void _setAutoIncrement(int val) {
this.entryno = val;
}
public static void onPreDelete(ICelesta celesta,
Consumer super CalllogCursor> cursorConsumer) {
celesta.getTriggerDispatcher().registerTrigger(TriggerType.PRE_DELETE, CalllogCursor.class, cursorConsumer);
}
public static void onPostDelete(ICelesta celesta,
Consumer super CalllogCursor> cursorConsumer) {
celesta.getTriggerDispatcher().registerTrigger(TriggerType.POST_DELETE, CalllogCursor.class, cursorConsumer);
}
public static void onPreInsert(ICelesta celesta,
Consumer super CalllogCursor> cursorConsumer) {
celesta.getTriggerDispatcher().registerTrigger(TriggerType.PRE_INSERT, CalllogCursor.class, cursorConsumer);
}
public static void onPostInsert(ICelesta celesta,
Consumer super CalllogCursor> cursorConsumer) {
celesta.getTriggerDispatcher().registerTrigger(TriggerType.POST_INSERT, CalllogCursor.class, cursorConsumer);
}
public static void onPreUpdate(ICelesta celesta,
Consumer super CalllogCursor> cursorConsumer) {
celesta.getTriggerDispatcher().registerTrigger(TriggerType.PRE_UPDATE, CalllogCursor.class, cursorConsumer);
}
public static void onPostUpdate(ICelesta celesta,
Consumer super CalllogCursor> cursorConsumer) {
celesta.getTriggerDispatcher().registerTrigger(TriggerType.POST_UPDATE, CalllogCursor.class, cursorConsumer);
}
@Override
public CalllogCursor _getBufferCopy(CallContext context, List fields) {
final CalllogCursor result;
if (Objects.isNull(fields)) {
result = new CalllogCursor(context);
}
else {
result = new CalllogCursor(context, new LinkedHashSet<>(fields));
}
result.copyFieldsFrom(this);
return result;
}
@Override
public void copyFieldsFrom(BasicCursor c) {
CalllogCursor from = (CalllogCursor)c;
this.entryno = from.entryno;
this.sessionid = from.sessionid;
this.userid = from.userid;
this.procname = from.procname;
this.starttime = from.starttime;
this.duration = from.duration;
}
@Override
public Iterator iterator() {
return new CursorIterator(this);
}
@Override
protected String _grainName() {
return GRAIN_NAME;
}
@Override
protected String _objectName() {
return OBJECT_NAME;
}
@SuppressWarnings("unchecked")
@Generated(
value = "ru.curs.celesta.plugin.maven.CursorGenerator",
date = "2023-02-12T00:37:44.201"
)
@CelestaGenerated
public static final class Columns {
private final Table element;
public Columns(ICelesta celesta) {
this.element = celesta.getScore().getGrains().get(GRAIN_NAME).getElements(Table.class).get(OBJECT_NAME);
}
public ColumnMeta entryno() {
return (ColumnMeta) this.element.getColumns().get("entryno");
}
public ColumnMeta sessionid() {
return (ColumnMeta) this.element.getColumns().get("sessionid");
}
public ColumnMeta userid() {
return (ColumnMeta) this.element.getColumns().get("userid");
}
public ColumnMeta procname() {
return (ColumnMeta) this.element.getColumns().get("procname");
}
public ColumnMeta starttime() {
return (ColumnMeta) this.element.getColumns().get("starttime");
}
public ColumnMeta duration() {
return (ColumnMeta) this.element.getColumns().get("duration");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy