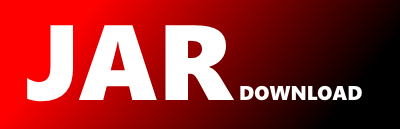
ru.curs.celesta.syscursors.GrainsCursor Maven / Gradle / Ivy
package ru.curs.celesta.syscursors;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Date;
import java.util.Iterator;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Objects;
import java.util.Set;
import java.util.function.Consumer;
import javax.annotation.Generated;
import ru.curs.celesta.CallContext;
import ru.curs.celesta.ICelesta;
import ru.curs.celesta.dbutils.BasicCursor;
import ru.curs.celesta.dbutils.CelestaGenerated;
import ru.curs.celesta.dbutils.Cursor;
import ru.curs.celesta.dbutils.CursorIterator;
import ru.curs.celesta.event.TriggerType;
import ru.curs.celesta.score.ColumnMeta;
import ru.curs.celesta.score.Table;
@Generated(
value = "ru.curs.celesta.plugin.maven.CursorGenerator",
date = "2023-02-12T00:37:44.169"
)
@CelestaGenerated
public class GrainsCursor extends Cursor implements Iterable, ISchemaCursor {
private static final String GRAIN_NAME = "celesta";
private static final String OBJECT_NAME = "grains";
public static final String TABLE_NAME = OBJECT_NAME;
public final GrainsCursor.Columns COLUMNS;
private String id;
private String version;
private Integer length;
private String checksum;
private Integer state;
private Date lastmodified;
private String message;
{
this.COLUMNS = new GrainsCursor.Columns(callContext().getCelesta());
}
public GrainsCursor(CallContext context) {
super(context);
}
public GrainsCursor(CallContext context, ColumnMeta>... columns) {
super(context, columns);
}
@Deprecated
public GrainsCursor(CallContext context, Set fields) {
super(context, fields);
}
public String getId() {
return this.id;
}
public GrainsCursor setId(String id) {
this.id = id;
return this;
}
public String getVersion() {
return this.version;
}
public GrainsCursor setVersion(String version) {
this.version = version;
return this;
}
public Integer getLength() {
return this.length;
}
public GrainsCursor setLength(Integer length) {
this.length = length;
return this;
}
public String getChecksum() {
return this.checksum;
}
public GrainsCursor setChecksum(String checksum) {
this.checksum = checksum;
return this;
}
public Integer getState() {
return this.state;
}
public GrainsCursor setState(Integer state) {
this.state = state;
return this;
}
public Date getLastmodified() {
return this.lastmodified;
}
public GrainsCursor setLastmodified(Date lastmodified) {
this.lastmodified = lastmodified;
return this;
}
public String getMessage() {
return this.message;
}
public GrainsCursor setMessage(String message) {
this.message = message;
return this;
}
@Override
protected Object _getFieldValue(String name) {
switch (name) {
case "id": return this.id;
case "version": return this.version;
case "length": return this.length;
case "checksum": return this.checksum;
case "state": return this.state;
case "lastmodified": return this.lastmodified;
case "message": return this.message;
default: return null;
}
}
@Override
protected void _setFieldValue(String name, Object value) {
switch (name) {
case "id": {
this.id = (String) value;
break;
}
case "version": {
this.version = (String) value;
break;
}
case "length": {
this.length = (Integer) value;
break;
}
case "checksum": {
this.checksum = (String) value;
break;
}
case "state": {
this.state = (Integer) value;
break;
}
case "lastmodified": {
this.lastmodified = (Date) value;
break;
}
case "message": {
this.message = (String) value;
break;
}
default:;
}
}
@Override
protected Object[] _currentKeyValues() {
return new Object[] {id};
}
public boolean tryGet(String id) {
return tryGetByValuesArray(id);
}
public void get(String id) {
getByValuesArray(id);
}
@Override
protected void _parseResultInternal(ResultSet rs) throws SQLException {
if (this.inRec("id")) {
this.id = rs.getString("id");
if (rs.wasNull()) {
this.id = null;
}
}
if (this.inRec("version")) {
this.version = rs.getString("version");
if (rs.wasNull()) {
this.version = null;
}
}
if (this.inRec("length")) {
this.length = rs.getInt("length");
if (rs.wasNull()) {
this.length = null;
}
}
if (this.inRec("checksum")) {
this.checksum = rs.getString("checksum");
if (rs.wasNull()) {
this.checksum = null;
}
}
if (this.inRec("state")) {
this.state = rs.getInt("state");
if (rs.wasNull()) {
this.state = null;
}
}
if (this.inRec("lastmodified")) {
this.lastmodified = rs.getTimestamp("lastmodified");
if (rs.wasNull()) {
this.lastmodified = null;
}
}
if (this.inRec("message")) {
this.message = rs.getString("message");
if (rs.wasNull()) {
this.message = null;
}
}
}
@Override
public void _clearBuffer(boolean withKeys) {
if (withKeys) {
this.id = null;
}
this.version = null;
this.length = null;
this.checksum = null;
this.state = null;
this.lastmodified = null;
this.message = null;
}
@Override
public Object[] _currentValues() {
return new Object[] {id, version, length, checksum, state, lastmodified, message};
}
@Override
protected void _setAutoIncrement(int val) {
}
public static void onPreDelete(ICelesta celesta,
Consumer super GrainsCursor> cursorConsumer) {
celesta.getTriggerDispatcher().registerTrigger(TriggerType.PRE_DELETE, GrainsCursor.class, cursorConsumer);
}
public static void onPostDelete(ICelesta celesta,
Consumer super GrainsCursor> cursorConsumer) {
celesta.getTriggerDispatcher().registerTrigger(TriggerType.POST_DELETE, GrainsCursor.class, cursorConsumer);
}
public static void onPreInsert(ICelesta celesta,
Consumer super GrainsCursor> cursorConsumer) {
celesta.getTriggerDispatcher().registerTrigger(TriggerType.PRE_INSERT, GrainsCursor.class, cursorConsumer);
}
public static void onPostInsert(ICelesta celesta,
Consumer super GrainsCursor> cursorConsumer) {
celesta.getTriggerDispatcher().registerTrigger(TriggerType.POST_INSERT, GrainsCursor.class, cursorConsumer);
}
public static void onPreUpdate(ICelesta celesta,
Consumer super GrainsCursor> cursorConsumer) {
celesta.getTriggerDispatcher().registerTrigger(TriggerType.PRE_UPDATE, GrainsCursor.class, cursorConsumer);
}
public static void onPostUpdate(ICelesta celesta,
Consumer super GrainsCursor> cursorConsumer) {
celesta.getTriggerDispatcher().registerTrigger(TriggerType.POST_UPDATE, GrainsCursor.class, cursorConsumer);
}
@Override
public GrainsCursor _getBufferCopy(CallContext context, List fields) {
final GrainsCursor result;
if (Objects.isNull(fields)) {
result = new GrainsCursor(context);
}
else {
result = new GrainsCursor(context, new LinkedHashSet<>(fields));
}
result.copyFieldsFrom(this);
return result;
}
@Override
public void copyFieldsFrom(BasicCursor c) {
GrainsCursor from = (GrainsCursor)c;
this.id = from.id;
this.version = from.version;
this.length = from.length;
this.checksum = from.checksum;
this.state = from.state;
this.lastmodified = from.lastmodified;
this.message = from.message;
}
@Override
public Iterator iterator() {
return new CursorIterator(this);
}
@Override
protected String _grainName() {
return GRAIN_NAME;
}
@Override
protected String _objectName() {
return OBJECT_NAME;
}
@SuppressWarnings("unchecked")
@Generated(
value = "ru.curs.celesta.plugin.maven.CursorGenerator",
date = "2023-02-12T00:37:44.169"
)
@CelestaGenerated
public static final class Columns {
private final Table element;
public Columns(ICelesta celesta) {
this.element = celesta.getScore().getGrains().get(GRAIN_NAME).getElements(Table.class).get(OBJECT_NAME);
}
public ColumnMeta id() {
return (ColumnMeta) this.element.getColumns().get("id");
}
public ColumnMeta version() {
return (ColumnMeta) this.element.getColumns().get("version");
}
public ColumnMeta length() {
return (ColumnMeta) this.element.getColumns().get("length");
}
public ColumnMeta checksum() {
return (ColumnMeta) this.element.getColumns().get("checksum");
}
public ColumnMeta state() {
return (ColumnMeta) this.element.getColumns().get("state");
}
public ColumnMeta lastmodified() {
return (ColumnMeta) this.element.getColumns().get("lastmodified");
}
public ColumnMeta message() {
return (ColumnMeta) this.element.getColumns().get("message");
}
}
@Generated(
value = "ru.curs.celesta.plugin.maven.CursorGenerator",
date = "2023-02-12T00:37:44.17"
)
@CelestaGenerated
public static final class State {
public static final Integer ready = 0;
public static final Integer upgrading = 1;
public static final Integer error = 2;
public static final Integer recover = 3;
public static final Integer lock = 4;
private State() {
throw new AssertionError();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy