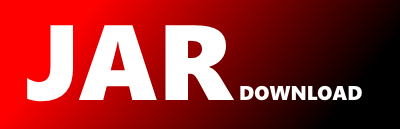
ru.curs.celesta.syscursors.LogCursor Maven / Gradle / Ivy
package ru.curs.celesta.syscursors;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Date;
import java.util.Iterator;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Objects;
import java.util.Set;
import java.util.function.Consumer;
import javax.annotation.Generated;
import ru.curs.celesta.CallContext;
import ru.curs.celesta.ICelesta;
import ru.curs.celesta.dbutils.BasicCursor;
import ru.curs.celesta.dbutils.CelestaGenerated;
import ru.curs.celesta.dbutils.Cursor;
import ru.curs.celesta.dbutils.CursorIterator;
import ru.curs.celesta.event.TriggerType;
import ru.curs.celesta.score.ColumnMeta;
import ru.curs.celesta.score.Table;
@Generated(
value = "ru.curs.celesta.plugin.maven.CursorGenerator",
date = "2023-02-12T00:37:44.189"
)
@CelestaGenerated
public class LogCursor extends Cursor implements Iterable {
private static final String GRAIN_NAME = "celesta";
private static final String OBJECT_NAME = "log";
public static final String TABLE_NAME = OBJECT_NAME;
public final LogCursor.Columns COLUMNS;
private Integer entryno;
private Date entryTime;
private String userid;
private String sessionid;
private String grainid;
private String tablename;
private String actionType;
private String pkvalue1;
private String pkvalue2;
private String pkvalue3;
private String oldvalues;
private String newvalues;
{
this.COLUMNS = new LogCursor.Columns(callContext().getCelesta());
}
public LogCursor(CallContext context) {
super(context);
}
public LogCursor(CallContext context, ColumnMeta>... columns) {
super(context, columns);
}
@Deprecated
public LogCursor(CallContext context, Set fields) {
super(context, fields);
}
public Integer getEntryno() {
return this.entryno;
}
public LogCursor setEntryno(Integer entryno) {
this.entryno = entryno;
return this;
}
public Date getEntryTime() {
return this.entryTime;
}
public LogCursor setEntryTime(Date entryTime) {
this.entryTime = entryTime;
return this;
}
public String getUserid() {
return this.userid;
}
public LogCursor setUserid(String userid) {
this.userid = userid;
return this;
}
public String getSessionid() {
return this.sessionid;
}
public LogCursor setSessionid(String sessionid) {
this.sessionid = sessionid;
return this;
}
public String getGrainid() {
return this.grainid;
}
public LogCursor setGrainid(String grainid) {
this.grainid = grainid;
return this;
}
public String getTablename() {
return this.tablename;
}
public LogCursor setTablename(String tablename) {
this.tablename = tablename;
return this;
}
public String getActionType() {
return this.actionType;
}
public LogCursor setActionType(String actionType) {
this.actionType = actionType;
return this;
}
public String getPkvalue1() {
return this.pkvalue1;
}
public LogCursor setPkvalue1(String pkvalue1) {
this.pkvalue1 = pkvalue1;
return this;
}
public String getPkvalue2() {
return this.pkvalue2;
}
public LogCursor setPkvalue2(String pkvalue2) {
this.pkvalue2 = pkvalue2;
return this;
}
public String getPkvalue3() {
return this.pkvalue3;
}
public LogCursor setPkvalue3(String pkvalue3) {
this.pkvalue3 = pkvalue3;
return this;
}
public String getOldvalues() {
return this.oldvalues;
}
public LogCursor setOldvalues(String oldvalues) {
this.oldvalues = oldvalues;
return this;
}
public String getNewvalues() {
return this.newvalues;
}
public LogCursor setNewvalues(String newvalues) {
this.newvalues = newvalues;
return this;
}
@Override
protected Object _getFieldValue(String name) {
switch (name) {
case "entryno": return this.entryno;
case "entry_time": return this.entryTime;
case "userid": return this.userid;
case "sessionid": return this.sessionid;
case "grainid": return this.grainid;
case "tablename": return this.tablename;
case "action_type": return this.actionType;
case "pkvalue1": return this.pkvalue1;
case "pkvalue2": return this.pkvalue2;
case "pkvalue3": return this.pkvalue3;
case "oldvalues": return this.oldvalues;
case "newvalues": return this.newvalues;
default: return null;
}
}
@Override
protected void _setFieldValue(String name, Object value) {
switch (name) {
case "entryno": {
this.entryno = (Integer) value;
break;
}
case "entry_time": {
this.entryTime = (Date) value;
break;
}
case "userid": {
this.userid = (String) value;
break;
}
case "sessionid": {
this.sessionid = (String) value;
break;
}
case "grainid": {
this.grainid = (String) value;
break;
}
case "tablename": {
this.tablename = (String) value;
break;
}
case "action_type": {
this.actionType = (String) value;
break;
}
case "pkvalue1": {
this.pkvalue1 = (String) value;
break;
}
case "pkvalue2": {
this.pkvalue2 = (String) value;
break;
}
case "pkvalue3": {
this.pkvalue3 = (String) value;
break;
}
case "oldvalues": {
this.oldvalues = (String) value;
break;
}
case "newvalues": {
this.newvalues = (String) value;
break;
}
default:;
}
}
@Override
protected Object[] _currentKeyValues() {
return new Object[] {entryno};
}
public boolean tryGet(Integer entryno) {
return tryGetByValuesArray(entryno);
}
public void get(Integer entryno) {
getByValuesArray(entryno);
}
@Override
protected void _parseResultInternal(ResultSet rs) throws SQLException {
if (this.inRec("entryno")) {
this.entryno = rs.getInt("entryno");
if (rs.wasNull()) {
this.entryno = null;
}
}
if (this.inRec("entry_time")) {
this.entryTime = rs.getTimestamp("entry_time");
if (rs.wasNull()) {
this.entryTime = null;
}
}
if (this.inRec("userid")) {
this.userid = rs.getString("userid");
if (rs.wasNull()) {
this.userid = null;
}
}
if (this.inRec("sessionid")) {
this.sessionid = rs.getString("sessionid");
if (rs.wasNull()) {
this.sessionid = null;
}
}
if (this.inRec("grainid")) {
this.grainid = rs.getString("grainid");
if (rs.wasNull()) {
this.grainid = null;
}
}
if (this.inRec("tablename")) {
this.tablename = rs.getString("tablename");
if (rs.wasNull()) {
this.tablename = null;
}
}
if (this.inRec("action_type")) {
this.actionType = rs.getString("action_type");
if (rs.wasNull()) {
this.actionType = null;
}
}
if (this.inRec("pkvalue1")) {
this.pkvalue1 = rs.getString("pkvalue1");
if (rs.wasNull()) {
this.pkvalue1 = null;
}
}
if (this.inRec("pkvalue2")) {
this.pkvalue2 = rs.getString("pkvalue2");
if (rs.wasNull()) {
this.pkvalue2 = null;
}
}
if (this.inRec("pkvalue3")) {
this.pkvalue3 = rs.getString("pkvalue3");
if (rs.wasNull()) {
this.pkvalue3 = null;
}
}
if (this.inRec("oldvalues")) {
this.oldvalues = rs.getString("oldvalues");
if (rs.wasNull()) {
this.oldvalues = null;
}
}
if (this.inRec("newvalues")) {
this.newvalues = rs.getString("newvalues");
if (rs.wasNull()) {
this.newvalues = null;
}
}
}
@Override
public void _clearBuffer(boolean withKeys) {
if (withKeys) {
this.entryno = null;
}
this.entryTime = null;
this.userid = null;
this.sessionid = null;
this.grainid = null;
this.tablename = null;
this.actionType = null;
this.pkvalue1 = null;
this.pkvalue2 = null;
this.pkvalue3 = null;
this.oldvalues = null;
this.newvalues = null;
}
@Override
public Object[] _currentValues() {
return new Object[] {entryno, entryTime, userid, sessionid, grainid, tablename, actionType, pkvalue1, pkvalue2, pkvalue3, oldvalues, newvalues};
}
@Override
protected void _setAutoIncrement(int val) {
this.entryno = val;
}
public static void onPreDelete(ICelesta celesta, Consumer super LogCursor> cursorConsumer) {
celesta.getTriggerDispatcher().registerTrigger(TriggerType.PRE_DELETE, LogCursor.class, cursorConsumer);
}
public static void onPostDelete(ICelesta celesta, Consumer super LogCursor> cursorConsumer) {
celesta.getTriggerDispatcher().registerTrigger(TriggerType.POST_DELETE, LogCursor.class, cursorConsumer);
}
public static void onPreInsert(ICelesta celesta, Consumer super LogCursor> cursorConsumer) {
celesta.getTriggerDispatcher().registerTrigger(TriggerType.PRE_INSERT, LogCursor.class, cursorConsumer);
}
public static void onPostInsert(ICelesta celesta, Consumer super LogCursor> cursorConsumer) {
celesta.getTriggerDispatcher().registerTrigger(TriggerType.POST_INSERT, LogCursor.class, cursorConsumer);
}
public static void onPreUpdate(ICelesta celesta, Consumer super LogCursor> cursorConsumer) {
celesta.getTriggerDispatcher().registerTrigger(TriggerType.PRE_UPDATE, LogCursor.class, cursorConsumer);
}
public static void onPostUpdate(ICelesta celesta, Consumer super LogCursor> cursorConsumer) {
celesta.getTriggerDispatcher().registerTrigger(TriggerType.POST_UPDATE, LogCursor.class, cursorConsumer);
}
@Override
public LogCursor _getBufferCopy(CallContext context, List fields) {
final LogCursor result;
if (Objects.isNull(fields)) {
result = new LogCursor(context);
}
else {
result = new LogCursor(context, new LinkedHashSet<>(fields));
}
result.copyFieldsFrom(this);
return result;
}
@Override
public void copyFieldsFrom(BasicCursor c) {
LogCursor from = (LogCursor)c;
this.entryno = from.entryno;
this.entryTime = from.entryTime;
this.userid = from.userid;
this.sessionid = from.sessionid;
this.grainid = from.grainid;
this.tablename = from.tablename;
this.actionType = from.actionType;
this.pkvalue1 = from.pkvalue1;
this.pkvalue2 = from.pkvalue2;
this.pkvalue3 = from.pkvalue3;
this.oldvalues = from.oldvalues;
this.newvalues = from.newvalues;
}
@Override
public Iterator iterator() {
return new CursorIterator(this);
}
@Override
protected String _grainName() {
return GRAIN_NAME;
}
@Override
protected String _objectName() {
return OBJECT_NAME;
}
@SuppressWarnings("unchecked")
@Generated(
value = "ru.curs.celesta.plugin.maven.CursorGenerator",
date = "2023-02-12T00:37:44.19"
)
@CelestaGenerated
public static final class Columns {
private final Table element;
public Columns(ICelesta celesta) {
this.element = celesta.getScore().getGrains().get(GRAIN_NAME).getElements(Table.class).get(OBJECT_NAME);
}
public ColumnMeta entryno() {
return (ColumnMeta) this.element.getColumns().get("entryno");
}
public ColumnMeta entryTime() {
return (ColumnMeta) this.element.getColumns().get("entry_time");
}
public ColumnMeta userid() {
return (ColumnMeta) this.element.getColumns().get("userid");
}
public ColumnMeta sessionid() {
return (ColumnMeta) this.element.getColumns().get("sessionid");
}
public ColumnMeta grainid() {
return (ColumnMeta) this.element.getColumns().get("grainid");
}
public ColumnMeta tablename() {
return (ColumnMeta) this.element.getColumns().get("tablename");
}
public ColumnMeta actionType() {
return (ColumnMeta) this.element.getColumns().get("action_type");
}
public ColumnMeta pkvalue1() {
return (ColumnMeta) this.element.getColumns().get("pkvalue1");
}
public ColumnMeta pkvalue2() {
return (ColumnMeta) this.element.getColumns().get("pkvalue2");
}
public ColumnMeta pkvalue3() {
return (ColumnMeta) this.element.getColumns().get("pkvalue3");
}
public ColumnMeta oldvalues() {
return (ColumnMeta) this.element.getColumns().get("oldvalues");
}
public ColumnMeta newvalues() {
return (ColumnMeta) this.element.getColumns().get("newvalues");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy