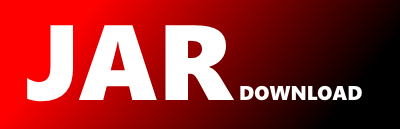
ru.cwcode.commands.executor.AbstractAutowiredExecutor Maven / Gradle / Ivy
package ru.cwcode.commands.executor;
import ru.cwcode.commands.Argument;
import ru.cwcode.commands.arguments.ExactStringArg;
import ru.cwcode.cwutils.collections.CollectionUtils;
import ru.cwcode.cwutils.messages.MessageReturn;
import ru.cwcode.cwutils.messages.TargetableMessageReturn;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
import static ru.cwcode.commands.api.CommandsAPI.l10n;
public abstract class AbstractAutowiredExecutor extends AbstractExecutor {
@Override
public void executeForPlayer() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy