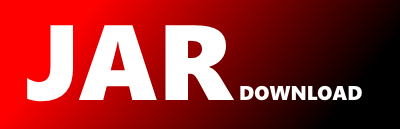
ru.cwcode.tkach.refreshmenu.inventory.shape.InventoryShape Maven / Gradle / Ivy
package ru.cwcode.tkach.refreshmenu.inventory.shape;
import org.bukkit.Material;
import org.bukkit.inventory.Inventory;
import ru.cwcode.tkach.locale.platform.MiniLocale;
import ru.cwcode.tkach.refreshmenu.inventory.ingredient.Ingredient;
import ru.cwcode.tkach.refreshmenu.inventory.type.InventoryTypeHolder;
import ru.cwcode.tkach.refreshmenu.inventory.view.View;
import java.util.HashMap;
import java.util.Optional;
public class InventoryShape {
InventoryTypeHolder type;
String name;
String[] shape;
HashMap ingredientMap = new HashMap<>();
transient String joinedShape = null;
public InventoryShape(String name, String[] shape, InventoryTypeHolder type, HashMap ingredients) {
this.name = name;
this.shape = shape;
this.type = type;
this.ingredientMap = ingredients;
}
public InventoryShape() {
}
public static ShapeBuilder builder() {
return new ShapeBuilder();
}
public static ShapeBuilder defaultPagedShape() {
//
return InventoryShape.builder()
.name("Меню")
.chest(54)
.shape("#########",
"#########",
"#########",
"#########",
"#########",
"#######<>")
.ingredient('<', Ingredient.builder()
.type(Material.ARROW)
.name("На страницу")
.description("/")
.build())
.ingredient('>', Ingredient.builder()
.type(Material.ARROW)
.name("На страницу")
.description("/")
.build());
//
}
public static ShapeBuilder defaultArtExtendedShape() {
//
return InventoryShape.builder()
.name("Меню")
.chest(54)
.shape("#########",
"#########",
"#########",
"#########",
"#########",
"#########",
"#########",
"#########",
"#########",
"#######<>")
.ingredient('<', Ingredient.builder()
.type(Material.ARROW)
.name("На страницу")
.description("/")
.build())
.ingredient('>', Ingredient.builder()
.type(Material.ARROW)
.name("На страницу")
.description("/")
.build());
//
}
public static ShapeBuilder defaultMultiShapeArtExtendedShape() {
//
return InventoryShape.builder()
.name("Меню")
.chest(54)
.shape("#########",
"#########",
"#########",
"#########",
"#########",
"#########",
"#########",
"#########",
"#########",
"#######{}")
.ingredient('{', Ingredient.builder()
.type(Material.SPECTRAL_ARROW)
.name("На страницу")
.description("/")
.build())
.ingredient('}', Ingredient.builder()
.type(Material.SPECTRAL_ARROW)
.name("На страницу")
.description("/")
.build());
//
}
public InventoryTypeHolder getType() {
return type;
}
public Inventory createInventory(View view) {
return type.createInventory(view, MiniLocale.getInstance().miniMessageWrapper().deserialize(getName(), view.getPlaceholders()));
}
/**
* @throws IndexOutOfBoundsException if the {@code index}
* argument is negative or not less than the length of this
* shape.
* Use the {@link #findCharAtIndex(int) findCharAtIndex} instead
*/
@Deprecated
public char charAtIndex(int index) {
return getJoinedShape().charAt(index);
}
public Optional findCharAtIndex(int index) {
if (index < 0 || index >= getJoinedShape().length()) {
return Optional.empty();
}
return Optional.of(getJoinedShape().charAt(index));
}
public int howMany(char toCount) {
int count = 0;
for (char c : getJoinedShape().toCharArray()) {
if (c == toCount) count++;
}
return count;
}
public void setType(InventoryTypeHolder type) {
this.type = type;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String[] getShape() {
return shape;
}
public void setShape(String... shape) {
this.shape = shape;
this.joinedShape = null;
}
public HashMap getIngredientMap() {
return ingredientMap;
}
public String getJoinedShape() {
if (joinedShape == null) joinedShape = String.join("", getShape());
return joinedShape;
}
@Override
public InventoryShape clone() {
return new InventoryShape(
this.getName(),
this.getShape().clone(),
this.getType(),
new HashMap<>(this.getIngredientMap())
);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy