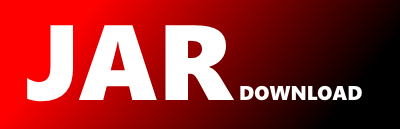
one.nio.server.acceptor.AcceptorSupport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of one-nio Show documentation
Show all versions of one-nio Show documentation
Unconventional Java I/O library
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy