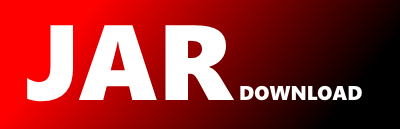
ru.saidgajiev.ormnext.cache.EvictHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ormnext-cache Show documentation
Show all versions of ormnext-cache Show documentation
Library which contains implementations Ormnext cache
The newest version!
package ru.saidgajiev.ormnext.cache;
import ru.saidgadjiev.ormnext.core.cache.CacheEvict;
import ru.saidgadjiev.ormnext.core.cache.ObjectCache;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Evict api implementation.
*
* @author Said Gadjiev
*/
public class EvictHelper implements CacheEvict {
/**
* Object cache map.
*/
private Map, ObjectCache> objectCacheMap = new HashMap<>();
/**
* Query for all results cache.
*/
private Map, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy