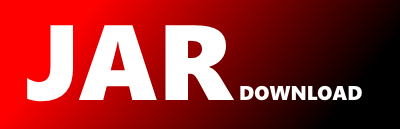
ru.testit.kotlin.client.apis.AttachmentsApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of testit-api-client-kotlin Show documentation
Show all versions of testit-api-client-kotlin Show documentation
Kotlin API client for TestIT.
/**
*
* Please note:
* This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit this file manually.
*
*/
@file:Suppress(
"ArrayInDataClass",
"EnumEntryName",
"RemoveRedundantQualifierName",
"UnusedImport"
)
package ru.testit.kotlin.client.apis
import java.io.IOException
import okhttp3.OkHttpClient
import okhttp3.HttpUrl
import ru.testit.kotlin.client.models.AttachmentModel
import ru.testit.kotlin.client.models.ImageResizeType
import ru.testit.kotlin.client.models.ProblemDetails
import ru.testit.kotlin.client.models.ValidationProblemDetails
import com.squareup.moshi.Json
import ru.testit.kotlin.client.infrastructure.ApiClient
import ru.testit.kotlin.client.infrastructure.ApiResponse
import ru.testit.kotlin.client.infrastructure.ClientException
import ru.testit.kotlin.client.infrastructure.ClientError
import ru.testit.kotlin.client.infrastructure.ServerException
import ru.testit.kotlin.client.infrastructure.ServerError
import ru.testit.kotlin.client.infrastructure.MultiValueMap
import ru.testit.kotlin.client.infrastructure.PartConfig
import ru.testit.kotlin.client.infrastructure.RequestConfig
import ru.testit.kotlin.client.infrastructure.RequestMethod
import ru.testit.kotlin.client.infrastructure.ResponseType
import ru.testit.kotlin.client.infrastructure.Success
import ru.testit.kotlin.client.infrastructure.toMultiValue
class AttachmentsApi(basePath: kotlin.String = defaultBasePath, client: OkHttpClient = ApiClient.defaultClient) : ApiClient(basePath, client) {
companion object {
@JvmStatic
val defaultBasePath: String by lazy {
System.getProperties().getProperty(ApiClient.baseUrlKey, "http://localhost")
}
}
/**
* Delete attachment file
*
* @param id
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2AttachmentsIdDelete(id: java.util.UUID) : Unit {
val localVarResponse = apiV2AttachmentsIdDeleteWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Delete attachment file
*
* @param id
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2AttachmentsIdDeleteWithHttpInfo(id: java.util.UUID) : ApiResponse {
val localVariableConfig = apiV2AttachmentsIdDeleteRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2AttachmentsIdDelete
*
* @param id
* @return RequestConfig
*/
fun apiV2AttachmentsIdDeleteRequestConfig(id: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.DELETE,
path = "/api/v2/attachments/{id}".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Download attachment file
*
* @param id
* @param width Width of the result image (optional)
* @param height Height of the result image (optional)
* @param resizeType Type of resizing to apply to the result image (optional)
* @param backgroundColor Color of the background if the `resizeType` is `AddBackgroundStripes` (optional)
* @param preview If image must be converted to a preview (lower quality, no animation) (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2AttachmentsIdGet(id: java.util.UUID, width: kotlin.Int? = null, height: kotlin.Int? = null, resizeType: ImageResizeType? = null, backgroundColor: kotlin.String? = null, preview: kotlin.Boolean? = null) : Unit {
val localVarResponse = apiV2AttachmentsIdGetWithHttpInfo(id = id, width = width, height = height, resizeType = resizeType, backgroundColor = backgroundColor, preview = preview)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Download attachment file
*
* @param id
* @param width Width of the result image (optional)
* @param height Height of the result image (optional)
* @param resizeType Type of resizing to apply to the result image (optional)
* @param backgroundColor Color of the background if the `resizeType` is `AddBackgroundStripes` (optional)
* @param preview If image must be converted to a preview (lower quality, no animation) (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2AttachmentsIdGetWithHttpInfo(id: java.util.UUID, width: kotlin.Int?, height: kotlin.Int?, resizeType: ImageResizeType?, backgroundColor: kotlin.String?, preview: kotlin.Boolean?) : ApiResponse {
val localVariableConfig = apiV2AttachmentsIdGetRequestConfig(id = id, width = width, height = height, resizeType = resizeType, backgroundColor = backgroundColor, preview = preview)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2AttachmentsIdGet
*
* @param id
* @param width Width of the result image (optional)
* @param height Height of the result image (optional)
* @param resizeType Type of resizing to apply to the result image (optional)
* @param backgroundColor Color of the background if the `resizeType` is `AddBackgroundStripes` (optional)
* @param preview If image must be converted to a preview (lower quality, no animation) (optional)
* @return RequestConfig
*/
fun apiV2AttachmentsIdGetRequestConfig(id: java.util.UUID, width: kotlin.Int?, height: kotlin.Int?, resizeType: ImageResizeType?, backgroundColor: kotlin.String?, preview: kotlin.Boolean?) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (width != null) {
put("width", listOf(width.toString()))
}
if (height != null) {
put("height", listOf(height.toString()))
}
if (resizeType != null) {
put("resizeType", listOf(resizeType.toString()))
}
if (backgroundColor != null) {
put("backgroundColor", listOf(backgroundColor.toString()))
}
if (preview != null) {
put("preview", listOf(preview.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/attachments/{id}".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get size of attachments storage in bytes
*
* @return kotlin.Long
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2AttachmentsOccupiedFileStorageSizeGet() : kotlin.Long {
val localVarResponse = apiV2AttachmentsOccupiedFileStorageSizeGetWithHttpInfo()
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.Long
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get size of attachments storage in bytes
*
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2AttachmentsOccupiedFileStorageSizeGetWithHttpInfo() : ApiResponse {
val localVariableConfig = apiV2AttachmentsOccupiedFileStorageSizeGetRequestConfig()
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2AttachmentsOccupiedFileStorageSizeGet
*
* @return RequestConfig
*/
fun apiV2AttachmentsOccupiedFileStorageSizeGetRequestConfig() : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/attachments/occupiedFileStorageSize",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Upload new attachment file
* File size is restricted to 50 MB (52 428 800 bytes)
* @param file (optional)
* @return AttachmentModel
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2AttachmentsPost(file: java.io.File? = null) : AttachmentModel {
val localVarResponse = apiV2AttachmentsPostWithHttpInfo(file = file)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as AttachmentModel
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Upload new attachment file
* File size is restricted to 50 MB (52 428 800 bytes)
* @param file (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2AttachmentsPostWithHttpInfo(file: java.io.File?) : ApiResponse {
val localVariableConfig = apiV2AttachmentsPostRequestConfig(file = file)
return request
© 2015 - 2024 Weber Informatics LLC | Privacy Policy