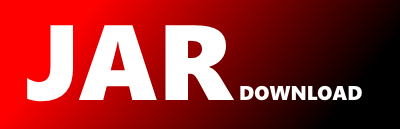
ru.testit.kotlin.client.apis.AutoTestsApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of testit-api-client-kotlin Show documentation
Show all versions of testit-api-client-kotlin Show documentation
Kotlin API client for TestIT.
/**
*
* Please note:
* This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit this file manually.
*
*/
@file:Suppress(
"ArrayInDataClass",
"EnumEntryName",
"RemoveRedundantQualifierName",
"UnusedImport"
)
package ru.testit.kotlin.client.apis
import java.io.IOException
import okhttp3.OkHttpClient
import okhttp3.HttpUrl
import ru.testit.kotlin.client.models.AutoTestAverageDurationModel
import ru.testit.kotlin.client.models.AutoTestModel
import ru.testit.kotlin.client.models.AutoTestPostModel
import ru.testit.kotlin.client.models.AutoTestPutModel
import ru.testit.kotlin.client.models.AutotestHistoricalResultSelectModel
import ru.testit.kotlin.client.models.AutotestResultHistoricalGetModel
import ru.testit.kotlin.client.models.AutotestsSelectModel
import ru.testit.kotlin.client.models.FlakyBulkModel
import ru.testit.kotlin.client.models.Operation
import ru.testit.kotlin.client.models.ProblemDetails
import ru.testit.kotlin.client.models.TestResultChronologyModel
import ru.testit.kotlin.client.models.TestResultHistoryReportModel
import ru.testit.kotlin.client.models.TestRunShortModel
import ru.testit.kotlin.client.models.ValidationProblemDetails
import ru.testit.kotlin.client.models.WorkItemIdModel
import ru.testit.kotlin.client.models.WorkItemIdentifierModel
import com.squareup.moshi.Json
import ru.testit.kotlin.client.infrastructure.ApiClient
import ru.testit.kotlin.client.infrastructure.ApiResponse
import ru.testit.kotlin.client.infrastructure.ClientException
import ru.testit.kotlin.client.infrastructure.ClientError
import ru.testit.kotlin.client.infrastructure.ServerException
import ru.testit.kotlin.client.infrastructure.ServerError
import ru.testit.kotlin.client.infrastructure.MultiValueMap
import ru.testit.kotlin.client.infrastructure.PartConfig
import ru.testit.kotlin.client.infrastructure.RequestConfig
import ru.testit.kotlin.client.infrastructure.RequestMethod
import ru.testit.kotlin.client.infrastructure.ResponseType
import ru.testit.kotlin.client.infrastructure.Success
import ru.testit.kotlin.client.infrastructure.toMultiValue
class AutoTestsApi(basePath: kotlin.String = defaultBasePath, client: OkHttpClient = ApiClient.defaultClient) : ApiClient(basePath, client) {
companion object {
@JvmStatic
val defaultBasePath: String by lazy {
System.getProperties().getProperty(ApiClient.baseUrlKey, "http://localhost")
}
}
/**
* Set \"Flaky\" status for multiple autotests
* User permissions for project: - Read only - Execute - Write - Full control
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param flakyBulkModel (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2AutoTestsFlakyBulkPost(skip: kotlin.Int? = null, take: kotlin.Int? = null, orderBy: kotlin.String? = null, searchField: kotlin.String? = null, searchValue: kotlin.String? = null, flakyBulkModel: FlakyBulkModel? = null) : Unit {
val localVarResponse = apiV2AutoTestsFlakyBulkPostWithHttpInfo(skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue, flakyBulkModel = flakyBulkModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Set \"Flaky\" status for multiple autotests
* User permissions for project: - Read only - Execute - Write - Full control
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param flakyBulkModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2AutoTestsFlakyBulkPostWithHttpInfo(skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?, flakyBulkModel: FlakyBulkModel?) : ApiResponse {
val localVariableConfig = apiV2AutoTestsFlakyBulkPostRequestConfig(skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue, flakyBulkModel = flakyBulkModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2AutoTestsFlakyBulkPost
*
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param flakyBulkModel (optional)
* @return RequestConfig
*/
fun apiV2AutoTestsFlakyBulkPostRequestConfig(skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?, flakyBulkModel: FlakyBulkModel?) : RequestConfig {
val localVariableBody = flakyBulkModel
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (skip != null) {
put("Skip", listOf(skip.toString()))
}
if (take != null) {
put("Take", listOf(take.toString()))
}
if (orderBy != null) {
put("OrderBy", listOf(orderBy.toString()))
}
if (searchField != null) {
put("SearchField", listOf(searchField.toString()))
}
if (searchValue != null) {
put("SearchValue", listOf(searchValue.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/autoTests/flaky/bulk",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Patch auto test
* See <a href=\"https://www.rfc-editor.org/rfc/rfc6902\" target=\"_blank\">RFC 6902: JavaScript Object Notation (JSON) Patch</a> for details
* @param id Global Id of auto test
* @param operation (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2AutoTestsIdPatch(id: java.util.UUID, operation: kotlin.collections.List? = null) : Unit {
val localVarResponse = apiV2AutoTestsIdPatchWithHttpInfo(id = id, operation = operation)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Patch auto test
* See <a href=\"https://www.rfc-editor.org/rfc/rfc6902\" target=\"_blank\">RFC 6902: JavaScript Object Notation (JSON) Patch</a> for details
* @param id Global Id of auto test
* @param operation (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2AutoTestsIdPatchWithHttpInfo(id: java.util.UUID, operation: kotlin.collections.List?) : ApiResponse {
val localVariableConfig = apiV2AutoTestsIdPatchRequestConfig(id = id, operation = operation)
return request, Unit>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2AutoTestsIdPatch
*
* @param id Global Id of auto test
* @param operation (optional)
* @return RequestConfig
*/
fun apiV2AutoTestsIdPatchRequestConfig(id: java.util.UUID, operation: kotlin.collections.List?) : RequestConfig> {
val localVariableBody = operation
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.PATCH,
path = "/api/v2/autoTests/{id}".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get test results history for autotest
* Use case User sets autotest internal (guid format) or global (integer format) identifier User sets getTestResultHistoryReportQuery (listed in the example) User runs method execution System search for test results using filters set by user in getTestResultHistoryReportQuery and id System returns the enumeration of test results
* @param id Autotest identifier
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param autotestHistoricalResultSelectModel (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2AutoTestsIdTestResultsSearchPost(id: kotlin.String, skip: kotlin.Int? = null, take: kotlin.Int? = null, orderBy: kotlin.String? = null, searchField: kotlin.String? = null, searchValue: kotlin.String? = null, autotestHistoricalResultSelectModel: AutotestHistoricalResultSelectModel? = null) : kotlin.collections.List {
val localVarResponse = apiV2AutoTestsIdTestResultsSearchPostWithHttpInfo(id = id, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue, autotestHistoricalResultSelectModel = autotestHistoricalResultSelectModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get test results history for autotest
* Use case User sets autotest internal (guid format) or global (integer format) identifier User sets getTestResultHistoryReportQuery (listed in the example) User runs method execution System search for test results using filters set by user in getTestResultHistoryReportQuery and id System returns the enumeration of test results
* @param id Autotest identifier
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param autotestHistoricalResultSelectModel (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2AutoTestsIdTestResultsSearchPostWithHttpInfo(id: kotlin.String, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?, autotestHistoricalResultSelectModel: AutotestHistoricalResultSelectModel?) : ApiResponse?> {
val localVariableConfig = apiV2AutoTestsIdTestResultsSearchPostRequestConfig(id = id, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue, autotestHistoricalResultSelectModel = autotestHistoricalResultSelectModel)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2AutoTestsIdTestResultsSearchPost
*
* @param id Autotest identifier
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param autotestHistoricalResultSelectModel (optional)
* @return RequestConfig
*/
fun apiV2AutoTestsIdTestResultsSearchPostRequestConfig(id: kotlin.String, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?, autotestHistoricalResultSelectModel: AutotestHistoricalResultSelectModel?) : RequestConfig {
val localVariableBody = autotestHistoricalResultSelectModel
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (skip != null) {
put("Skip", listOf(skip.toString()))
}
if (take != null) {
put("Take", listOf(take.toString()))
}
if (orderBy != null) {
put("OrderBy", listOf(orderBy.toString()))
}
if (searchField != null) {
put("SearchField", listOf(searchField.toString()))
}
if (searchValue != null) {
put("SearchValue", listOf(searchValue.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/autoTests/{id}/testResults/search".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get identifiers of changed linked work items
* User permissions for project: - Read only - Execute - Write - Full control
* @param id
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2AutoTestsIdWorkItemsChangedIdGet(id: java.util.UUID) : kotlin.collections.List {
val localVarResponse = apiV2AutoTestsIdWorkItemsChangedIdGetWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get identifiers of changed linked work items
* User permissions for project: - Read only - Execute - Write - Full control
* @param id
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2AutoTestsIdWorkItemsChangedIdGetWithHttpInfo(id: java.util.UUID) : ApiResponse?> {
val localVariableConfig = apiV2AutoTestsIdWorkItemsChangedIdGetRequestConfig(id = id)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2AutoTestsIdWorkItemsChangedIdGet
*
* @param id
* @return RequestConfig
*/
fun apiV2AutoTestsIdWorkItemsChangedIdGetRequestConfig(id: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/autoTests/{id}/workItems/changed/id".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Approve changes to work items linked to autotest
* User permissions for project: - Read only - Execute - Write - Full control
* @param id
* @param workItemId
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2AutoTestsIdWorkItemsChangedWorkItemIdApprovePost(id: java.util.UUID, workItemId: java.util.UUID) : Unit {
val localVarResponse = apiV2AutoTestsIdWorkItemsChangedWorkItemIdApprovePostWithHttpInfo(id = id, workItemId = workItemId)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Approve changes to work items linked to autotest
* User permissions for project: - Read only - Execute - Write - Full control
* @param id
* @param workItemId
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2AutoTestsIdWorkItemsChangedWorkItemIdApprovePostWithHttpInfo(id: java.util.UUID, workItemId: java.util.UUID) : ApiResponse {
val localVariableConfig = apiV2AutoTestsIdWorkItemsChangedWorkItemIdApprovePostRequestConfig(id = id, workItemId = workItemId)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2AutoTestsIdWorkItemsChangedWorkItemIdApprovePost
*
* @param id
* @param workItemId
* @return RequestConfig
*/
fun apiV2AutoTestsIdWorkItemsChangedWorkItemIdApprovePostRequestConfig(id: java.util.UUID, workItemId: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/autoTests/{id}/workItems/changed/{workItemId}/approve".replace("{"+"id"+"}", encodeURIComponent(id.toString())).replace("{"+"workItemId"+"}", encodeURIComponent(workItemId.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Search for autotests
*
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param autotestsSelectModel (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2AutoTestsSearchPost(skip: kotlin.Int? = null, take: kotlin.Int? = null, orderBy: kotlin.String? = null, searchField: kotlin.String? = null, searchValue: kotlin.String? = null, autotestsSelectModel: AutotestsSelectModel? = null) : kotlin.collections.List {
val localVarResponse = apiV2AutoTestsSearchPostWithHttpInfo(skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue, autotestsSelectModel = autotestsSelectModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Search for autotests
*
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param autotestsSelectModel (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2AutoTestsSearchPostWithHttpInfo(skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?, autotestsSelectModel: AutotestsSelectModel?) : ApiResponse?> {
val localVariableConfig = apiV2AutoTestsSearchPostRequestConfig(skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue, autotestsSelectModel = autotestsSelectModel)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2AutoTestsSearchPost
*
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param autotestsSelectModel (optional)
* @return RequestConfig
*/
fun apiV2AutoTestsSearchPostRequestConfig(skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?, autotestsSelectModel: AutotestsSelectModel?) : RequestConfig {
val localVariableBody = autotestsSelectModel
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (skip != null) {
put("Skip", listOf(skip.toString()))
}
if (take != null) {
put("Take", listOf(take.toString()))
}
if (orderBy != null) {
put("OrderBy", listOf(orderBy.toString()))
}
if (searchField != null) {
put("SearchField", listOf(searchField.toString()))
}
if (searchValue != null) {
put("SearchValue", listOf(searchValue.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/autoTests/search",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Create autotest
* This method creates a new autotest. To add an autotest to the test plan, link it to a work item using the `POST /api/v2/autoTests/{autoTestId}/workItems` method. Use the `POST /api/v2/testRuns/byAutoTests` method to run autotest outside the test plan.
* @param autoTestPostModel (optional)
* @return AutoTestModel
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun createAutoTest(autoTestPostModel: AutoTestPostModel? = null) : AutoTestModel {
val localVarResponse = createAutoTestWithHttpInfo(autoTestPostModel = autoTestPostModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as AutoTestModel
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Create autotest
* This method creates a new autotest. To add an autotest to the test plan, link it to a work item using the `POST /api/v2/autoTests/{autoTestId}/workItems` method. Use the `POST /api/v2/testRuns/byAutoTests` method to run autotest outside the test plan.
* @param autoTestPostModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun createAutoTestWithHttpInfo(autoTestPostModel: AutoTestPostModel?) : ApiResponse {
val localVariableConfig = createAutoTestRequestConfig(autoTestPostModel = autoTestPostModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation createAutoTest
*
* @param autoTestPostModel (optional)
* @return RequestConfig
*/
fun createAutoTestRequestConfig(autoTestPostModel: AutoTestPostModel?) : RequestConfig {
val localVariableBody = autoTestPostModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/autoTests",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Create multiple autotests
* Use case User sets autotest parameters (listed in the example) and runs method execution System creates autotest [Optional] If steps enumeration is set, system creates step items and relates them to autotest [Optional] If setup enumeration is set, system creates setup items and relates them to autotest [Optional] If teardown enumeration is set, system creates teardown items and relates them to autotest [Optional] If label enumeration is set, system creates labels and relates them to autotest [Optional] If link enumeration is set, system creates links and relates them to autotest System returns autotest model (example listed in response parameters)
* @param autoTestPostModel (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun createMultiple(autoTestPostModel: kotlin.collections.List? = null) : kotlin.collections.List {
val localVarResponse = createMultipleWithHttpInfo(autoTestPostModel = autoTestPostModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Create multiple autotests
* Use case User sets autotest parameters (listed in the example) and runs method execution System creates autotest [Optional] If steps enumeration is set, system creates step items and relates them to autotest [Optional] If setup enumeration is set, system creates setup items and relates them to autotest [Optional] If teardown enumeration is set, system creates teardown items and relates them to autotest [Optional] If label enumeration is set, system creates labels and relates them to autotest [Optional] If link enumeration is set, system creates links and relates them to autotest System returns autotest model (example listed in response parameters)
* @param autoTestPostModel (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun createMultipleWithHttpInfo(autoTestPostModel: kotlin.collections.List?) : ApiResponse?> {
val localVariableConfig = createMultipleRequestConfig(autoTestPostModel = autoTestPostModel)
return request, kotlin.collections.List>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation createMultiple
*
* @param autoTestPostModel (optional)
* @return RequestConfig
*/
fun createMultipleRequestConfig(autoTestPostModel: kotlin.collections.List?) : RequestConfig> {
val localVariableBody = autoTestPostModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/autoTests/bulk",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Delete autotest
* Use case User sets autotest internal (guid format) or global (integer format) identifier and runs method execution System finds the autotest by the identifier System deletes autotest and returns no content response
* @param id Autotest internal (UUID) or global (integer) identifier
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun deleteAutoTest(id: kotlin.String) : Unit {
val localVarResponse = deleteAutoTestWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Delete autotest
* Use case User sets autotest internal (guid format) or global (integer format) identifier and runs method execution System finds the autotest by the identifier System deletes autotest and returns no content response
* @param id Autotest internal (UUID) or global (integer) identifier
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun deleteAutoTestWithHttpInfo(id: kotlin.String) : ApiResponse {
val localVariableConfig = deleteAutoTestRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation deleteAutoTest
*
* @param id Autotest internal (UUID) or global (integer) identifier
* @return RequestConfig
*/
fun deleteAutoTestRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.DELETE,
path = "/api/v2/autoTests/{id}".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Unlink autotest from work item
* Use case User sets autotest internal (guid format) or global (integer format) identifier [Optional] User sets workitem internal (guid format) or global (integer format) identifier User runs method execution System finds the autotest by the autotest identifier [Optional] if workitem id is set by User, System finds the workitem by the workitem identifier and unlinks it from autotest. [Optional] Otherwise, if workitem id is not specified, System unlinks all workitems linked to autotest. System returns no content response
* @param id Autotest internal (UUID) or global (integer) identifier
* @param workItemId Work item internal (UUID) or global (integer) identifier (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun deleteAutoTestLinkFromWorkItem(id: kotlin.String, workItemId: kotlin.String? = null) : Unit {
val localVarResponse = deleteAutoTestLinkFromWorkItemWithHttpInfo(id = id, workItemId = workItemId)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Unlink autotest from work item
* Use case User sets autotest internal (guid format) or global (integer format) identifier [Optional] User sets workitem internal (guid format) or global (integer format) identifier User runs method execution System finds the autotest by the autotest identifier [Optional] if workitem id is set by User, System finds the workitem by the workitem identifier and unlinks it from autotest. [Optional] Otherwise, if workitem id is not specified, System unlinks all workitems linked to autotest. System returns no content response
* @param id Autotest internal (UUID) or global (integer) identifier
* @param workItemId Work item internal (UUID) or global (integer) identifier (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun deleteAutoTestLinkFromWorkItemWithHttpInfo(id: kotlin.String, workItemId: kotlin.String?) : ApiResponse {
val localVariableConfig = deleteAutoTestLinkFromWorkItemRequestConfig(id = id, workItemId = workItemId)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation deleteAutoTestLinkFromWorkItem
*
* @param id Autotest internal (UUID) or global (integer) identifier
* @param workItemId Work item internal (UUID) or global (integer) identifier (optional)
* @return RequestConfig
*/
fun deleteAutoTestLinkFromWorkItemRequestConfig(id: kotlin.String, workItemId: kotlin.String?) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (workItemId != null) {
put("workItemId", listOf(workItemId.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.DELETE,
path = "/api/v2/autoTests/{id}/workItems".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
*
*
* @param projectId Project internal ID (optional)
* @param externalId Autotest external ID (optional)
* @param globalId Autotest global ID (optional)
* @param namespace Namespace in which autotest is located (optional)
* @param isNamespaceNull OBSOLETE: Use `includeEmptyNamespaces` instead (optional)
* @param includeEmptyNamespaces If result must contain autotests without namespace (optional)
* @param className Name of class in which autotest is located (optional)
* @param isClassnameNull OBSOLETE: Use `includeEmptyClassNames` instead (optional)
* @param includeEmptyClassNames If result must contain autotests without class (optional)
* @param isDeleted OBSOLETE: Use `deleted` instead (optional)
* @param deleted Is autotest deleted (optional)
* @param labels Include only autotests with provided labels (optional)
* @param stabilityMinimal OBSOLETE: Use `minStability` instead (optional)
* @param minStability Minimum stability value of autotest (optional)
* @param stabilityMaximal OBSOLETE: Use `maxStability` instead (optional)
* @param maxStability Maximum stability value of autotest (optional)
* @param isFlaky OBSOLETE: Use `flaky` instead (optional)
* @param flaky Is autotest marked as \"Flaky\" (optional)
* @param includeSteps If result must also include autotest steps (optional)
* @param includeLabels If result must also include autotest labels (optional)
* @param externalKey External key of autotest (optional)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
@Deprecated(message = "This operation is deprecated.")
fun getAllAutoTests(projectId: java.util.UUID? = null, externalId: kotlin.String? = null, globalId: kotlin.Long? = null, namespace: kotlin.String? = null, isNamespaceNull: kotlin.Boolean? = null, includeEmptyNamespaces: kotlin.Boolean? = null, className: kotlin.String? = null, isClassnameNull: kotlin.Boolean? = null, includeEmptyClassNames: kotlin.Boolean? = null, isDeleted: kotlin.Boolean? = null, deleted: kotlin.Boolean? = null, labels: kotlin.collections.List? = null, stabilityMinimal: kotlin.Int? = null, minStability: kotlin.Int? = null, stabilityMaximal: kotlin.Int? = null, maxStability: kotlin.Int? = null, isFlaky: kotlin.Boolean? = null, flaky: kotlin.Boolean? = null, includeSteps: kotlin.Boolean? = null, includeLabels: kotlin.Boolean? = null, externalKey: kotlin.String? = null, skip: kotlin.Int? = null, take: kotlin.Int? = null, orderBy: kotlin.String? = null, searchField: kotlin.String? = null, searchValue: kotlin.String? = null) : kotlin.collections.List {
@Suppress("DEPRECATION")
val localVarResponse = getAllAutoTestsWithHttpInfo(projectId = projectId, externalId = externalId, globalId = globalId, namespace = namespace, isNamespaceNull = isNamespaceNull, includeEmptyNamespaces = includeEmptyNamespaces, className = className, isClassnameNull = isClassnameNull, includeEmptyClassNames = includeEmptyClassNames, isDeleted = isDeleted, deleted = deleted, labels = labels, stabilityMinimal = stabilityMinimal, minStability = minStability, stabilityMaximal = stabilityMaximal, maxStability = maxStability, isFlaky = isFlaky, flaky = flaky, includeSteps = includeSteps, includeLabels = includeLabels, externalKey = externalKey, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
*
*
* @param projectId Project internal ID (optional)
* @param externalId Autotest external ID (optional)
* @param globalId Autotest global ID (optional)
* @param namespace Namespace in which autotest is located (optional)
* @param isNamespaceNull OBSOLETE: Use `includeEmptyNamespaces` instead (optional)
* @param includeEmptyNamespaces If result must contain autotests without namespace (optional)
* @param className Name of class in which autotest is located (optional)
* @param isClassnameNull OBSOLETE: Use `includeEmptyClassNames` instead (optional)
* @param includeEmptyClassNames If result must contain autotests without class (optional)
* @param isDeleted OBSOLETE: Use `deleted` instead (optional)
* @param deleted Is autotest deleted (optional)
* @param labels Include only autotests with provided labels (optional)
* @param stabilityMinimal OBSOLETE: Use `minStability` instead (optional)
* @param minStability Minimum stability value of autotest (optional)
* @param stabilityMaximal OBSOLETE: Use `maxStability` instead (optional)
* @param maxStability Maximum stability value of autotest (optional)
* @param isFlaky OBSOLETE: Use `flaky` instead (optional)
* @param flaky Is autotest marked as \"Flaky\" (optional)
* @param includeSteps If result must also include autotest steps (optional)
* @param includeLabels If result must also include autotest labels (optional)
* @param externalKey External key of autotest (optional)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
@Deprecated(message = "This operation is deprecated.")
fun getAllAutoTestsWithHttpInfo(projectId: java.util.UUID?, externalId: kotlin.String?, globalId: kotlin.Long?, namespace: kotlin.String?, isNamespaceNull: kotlin.Boolean?, includeEmptyNamespaces: kotlin.Boolean?, className: kotlin.String?, isClassnameNull: kotlin.Boolean?, includeEmptyClassNames: kotlin.Boolean?, isDeleted: kotlin.Boolean?, deleted: kotlin.Boolean?, labels: kotlin.collections.List?, stabilityMinimal: kotlin.Int?, minStability: kotlin.Int?, stabilityMaximal: kotlin.Int?, maxStability: kotlin.Int?, isFlaky: kotlin.Boolean?, flaky: kotlin.Boolean?, includeSteps: kotlin.Boolean?, includeLabels: kotlin.Boolean?, externalKey: kotlin.String?, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?) : ApiResponse?> {
@Suppress("DEPRECATION")
val localVariableConfig = getAllAutoTestsRequestConfig(projectId = projectId, externalId = externalId, globalId = globalId, namespace = namespace, isNamespaceNull = isNamespaceNull, includeEmptyNamespaces = includeEmptyNamespaces, className = className, isClassnameNull = isClassnameNull, includeEmptyClassNames = includeEmptyClassNames, isDeleted = isDeleted, deleted = deleted, labels = labels, stabilityMinimal = stabilityMinimal, minStability = minStability, stabilityMaximal = stabilityMaximal, maxStability = maxStability, isFlaky = isFlaky, flaky = flaky, includeSteps = includeSteps, includeLabels = includeLabels, externalKey = externalKey, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getAllAutoTests
*
* @param projectId Project internal ID (optional)
* @param externalId Autotest external ID (optional)
* @param globalId Autotest global ID (optional)
* @param namespace Namespace in which autotest is located (optional)
* @param isNamespaceNull OBSOLETE: Use `includeEmptyNamespaces` instead (optional)
* @param includeEmptyNamespaces If result must contain autotests without namespace (optional)
* @param className Name of class in which autotest is located (optional)
* @param isClassnameNull OBSOLETE: Use `includeEmptyClassNames` instead (optional)
* @param includeEmptyClassNames If result must contain autotests without class (optional)
* @param isDeleted OBSOLETE: Use `deleted` instead (optional)
* @param deleted Is autotest deleted (optional)
* @param labels Include only autotests with provided labels (optional)
* @param stabilityMinimal OBSOLETE: Use `minStability` instead (optional)
* @param minStability Minimum stability value of autotest (optional)
* @param stabilityMaximal OBSOLETE: Use `maxStability` instead (optional)
* @param maxStability Maximum stability value of autotest (optional)
* @param isFlaky OBSOLETE: Use `flaky` instead (optional)
* @param flaky Is autotest marked as \"Flaky\" (optional)
* @param includeSteps If result must also include autotest steps (optional)
* @param includeLabels If result must also include autotest labels (optional)
* @param externalKey External key of autotest (optional)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return RequestConfig
*/
@Deprecated(message = "This operation is deprecated.")
fun getAllAutoTestsRequestConfig(projectId: java.util.UUID?, externalId: kotlin.String?, globalId: kotlin.Long?, namespace: kotlin.String?, isNamespaceNull: kotlin.Boolean?, includeEmptyNamespaces: kotlin.Boolean?, className: kotlin.String?, isClassnameNull: kotlin.Boolean?, includeEmptyClassNames: kotlin.Boolean?, isDeleted: kotlin.Boolean?, deleted: kotlin.Boolean?, labels: kotlin.collections.List?, stabilityMinimal: kotlin.Int?, minStability: kotlin.Int?, stabilityMaximal: kotlin.Int?, maxStability: kotlin.Int?, isFlaky: kotlin.Boolean?, flaky: kotlin.Boolean?, includeSteps: kotlin.Boolean?, includeLabels: kotlin.Boolean?, externalKey: kotlin.String?, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (projectId != null) {
put("projectId", listOf(projectId.toString()))
}
if (externalId != null) {
put("externalId", listOf(externalId.toString()))
}
if (globalId != null) {
put("globalId", listOf(globalId.toString()))
}
if (namespace != null) {
put("namespace", listOf(namespace.toString()))
}
if (isNamespaceNull != null) {
put("isNamespaceNull", listOf(isNamespaceNull.toString()))
}
if (includeEmptyNamespaces != null) {
put("includeEmptyNamespaces", listOf(includeEmptyNamespaces.toString()))
}
if (className != null) {
put("className", listOf(className.toString()))
}
if (isClassnameNull != null) {
put("isClassnameNull", listOf(isClassnameNull.toString()))
}
if (includeEmptyClassNames != null) {
put("includeEmptyClassNames", listOf(includeEmptyClassNames.toString()))
}
if (isDeleted != null) {
put("isDeleted", listOf(isDeleted.toString()))
}
if (deleted != null) {
put("deleted", listOf(deleted.toString()))
}
if (labels != null) {
put("labels", toMultiValue(labels.toList(), "multi"))
}
if (stabilityMinimal != null) {
put("stabilityMinimal", listOf(stabilityMinimal.toString()))
}
if (minStability != null) {
put("minStability", listOf(minStability.toString()))
}
if (stabilityMaximal != null) {
put("stabilityMaximal", listOf(stabilityMaximal.toString()))
}
if (maxStability != null) {
put("maxStability", listOf(maxStability.toString()))
}
if (isFlaky != null) {
put("isFlaky", listOf(isFlaky.toString()))
}
if (flaky != null) {
put("flaky", listOf(flaky.toString()))
}
if (includeSteps != null) {
put("includeSteps", listOf(includeSteps.toString()))
}
if (includeLabels != null) {
put("includeLabels", listOf(includeLabels.toString()))
}
if (externalKey != null) {
put("externalKey", listOf(externalKey.toString()))
}
if (skip != null) {
put("Skip", listOf(skip.toString()))
}
if (take != null) {
put("Take", listOf(take.toString()))
}
if (orderBy != null) {
put("OrderBy", listOf(orderBy.toString()))
}
if (searchField != null) {
put("SearchField", listOf(searchField.toString()))
}
if (searchValue != null) {
put("SearchValue", listOf(searchValue.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/autoTests",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get average autotest duration
* Use case User sets autotest internal (guid format) or global (integer format) identifier User runs method execution System calculates pass average duration and fail average duration of autotest from all related test results System returns pass average duration and fail average duration for autotest
* @param id Autotest internal (UUID) or global (integer) identifier
* @return AutoTestAverageDurationModel
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun getAutoTestAverageDuration(id: kotlin.String) : AutoTestAverageDurationModel {
val localVarResponse = getAutoTestAverageDurationWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as AutoTestAverageDurationModel
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get average autotest duration
* Use case User sets autotest internal (guid format) or global (integer format) identifier User runs method execution System calculates pass average duration and fail average duration of autotest from all related test results System returns pass average duration and fail average duration for autotest
* @param id Autotest internal (UUID) or global (integer) identifier
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun getAutoTestAverageDurationWithHttpInfo(id: kotlin.String) : ApiResponse {
val localVariableConfig = getAutoTestAverageDurationRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getAutoTestAverageDuration
*
* @param id Autotest internal (UUID) or global (integer) identifier
* @return RequestConfig
*/
fun getAutoTestAverageDurationRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/autoTests/{id}/averageDuration".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get autotest by internal or global ID
* Use case User sets autotest internal or global identifier and runs method execution System returns autotest, which internal or global identifier equals the identifier value set in the previous action
* @param id Autotest internal (UUID) or global (integer) identifier
* @return AutoTestModel
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun getAutoTestById(id: kotlin.String) : AutoTestModel {
val localVarResponse = getAutoTestByIdWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as AutoTestModel
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get autotest by internal or global ID
* Use case User sets autotest internal or global identifier and runs method execution System returns autotest, which internal or global identifier equals the identifier value set in the previous action
* @param id Autotest internal (UUID) or global (integer) identifier
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun getAutoTestByIdWithHttpInfo(id: kotlin.String) : ApiResponse {
val localVariableConfig = getAutoTestByIdRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getAutoTestById
*
* @param id Autotest internal (UUID) or global (integer) identifier
* @return RequestConfig
*/
fun getAutoTestByIdRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/autoTests/{id}".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get autotest chronology
* Use case User sets autotest internal (guid format) or global (integer format) identifier User runs method execution System search all test results related to autotest (with default limit equal 100) System orders the test results by CompletedOn property descending and then orders by CreatedDate property descending System returns test result chronology for autotest
* @param id Autotest internal (UUID) or global (integer) identifier
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun getAutoTestChronology(id: kotlin.String) : kotlin.collections.List {
val localVarResponse = getAutoTestChronologyWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get autotest chronology
* Use case User sets autotest internal (guid format) or global (integer format) identifier User runs method execution System search all test results related to autotest (with default limit equal 100) System orders the test results by CompletedOn property descending and then orders by CreatedDate property descending System returns test result chronology for autotest
* @param id Autotest internal (UUID) or global (integer) identifier
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun getAutoTestChronologyWithHttpInfo(id: kotlin.String) : ApiResponse?> {
val localVariableConfig = getAutoTestChronologyRequestConfig(id = id)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getAutoTestChronology
*
* @param id Autotest internal (UUID) or global (integer) identifier
* @return RequestConfig
*/
fun getAutoTestChronologyRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/autoTests/{id}/chronology".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get completed tests runs for autotests
* Use case User sets autotest internal (guid format) or global (integer format) identifier User runs method execution System search for all test runs related to the autotest System returns the enumeration of test runs
* @param id Autotest internal (UUID) or global (integer) identifier
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun getTestRuns(id: kotlin.String) : kotlin.collections.List {
val localVarResponse = getTestRunsWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get completed tests runs for autotests
* Use case User sets autotest internal (guid format) or global (integer format) identifier User runs method execution System search for all test runs related to the autotest System returns the enumeration of test runs
* @param id Autotest internal (UUID) or global (integer) identifier
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun getTestRunsWithHttpInfo(id: kotlin.String) : ApiResponse?> {
val localVariableConfig = getTestRunsRequestConfig(id = id)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getTestRuns
*
* @param id Autotest internal (UUID) or global (integer) identifier
* @return RequestConfig
*/
fun getTestRunsRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/autoTests/{id}/testRuns".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
*
*
* @param id
* @param from Take results from this date (optional)
* @param to Take results until this date (optional)
* @param configurationIds Identifiers of test result configurations (optional)
* @param testPlanIds Identifiers of test plans which contain test results (optional)
* @param userIds Identifiers of users who set test results (optional)
* @param outcomes List of outcomes of test results (optional)
* @param isAutomated OBSOLETE: Use `Automated` instead (optional)
* @param automated If result must consist of only manual/automated test results (optional)
* @param testRunIds Identifiers of test runs which contain test results (optional)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
@Deprecated(message = "This operation is deprecated.")
fun getWorkItemResults(id: kotlin.String, from: java.time.OffsetDateTime? = null, to: java.time.OffsetDateTime? = null, configurationIds: kotlin.collections.List? = null, testPlanIds: kotlin.collections.List? = null, userIds: kotlin.collections.List? = null, outcomes: kotlin.collections.List? = null, isAutomated: kotlin.Boolean? = null, automated: kotlin.Boolean? = null, testRunIds: kotlin.collections.List? = null, skip: kotlin.Int? = null, take: kotlin.Int? = null, orderBy: kotlin.String? = null, searchField: kotlin.String? = null, searchValue: kotlin.String? = null) : kotlin.collections.List {
@Suppress("DEPRECATION")
val localVarResponse = getWorkItemResultsWithHttpInfo(id = id, from = from, to = to, configurationIds = configurationIds, testPlanIds = testPlanIds, userIds = userIds, outcomes = outcomes, isAutomated = isAutomated, automated = automated, testRunIds = testRunIds, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
*
*
* @param id
* @param from Take results from this date (optional)
* @param to Take results until this date (optional)
* @param configurationIds Identifiers of test result configurations (optional)
* @param testPlanIds Identifiers of test plans which contain test results (optional)
* @param userIds Identifiers of users who set test results (optional)
* @param outcomes List of outcomes of test results (optional)
* @param isAutomated OBSOLETE: Use `Automated` instead (optional)
* @param automated If result must consist of only manual/automated test results (optional)
* @param testRunIds Identifiers of test runs which contain test results (optional)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
@Deprecated(message = "This operation is deprecated.")
fun getWorkItemResultsWithHttpInfo(id: kotlin.String, from: java.time.OffsetDateTime?, to: java.time.OffsetDateTime?, configurationIds: kotlin.collections.List?, testPlanIds: kotlin.collections.List?, userIds: kotlin.collections.List?, outcomes: kotlin.collections.List?, isAutomated: kotlin.Boolean?, automated: kotlin.Boolean?, testRunIds: kotlin.collections.List?, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?) : ApiResponse?> {
@Suppress("DEPRECATION")
val localVariableConfig = getWorkItemResultsRequestConfig(id = id, from = from, to = to, configurationIds = configurationIds, testPlanIds = testPlanIds, userIds = userIds, outcomes = outcomes, isAutomated = isAutomated, automated = automated, testRunIds = testRunIds, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getWorkItemResults
*
* @param id
* @param from Take results from this date (optional)
* @param to Take results until this date (optional)
* @param configurationIds Identifiers of test result configurations (optional)
* @param testPlanIds Identifiers of test plans which contain test results (optional)
* @param userIds Identifiers of users who set test results (optional)
* @param outcomes List of outcomes of test results (optional)
* @param isAutomated OBSOLETE: Use `Automated` instead (optional)
* @param automated If result must consist of only manual/automated test results (optional)
* @param testRunIds Identifiers of test runs which contain test results (optional)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return RequestConfig
*/
@Deprecated(message = "This operation is deprecated.")
fun getWorkItemResultsRequestConfig(id: kotlin.String, from: java.time.OffsetDateTime?, to: java.time.OffsetDateTime?, configurationIds: kotlin.collections.List?, testPlanIds: kotlin.collections.List?, userIds: kotlin.collections.List?, outcomes: kotlin.collections.List?, isAutomated: kotlin.Boolean?, automated: kotlin.Boolean?, testRunIds: kotlin.collections.List?, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (from != null) {
put("from", listOf(parseDateToQueryString(from)))
}
if (to != null) {
put("to", listOf(parseDateToQueryString(to)))
}
if (configurationIds != null) {
put("configurationIds", toMultiValue(configurationIds.toList(), "multi"))
}
if (testPlanIds != null) {
put("testPlanIds", toMultiValue(testPlanIds.toList(), "multi"))
}
if (userIds != null) {
put("userIds", toMultiValue(userIds.toList(), "multi"))
}
if (outcomes != null) {
put("outcomes", toMultiValue(outcomes.toList(), "multi"))
}
if (isAutomated != null) {
put("isAutomated", listOf(isAutomated.toString()))
}
if (automated != null) {
put("automated", listOf(automated.toString()))
}
if (testRunIds != null) {
put("testRunIds", toMultiValue(testRunIds.toList(), "multi"))
}
if (skip != null) {
put("Skip", listOf(skip.toString()))
}
if (take != null) {
put("Take", listOf(take.toString()))
}
if (orderBy != null) {
put("OrderBy", listOf(orderBy.toString()))
}
if (searchField != null) {
put("SearchField", listOf(searchField.toString()))
}
if (searchValue != null) {
put("SearchValue", listOf(searchValue.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/autoTests/{id}/testResultHistory".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get work items linked to autotest
* This method links an autotest to a test case or a checklist. A manual test case with a linked automated work item is marked in the test management system as an autotest. You can run it from graphical user interface (GUI). To do that: 1. Open the project in GUI. 2. Go to <b>Test plans</b> section and switch to the <b>Execution</b> tab. 3. Select the autotest(s) you want to run using checkboxes. 4. In the toolbar above the test list, click <b>Run autotests</b>.
* @param id Specifies the autotest entity ID. You can copy it from the address bar in your web browser or use autotest GUID.
* @param isDeleted Specifies that a test is deleted or still relevant. (optional)
* @param isWorkItemDeleted OBSOLETE: Use `isDeleted` instead (optional, default to false)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun getWorkItemsLinkedToAutoTest(id: kotlin.String, isDeleted: kotlin.Boolean? = null, isWorkItemDeleted: kotlin.Boolean? = false) : kotlin.collections.List {
val localVarResponse = getWorkItemsLinkedToAutoTestWithHttpInfo(id = id, isDeleted = isDeleted, isWorkItemDeleted = isWorkItemDeleted)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get work items linked to autotest
* This method links an autotest to a test case or a checklist. A manual test case with a linked automated work item is marked in the test management system as an autotest. You can run it from graphical user interface (GUI). To do that: 1. Open the project in GUI. 2. Go to <b>Test plans</b> section and switch to the <b>Execution</b> tab. 3. Select the autotest(s) you want to run using checkboxes. 4. In the toolbar above the test list, click <b>Run autotests</b>.
* @param id Specifies the autotest entity ID. You can copy it from the address bar in your web browser or use autotest GUID.
* @param isDeleted Specifies that a test is deleted or still relevant. (optional)
* @param isWorkItemDeleted OBSOLETE: Use `isDeleted` instead (optional, default to false)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun getWorkItemsLinkedToAutoTestWithHttpInfo(id: kotlin.String, isDeleted: kotlin.Boolean?, isWorkItemDeleted: kotlin.Boolean?) : ApiResponse?> {
val localVariableConfig = getWorkItemsLinkedToAutoTestRequestConfig(id = id, isDeleted = isDeleted, isWorkItemDeleted = isWorkItemDeleted)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getWorkItemsLinkedToAutoTest
*
* @param id Specifies the autotest entity ID. You can copy it from the address bar in your web browser or use autotest GUID.
* @param isDeleted Specifies that a test is deleted or still relevant. (optional)
* @param isWorkItemDeleted OBSOLETE: Use `isDeleted` instead (optional, default to false)
* @return RequestConfig
*/
fun getWorkItemsLinkedToAutoTestRequestConfig(id: kotlin.String, isDeleted: kotlin.Boolean?, isWorkItemDeleted: kotlin.Boolean?) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (isDeleted != null) {
put("isDeleted", listOf(isDeleted.toString()))
}
if (isWorkItemDeleted != null) {
put("isWorkItemDeleted", listOf(isWorkItemDeleted.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/autoTests/{id}/workItems".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Link autotest with work items
* Use case User sets autotest internal (guid format) or global (integer format) identifier User sets work item internal (guid format) or global (integer format) identifier User runs method execution System finds the autotest by the autotest identifier System finds the work item by the work item identifier System relates the work item with the autotest and returns no content response
* @param id Autotest internal (UUID) or global (integer) identifier
* @param workItemIdModel (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun linkAutoTestToWorkItem(id: kotlin.String, workItemIdModel: WorkItemIdModel? = null) : Unit {
val localVarResponse = linkAutoTestToWorkItemWithHttpInfo(id = id, workItemIdModel = workItemIdModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Link autotest with work items
* Use case User sets autotest internal (guid format) or global (integer format) identifier User sets work item internal (guid format) or global (integer format) identifier User runs method execution System finds the autotest by the autotest identifier System finds the work item by the work item identifier System relates the work item with the autotest and returns no content response
* @param id Autotest internal (UUID) or global (integer) identifier
* @param workItemIdModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun linkAutoTestToWorkItemWithHttpInfo(id: kotlin.String, workItemIdModel: WorkItemIdModel?) : ApiResponse {
val localVariableConfig = linkAutoTestToWorkItemRequestConfig(id = id, workItemIdModel = workItemIdModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation linkAutoTestToWorkItem
*
* @param id Autotest internal (UUID) or global (integer) identifier
* @param workItemIdModel (optional)
* @return RequestConfig
*/
fun linkAutoTestToWorkItemRequestConfig(id: kotlin.String, workItemIdModel: WorkItemIdModel?) : RequestConfig {
val localVariableBody = workItemIdModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/autoTests/{id}/workItems".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Update autotest
* Use case User sets autotest updated parameters values (listed in the example) and runs method execution System finds the autotest by the identifier System updates autotest parameters [Optional] If steps enumeration is set, system creates step items, relates them to autotest and deletes relations with current steps( if exist) [Optional] If Setup enumeration is set, system creates setup items and relates them to autotest and deletes relations with current Setup items (if exist) [Optional] If teardown enumeration is set, system creates teardown items and relates them to autotest and deletes relations with current teardown items (if exist) [Optional] If label enumeration is set, system creates labels and relates them to autotest and deletes relations with current Labels (if exist) [Optional] If link enumeration is set, system creates links and relates them to autotest and deletes relations with current Links (if exist) System updates autotest and returns no content response
* @param autoTestPutModel (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun updateAutoTest(autoTestPutModel: AutoTestPutModel? = null) : Unit {
val localVarResponse = updateAutoTestWithHttpInfo(autoTestPutModel = autoTestPutModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Update autotest
* Use case User sets autotest updated parameters values (listed in the example) and runs method execution System finds the autotest by the identifier System updates autotest parameters [Optional] If steps enumeration is set, system creates step items, relates them to autotest and deletes relations with current steps( if exist) [Optional] If Setup enumeration is set, system creates setup items and relates them to autotest and deletes relations with current Setup items (if exist) [Optional] If teardown enumeration is set, system creates teardown items and relates them to autotest and deletes relations with current teardown items (if exist) [Optional] If label enumeration is set, system creates labels and relates them to autotest and deletes relations with current Labels (if exist) [Optional] If link enumeration is set, system creates links and relates them to autotest and deletes relations with current Links (if exist) System updates autotest and returns no content response
* @param autoTestPutModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun updateAutoTestWithHttpInfo(autoTestPutModel: AutoTestPutModel?) : ApiResponse {
val localVariableConfig = updateAutoTestRequestConfig(autoTestPutModel = autoTestPutModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation updateAutoTest
*
* @param autoTestPutModel (optional)
* @return RequestConfig
*/
fun updateAutoTestRequestConfig(autoTestPutModel: AutoTestPutModel?) : RequestConfig {
val localVariableBody = autoTestPutModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.PUT,
path = "/api/v2/autoTests",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Update multiple autotests
* Use case User sets autotest updated parameters values (listed in the example) and runs method execution System finds the autotest by the identifier System updates autotest parameters [Optional] If steps enumeration is set, system creates step items, relates them to autotest and deletes relations with current steps( if exist) [Optional] If Setup enumeration is set, system creates setup items and relates them to autotest and deletes relations with current Setup items (if exist) [Optional] If teardown enumeration is set, system creates teardown items and relates them to autotest and deletes relations with current teardown items (if exist) [Optional] If label enumeration is set, system creates labels and relates them to autotest and deletes relations with current Labels (if exist) [Optional] If link enumeration is set, system creates links and relates them to autotest and deletes relations with current Links (if exist) System updates autotest and returns no content response
* @param autoTestPutModel (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun updateMultiple(autoTestPutModel: kotlin.collections.List? = null) : Unit {
val localVarResponse = updateMultipleWithHttpInfo(autoTestPutModel = autoTestPutModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Update multiple autotests
* Use case User sets autotest updated parameters values (listed in the example) and runs method execution System finds the autotest by the identifier System updates autotest parameters [Optional] If steps enumeration is set, system creates step items, relates them to autotest and deletes relations with current steps( if exist) [Optional] If Setup enumeration is set, system creates setup items and relates them to autotest and deletes relations with current Setup items (if exist) [Optional] If teardown enumeration is set, system creates teardown items and relates them to autotest and deletes relations with current teardown items (if exist) [Optional] If label enumeration is set, system creates labels and relates them to autotest and deletes relations with current Labels (if exist) [Optional] If link enumeration is set, system creates links and relates them to autotest and deletes relations with current Links (if exist) System updates autotest and returns no content response
* @param autoTestPutModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun updateMultipleWithHttpInfo(autoTestPutModel: kotlin.collections.List?) : ApiResponse {
val localVariableConfig = updateMultipleRequestConfig(autoTestPutModel = autoTestPutModel)
return request, Unit>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation updateMultiple
*
* @param autoTestPutModel (optional)
* @return RequestConfig
*/
fun updateMultipleRequestConfig(autoTestPutModel: kotlin.collections.List?) : RequestConfig> {
val localVariableBody = autoTestPutModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.PUT,
path = "/api/v2/autoTests/bulk",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
private fun encodeURIComponent(uriComponent: kotlin.String): kotlin.String =
HttpUrl.Builder().scheme("http").host("localhost").addPathSegment(uriComponent).build().encodedPathSegments[0]
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy