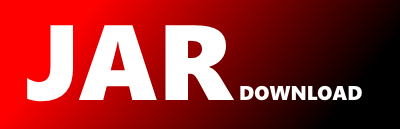
ru.testit.kotlin.client.apis.ParametersApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of testit-api-client-kotlin Show documentation
Show all versions of testit-api-client-kotlin Show documentation
Kotlin API client for TestIT.
/**
*
* Please note:
* This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit this file manually.
*
*/
@file:Suppress(
"ArrayInDataClass",
"EnumEntryName",
"RemoveRedundantQualifierName",
"UnusedImport"
)
package ru.testit.kotlin.client.apis
import java.io.IOException
import okhttp3.OkHttpClient
import okhttp3.HttpUrl
import ru.testit.kotlin.client.models.ParameterFilterModel
import ru.testit.kotlin.client.models.ParameterGroupModel
import ru.testit.kotlin.client.models.ParameterModel
import ru.testit.kotlin.client.models.ParameterPostModel
import ru.testit.kotlin.client.models.ParameterPutModel
import ru.testit.kotlin.client.models.ProblemDetails
import ru.testit.kotlin.client.models.ValidationProblemDetails
import com.squareup.moshi.Json
import ru.testit.kotlin.client.infrastructure.ApiClient
import ru.testit.kotlin.client.infrastructure.ApiResponse
import ru.testit.kotlin.client.infrastructure.ClientException
import ru.testit.kotlin.client.infrastructure.ClientError
import ru.testit.kotlin.client.infrastructure.ServerException
import ru.testit.kotlin.client.infrastructure.ServerError
import ru.testit.kotlin.client.infrastructure.MultiValueMap
import ru.testit.kotlin.client.infrastructure.PartConfig
import ru.testit.kotlin.client.infrastructure.RequestConfig
import ru.testit.kotlin.client.infrastructure.RequestMethod
import ru.testit.kotlin.client.infrastructure.ResponseType
import ru.testit.kotlin.client.infrastructure.Success
import ru.testit.kotlin.client.infrastructure.toMultiValue
class ParametersApi(basePath: kotlin.String = defaultBasePath, client: OkHttpClient = ApiClient.defaultClient) : ApiClient(basePath, client) {
companion object {
@JvmStatic
val defaultBasePath: String by lazy {
System.getProperties().getProperty(ApiClient.baseUrlKey, "http://localhost")
}
}
/**
* Create multiple parameters
* Use case User sets list of parameter model (listed in the request example) User runs method execution System creates parameters System returns list of parameter model (listed in the response example)
* @param parameterPostModel (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2ParametersBulkPost(parameterPostModel: kotlin.collections.List? = null) : kotlin.collections.List {
val localVarResponse = apiV2ParametersBulkPostWithHttpInfo(parameterPostModel = parameterPostModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Create multiple parameters
* Use case User sets list of parameter model (listed in the request example) User runs method execution System creates parameters System returns list of parameter model (listed in the response example)
* @param parameterPostModel (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2ParametersBulkPostWithHttpInfo(parameterPostModel: kotlin.collections.List?) : ApiResponse?> {
val localVariableConfig = apiV2ParametersBulkPostRequestConfig(parameterPostModel = parameterPostModel)
return request, kotlin.collections.List>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ParametersBulkPost
*
* @param parameterPostModel (optional)
* @return RequestConfig
*/
fun apiV2ParametersBulkPostRequestConfig(parameterPostModel: kotlin.collections.List?) : RequestConfig> {
val localVariableBody = parameterPostModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/parameters/bulk",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Update multiple parameters
* Use case User sets list of parameter model (listed in the request example) User runs method execution System updates parameters
* @param parameterPutModel (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2ParametersBulkPut(parameterPutModel: kotlin.collections.List? = null) : Unit {
val localVarResponse = apiV2ParametersBulkPutWithHttpInfo(parameterPutModel = parameterPutModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Update multiple parameters
* Use case User sets list of parameter model (listed in the request example) User runs method execution System updates parameters
* @param parameterPutModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2ParametersBulkPutWithHttpInfo(parameterPutModel: kotlin.collections.List?) : ApiResponse {
val localVariableConfig = apiV2ParametersBulkPutRequestConfig(parameterPutModel = parameterPutModel)
return request, Unit>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ParametersBulkPut
*
* @param parameterPutModel (optional)
* @return RequestConfig
*/
fun apiV2ParametersBulkPutRequestConfig(parameterPutModel: kotlin.collections.List?) : RequestConfig> {
val localVariableBody = parameterPutModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.PUT,
path = "/api/v2/parameters/bulk",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get parameters as group
* Use case User runs method execution System search parameters System returns parameters models as groups (listed in the response example)
* @param isDeleted (optional)
* @param parameterKeyIds (optional)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2ParametersGroupsGet(isDeleted: kotlin.Boolean? = null, parameterKeyIds: kotlin.collections.Set? = null, skip: kotlin.Int? = null, take: kotlin.Int? = null, orderBy: kotlin.String? = null, searchField: kotlin.String? = null, searchValue: kotlin.String? = null) : kotlin.collections.List {
val localVarResponse = apiV2ParametersGroupsGetWithHttpInfo(isDeleted = isDeleted, parameterKeyIds = parameterKeyIds, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get parameters as group
* Use case User runs method execution System search parameters System returns parameters models as groups (listed in the response example)
* @param isDeleted (optional)
* @param parameterKeyIds (optional)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2ParametersGroupsGetWithHttpInfo(isDeleted: kotlin.Boolean?, parameterKeyIds: kotlin.collections.Set?, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?) : ApiResponse?> {
val localVariableConfig = apiV2ParametersGroupsGetRequestConfig(isDeleted = isDeleted, parameterKeyIds = parameterKeyIds, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ParametersGroupsGet
*
* @param isDeleted (optional)
* @param parameterKeyIds (optional)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return RequestConfig
*/
fun apiV2ParametersGroupsGetRequestConfig(isDeleted: kotlin.Boolean?, parameterKeyIds: kotlin.collections.Set?, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (isDeleted != null) {
put("isDeleted", listOf(isDeleted.toString()))
}
if (parameterKeyIds != null) {
put("parameterKeyIds", toMultiValue(parameterKeyIds.toList(), "multi"))
}
if (skip != null) {
put("Skip", listOf(skip.toString()))
}
if (take != null) {
put("Take", listOf(take.toString()))
}
if (orderBy != null) {
put("OrderBy", listOf(orderBy.toString()))
}
if (searchField != null) {
put("SearchField", listOf(searchField.toString()))
}
if (searchValue != null) {
put("SearchValue", listOf(searchValue.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/parameters/groups",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Check existence parameter key in system
* Use case User sets name of parameter key User runs method execution System search parameter key System returns the flag for the existence of the parameter key in the system
* @param name
* @return kotlin.Boolean
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2ParametersKeyNameNameExistsGet(name: kotlin.String) : kotlin.Boolean {
val localVarResponse = apiV2ParametersKeyNameNameExistsGetWithHttpInfo(name = name)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.Boolean
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Check existence parameter key in system
* Use case User sets name of parameter key User runs method execution System search parameter key System returns the flag for the existence of the parameter key in the system
* @param name
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2ParametersKeyNameNameExistsGetWithHttpInfo(name: kotlin.String) : ApiResponse {
val localVariableConfig = apiV2ParametersKeyNameNameExistsGetRequestConfig(name = name)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ParametersKeyNameNameExistsGet
*
* @param name
* @return RequestConfig
*/
fun apiV2ParametersKeyNameNameExistsGetRequestConfig(name: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/parameters/key/name/{name}/exists".replace("{"+"name"+"}", encodeURIComponent(name.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get all parameter key values
* Use case User sets parameter key (string format) User runs method execution System search parameter values using the key System returns parameter
* @param key Parameter key (string format)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2ParametersKeyValuesGet(key: kotlin.String) : kotlin.collections.List {
val localVarResponse = apiV2ParametersKeyValuesGetWithHttpInfo(key = key)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get all parameter key values
* Use case User sets parameter key (string format) User runs method execution System search parameter values using the key System returns parameter
* @param key Parameter key (string format)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2ParametersKeyValuesGetWithHttpInfo(key: kotlin.String) : ApiResponse?> {
val localVariableConfig = apiV2ParametersKeyValuesGetRequestConfig(key = key)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ParametersKeyValuesGet
*
* @param key Parameter key (string format)
* @return RequestConfig
*/
fun apiV2ParametersKeyValuesGetRequestConfig(key: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/parameters/{key}/values".replace("{"+"key"+"}", encodeURIComponent(key.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get all parameter keys
* Use case User runs method execution System search all parameter keys System returns parameter keys
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2ParametersKeysGet() : kotlin.collections.List {
val localVarResponse = apiV2ParametersKeysGetWithHttpInfo()
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get all parameter keys
* Use case User runs method execution System search all parameter keys System returns parameter keys
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2ParametersKeysGetWithHttpInfo() : ApiResponse?> {
val localVariableConfig = apiV2ParametersKeysGetRequestConfig()
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ParametersKeysGet
*
* @return RequestConfig
*/
fun apiV2ParametersKeysGetRequestConfig() : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/parameters/keys",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Search for parameters as group
*
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param parameterFilterModel (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2ParametersSearchGroupsPost(skip: kotlin.Int? = null, take: kotlin.Int? = null, orderBy: kotlin.String? = null, searchField: kotlin.String? = null, searchValue: kotlin.String? = null, parameterFilterModel: ParameterFilterModel? = null) : kotlin.collections.List {
val localVarResponse = apiV2ParametersSearchGroupsPostWithHttpInfo(skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue, parameterFilterModel = parameterFilterModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Search for parameters as group
*
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param parameterFilterModel (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2ParametersSearchGroupsPostWithHttpInfo(skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?, parameterFilterModel: ParameterFilterModel?) : ApiResponse?> {
val localVariableConfig = apiV2ParametersSearchGroupsPostRequestConfig(skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue, parameterFilterModel = parameterFilterModel)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ParametersSearchGroupsPost
*
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param parameterFilterModel (optional)
* @return RequestConfig
*/
fun apiV2ParametersSearchGroupsPostRequestConfig(skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?, parameterFilterModel: ParameterFilterModel?) : RequestConfig {
val localVariableBody = parameterFilterModel
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (skip != null) {
put("Skip", listOf(skip.toString()))
}
if (take != null) {
put("Take", listOf(take.toString()))
}
if (orderBy != null) {
put("OrderBy", listOf(orderBy.toString()))
}
if (searchField != null) {
put("SearchField", listOf(searchField.toString()))
}
if (searchValue != null) {
put("SearchValue", listOf(searchValue.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/parameters/search/groups",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Search for parameters
*
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param parameterFilterModel (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2ParametersSearchPost(skip: kotlin.Int? = null, take: kotlin.Int? = null, orderBy: kotlin.String? = null, searchField: kotlin.String? = null, searchValue: kotlin.String? = null, parameterFilterModel: ParameterFilterModel? = null) : kotlin.collections.List {
val localVarResponse = apiV2ParametersSearchPostWithHttpInfo(skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue, parameterFilterModel = parameterFilterModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Search for parameters
*
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param parameterFilterModel (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2ParametersSearchPostWithHttpInfo(skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?, parameterFilterModel: ParameterFilterModel?) : ApiResponse?> {
val localVariableConfig = apiV2ParametersSearchPostRequestConfig(skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue, parameterFilterModel = parameterFilterModel)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ParametersSearchPost
*
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param parameterFilterModel (optional)
* @return RequestConfig
*/
fun apiV2ParametersSearchPostRequestConfig(skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?, parameterFilterModel: ParameterFilterModel?) : RequestConfig {
val localVariableBody = parameterFilterModel
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (skip != null) {
put("Skip", listOf(skip.toString()))
}
if (take != null) {
put("Take", listOf(take.toString()))
}
if (orderBy != null) {
put("OrderBy", listOf(orderBy.toString()))
}
if (searchField != null) {
put("SearchField", listOf(searchField.toString()))
}
if (searchValue != null) {
put("SearchValue", listOf(searchValue.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/parameters/search",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Create parameter
* Use case User sets parameter model (listed in the request example) User runs method execution System creates parameter System returns parameter model
* @param parameterPostModel (optional)
* @return ParameterModel
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun createParameter(parameterPostModel: ParameterPostModel? = null) : ParameterModel {
val localVarResponse = createParameterWithHttpInfo(parameterPostModel = parameterPostModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as ParameterModel
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Create parameter
* Use case User sets parameter model (listed in the request example) User runs method execution System creates parameter System returns parameter model
* @param parameterPostModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun createParameterWithHttpInfo(parameterPostModel: ParameterPostModel?) : ApiResponse {
val localVariableConfig = createParameterRequestConfig(parameterPostModel = parameterPostModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation createParameter
*
* @param parameterPostModel (optional)
* @return RequestConfig
*/
fun createParameterRequestConfig(parameterPostModel: ParameterPostModel?) : RequestConfig {
val localVariableBody = parameterPostModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/parameters",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Delete parameter by name
* Deletes parameter and all it's values
* @param name Name of the parameter
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun deleteByName(name: kotlin.String) : Unit {
val localVarResponse = deleteByNameWithHttpInfo(name = name)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Delete parameter by name
* Deletes parameter and all it's values
* @param name Name of the parameter
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun deleteByNameWithHttpInfo(name: kotlin.String) : ApiResponse {
val localVariableConfig = deleteByNameRequestConfig(name = name)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation deleteByName
*
* @param name Name of the parameter
* @return RequestConfig
*/
fun deleteByNameRequestConfig(name: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.DELETE,
path = "/api/v2/parameters/name/{name}".replace("{"+"name"+"}", encodeURIComponent(name.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Delete parameters by parameter key identifier
* Deletes parameter and all it's values by parameter key identifier
* @param keyId Identifier of the parameter key
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun deleteByParameterKeyId(keyId: java.util.UUID) : Unit {
val localVarResponse = deleteByParameterKeyIdWithHttpInfo(keyId = keyId)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Delete parameters by parameter key identifier
* Deletes parameter and all it's values by parameter key identifier
* @param keyId Identifier of the parameter key
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun deleteByParameterKeyIdWithHttpInfo(keyId: java.util.UUID) : ApiResponse {
val localVariableConfig = deleteByParameterKeyIdRequestConfig(keyId = keyId)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation deleteByParameterKeyId
*
* @param keyId Identifier of the parameter key
* @return RequestConfig
*/
fun deleteByParameterKeyIdRequestConfig(keyId: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.DELETE,
path = "/api/v2/parameters/keyId/{keyId}".replace("{"+"keyId"+"}", encodeURIComponent(keyId.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Delete parameter
* Use case User sets parameter internal (guid format) identifier System search and delete parameter System returns deleted parameter
* @param id Parameter internal (UUID) identifier
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun deleteParameter(id: java.util.UUID) : Unit {
val localVarResponse = deleteParameterWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Delete parameter
* Use case User sets parameter internal (guid format) identifier System search and delete parameter System returns deleted parameter
* @param id Parameter internal (UUID) identifier
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun deleteParameterWithHttpInfo(id: java.util.UUID) : ApiResponse {
val localVariableConfig = deleteParameterRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation deleteParameter
*
* @param id Parameter internal (UUID) identifier
* @return RequestConfig
*/
fun deleteParameterRequestConfig(id: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.DELETE,
path = "/api/v2/parameters/{id}".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get all parameters
* Use case [Optional] User sets isDeleted field value [Optional] If User sets isDeleted field value as true, System search all deleted parameters [Optional] If User sets isDeleted field value as false, System search all parameters which are not deleted If User did not set isDeleted field value, System search all parameters System returns array of all found parameters(listed in response model)
* @param isDeleted If result must consist of only actual/deleted parameters (optional)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun getAllParameters(isDeleted: kotlin.Boolean? = null, skip: kotlin.Int? = null, take: kotlin.Int? = null, orderBy: kotlin.String? = null, searchField: kotlin.String? = null, searchValue: kotlin.String? = null) : kotlin.collections.List {
val localVarResponse = getAllParametersWithHttpInfo(isDeleted = isDeleted, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get all parameters
* Use case [Optional] User sets isDeleted field value [Optional] If User sets isDeleted field value as true, System search all deleted parameters [Optional] If User sets isDeleted field value as false, System search all parameters which are not deleted If User did not set isDeleted field value, System search all parameters System returns array of all found parameters(listed in response model)
* @param isDeleted If result must consist of only actual/deleted parameters (optional)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun getAllParametersWithHttpInfo(isDeleted: kotlin.Boolean?, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?) : ApiResponse?> {
val localVariableConfig = getAllParametersRequestConfig(isDeleted = isDeleted, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getAllParameters
*
* @param isDeleted If result must consist of only actual/deleted parameters (optional)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return RequestConfig
*/
fun getAllParametersRequestConfig(isDeleted: kotlin.Boolean?, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (isDeleted != null) {
put("isDeleted", listOf(isDeleted.toString()))
}
if (skip != null) {
put("Skip", listOf(skip.toString()))
}
if (take != null) {
put("Take", listOf(take.toString()))
}
if (orderBy != null) {
put("OrderBy", listOf(orderBy.toString()))
}
if (searchField != null) {
put("SearchField", listOf(searchField.toString()))
}
if (searchValue != null) {
put("SearchValue", listOf(searchValue.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/parameters",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get parameter by ID
* Use case User sets parameter internal (guid format) identifier User runs method execution System search parameter using the identifier System returns parameter
* @param id Parameter internal (UUID) identifier
* @return ParameterModel
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun getParameterById(id: java.util.UUID) : ParameterModel {
val localVarResponse = getParameterByIdWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as ParameterModel
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get parameter by ID
* Use case User sets parameter internal (guid format) identifier User runs method execution System search parameter using the identifier System returns parameter
* @param id Parameter internal (UUID) identifier
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun getParameterByIdWithHttpInfo(id: java.util.UUID) : ApiResponse {
val localVariableConfig = getParameterByIdRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getParameterById
*
* @param id Parameter internal (UUID) identifier
* @return RequestConfig
*/
fun getParameterByIdRequestConfig(id: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/parameters/{id}".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Update parameter
* Use case User sets parameter updated properties(listed in the request example) User runs method execution System updated parameter using updated properties System returns no content response
* @param parameterPutModel (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun updateParameter(parameterPutModel: ParameterPutModel? = null) : Unit {
val localVarResponse = updateParameterWithHttpInfo(parameterPutModel = parameterPutModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Update parameter
* Use case User sets parameter updated properties(listed in the request example) User runs method execution System updated parameter using updated properties System returns no content response
* @param parameterPutModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun updateParameterWithHttpInfo(parameterPutModel: ParameterPutModel?) : ApiResponse {
val localVariableConfig = updateParameterRequestConfig(parameterPutModel = parameterPutModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation updateParameter
*
* @param parameterPutModel (optional)
* @return RequestConfig
*/
fun updateParameterRequestConfig(parameterPutModel: ParameterPutModel?) : RequestConfig {
val localVariableBody = parameterPutModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.PUT,
path = "/api/v2/parameters",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
private fun encodeURIComponent(uriComponent: kotlin.String): kotlin.String =
HttpUrl.Builder().scheme("http").host("localhost").addPathSegment(uriComponent).build().encodedPathSegments[0]
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy