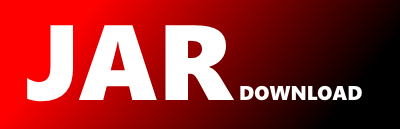
ru.testit.kotlin.client.apis.ProjectTestPlanAttributesApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of testit-api-client-kotlin Show documentation
Show all versions of testit-api-client-kotlin Show documentation
Kotlin API client for TestIT.
/**
*
* Please note:
* This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit this file manually.
*
*/
@file:Suppress(
"ArrayInDataClass",
"EnumEntryName",
"RemoveRedundantQualifierName",
"UnusedImport"
)
package ru.testit.kotlin.client.apis
import java.io.IOException
import okhttp3.OkHttpClient
import okhttp3.HttpUrl
import ru.testit.kotlin.client.models.CustomAttributeGetModel
import ru.testit.kotlin.client.models.CustomAttributeModel
import ru.testit.kotlin.client.models.CustomAttributeTestPlanProjectRelationPutModel
import ru.testit.kotlin.client.models.ProblemDetails
import ru.testit.kotlin.client.models.ProjectAttributesFilterModel
import ru.testit.kotlin.client.models.ValidationProblemDetails
import com.squareup.moshi.Json
import ru.testit.kotlin.client.infrastructure.ApiClient
import ru.testit.kotlin.client.infrastructure.ApiResponse
import ru.testit.kotlin.client.infrastructure.ClientException
import ru.testit.kotlin.client.infrastructure.ClientError
import ru.testit.kotlin.client.infrastructure.ServerException
import ru.testit.kotlin.client.infrastructure.ServerError
import ru.testit.kotlin.client.infrastructure.MultiValueMap
import ru.testit.kotlin.client.infrastructure.PartConfig
import ru.testit.kotlin.client.infrastructure.RequestConfig
import ru.testit.kotlin.client.infrastructure.RequestMethod
import ru.testit.kotlin.client.infrastructure.ResponseType
import ru.testit.kotlin.client.infrastructure.Success
import ru.testit.kotlin.client.infrastructure.toMultiValue
class ProjectTestPlanAttributesApi(basePath: kotlin.String = defaultBasePath, client: OkHttpClient = ApiClient.defaultClient) : ApiClient(basePath, client) {
companion object {
@JvmStatic
val defaultBasePath: String by lazy {
System.getProperties().getProperty(ApiClient.baseUrlKey, "http://localhost")
}
}
/**
* Add attributes to project's test plans
* Use case User sets project internal or global identifier and attributes identifiers User runs method execution System updates project and add attributes to project for test plans System returns no content response
* @param projectId Project internal (UUID) or global (integer) identifier
* @param javaUtilUUID (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun createCustomAttributeTestPlanProjectRelations(projectId: kotlin.String, javaUtilUUID: kotlin.collections.Set? = null) : Unit {
val localVarResponse = createCustomAttributeTestPlanProjectRelationsWithHttpInfo(projectId = projectId, javaUtilUUID = javaUtilUUID)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Add attributes to project's test plans
* Use case User sets project internal or global identifier and attributes identifiers User runs method execution System updates project and add attributes to project for test plans System returns no content response
* @param projectId Project internal (UUID) or global (integer) identifier
* @param javaUtilUUID (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun createCustomAttributeTestPlanProjectRelationsWithHttpInfo(projectId: kotlin.String, javaUtilUUID: kotlin.collections.Set?) : ApiResponse {
val localVariableConfig = createCustomAttributeTestPlanProjectRelationsRequestConfig(projectId = projectId, javaUtilUUID = javaUtilUUID)
return request, Unit>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation createCustomAttributeTestPlanProjectRelations
*
* @param projectId Project internal (UUID) or global (integer) identifier
* @param javaUtilUUID (optional)
* @return RequestConfig
*/
fun createCustomAttributeTestPlanProjectRelationsRequestConfig(projectId: kotlin.String, javaUtilUUID: kotlin.collections.Set?) : RequestConfig> {
val localVariableBody = javaUtilUUID
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/projects/{projectId}/testPlans/attributes".replace("{"+"projectId"+"}", encodeURIComponent(projectId.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Delete attribute from project's test plans
* Use case User sets project internal or global identifier and attribute identifier User runs method execution System updates project and delete attribute from project for test plans System returns no content response
* @param projectId Project internal (UUID) or global (integer) identifier
* @param attributeId
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun deleteCustomAttributeTestPlanProjectRelations(projectId: kotlin.String, attributeId: java.util.UUID) : Unit {
val localVarResponse = deleteCustomAttributeTestPlanProjectRelationsWithHttpInfo(projectId = projectId, attributeId = attributeId)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Delete attribute from project's test plans
* Use case User sets project internal or global identifier and attribute identifier User runs method execution System updates project and delete attribute from project for test plans System returns no content response
* @param projectId Project internal (UUID) or global (integer) identifier
* @param attributeId
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun deleteCustomAttributeTestPlanProjectRelationsWithHttpInfo(projectId: kotlin.String, attributeId: java.util.UUID) : ApiResponse {
val localVariableConfig = deleteCustomAttributeTestPlanProjectRelationsRequestConfig(projectId = projectId, attributeId = attributeId)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation deleteCustomAttributeTestPlanProjectRelations
*
* @param projectId Project internal (UUID) or global (integer) identifier
* @param attributeId
* @return RequestConfig
*/
fun deleteCustomAttributeTestPlanProjectRelationsRequestConfig(projectId: kotlin.String, attributeId: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.DELETE,
path = "/api/v2/projects/{projectId}/testPlans/attributes/{attributeId}".replace("{"+"projectId"+"}", encodeURIComponent(projectId.toString())).replace("{"+"attributeId"+"}", encodeURIComponent(attributeId.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get project's test plan attributes
* Use case User runs method execution System returns project for test plans attributes by project identifier
* @param projectId Project internal (UUID) or global (integer) identifier
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun getCustomAttributeTestPlanProjectRelations(projectId: kotlin.String) : kotlin.collections.List {
val localVarResponse = getCustomAttributeTestPlanProjectRelationsWithHttpInfo(projectId = projectId)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get project's test plan attributes
* Use case User runs method execution System returns project for test plans attributes by project identifier
* @param projectId Project internal (UUID) or global (integer) identifier
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun getCustomAttributeTestPlanProjectRelationsWithHttpInfo(projectId: kotlin.String) : ApiResponse?> {
val localVariableConfig = getCustomAttributeTestPlanProjectRelationsRequestConfig(projectId = projectId)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getCustomAttributeTestPlanProjectRelations
*
* @param projectId Project internal (UUID) or global (integer) identifier
* @return RequestConfig
*/
fun getCustomAttributeTestPlanProjectRelationsRequestConfig(projectId: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/projects/{projectId}/testPlans/attributes".replace("{"+"projectId"+"}", encodeURIComponent(projectId.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Search for attributes used in the project test plans
*
* @param projectId Unique or global project ID
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param projectAttributesFilterModel (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun searchTestPlanAttributesInProject(projectId: kotlin.String, skip: kotlin.Int? = null, take: kotlin.Int? = null, orderBy: kotlin.String? = null, searchField: kotlin.String? = null, searchValue: kotlin.String? = null, projectAttributesFilterModel: ProjectAttributesFilterModel? = null) : kotlin.collections.List {
val localVarResponse = searchTestPlanAttributesInProjectWithHttpInfo(projectId = projectId, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue, projectAttributesFilterModel = projectAttributesFilterModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Search for attributes used in the project test plans
*
* @param projectId Unique or global project ID
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param projectAttributesFilterModel (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun searchTestPlanAttributesInProjectWithHttpInfo(projectId: kotlin.String, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?, projectAttributesFilterModel: ProjectAttributesFilterModel?) : ApiResponse?> {
val localVariableConfig = searchTestPlanAttributesInProjectRequestConfig(projectId = projectId, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue, projectAttributesFilterModel = projectAttributesFilterModel)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation searchTestPlanAttributesInProject
*
* @param projectId Unique or global project ID
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param projectAttributesFilterModel (optional)
* @return RequestConfig
*/
fun searchTestPlanAttributesInProjectRequestConfig(projectId: kotlin.String, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?, projectAttributesFilterModel: ProjectAttributesFilterModel?) : RequestConfig {
val localVariableBody = projectAttributesFilterModel
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (skip != null) {
put("Skip", listOf(skip.toString()))
}
if (take != null) {
put("Take", listOf(take.toString()))
}
if (orderBy != null) {
put("OrderBy", listOf(orderBy.toString()))
}
if (searchField != null) {
put("SearchField", listOf(searchField.toString()))
}
if (searchValue != null) {
put("SearchValue", listOf(searchValue.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/projects/{projectId}/testPlans/attributes/search".replace("{"+"projectId"+"}", encodeURIComponent(projectId.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Update attribute of project's test plans
* Use case User sets project internal or global identifier and attribute model User runs method execution System updates project and project attribute for test plan System returns no content response
* @param projectId Project internal (UUID) or global (integer) identifier
* @param customAttributeTestPlanProjectRelationPutModel (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun updateCustomAttributeTestPlanProjectRelations(projectId: kotlin.String, customAttributeTestPlanProjectRelationPutModel: CustomAttributeTestPlanProjectRelationPutModel? = null) : Unit {
val localVarResponse = updateCustomAttributeTestPlanProjectRelationsWithHttpInfo(projectId = projectId, customAttributeTestPlanProjectRelationPutModel = customAttributeTestPlanProjectRelationPutModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Update attribute of project's test plans
* Use case User sets project internal or global identifier and attribute model User runs method execution System updates project and project attribute for test plan System returns no content response
* @param projectId Project internal (UUID) or global (integer) identifier
* @param customAttributeTestPlanProjectRelationPutModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun updateCustomAttributeTestPlanProjectRelationsWithHttpInfo(projectId: kotlin.String, customAttributeTestPlanProjectRelationPutModel: CustomAttributeTestPlanProjectRelationPutModel?) : ApiResponse {
val localVariableConfig = updateCustomAttributeTestPlanProjectRelationsRequestConfig(projectId = projectId, customAttributeTestPlanProjectRelationPutModel = customAttributeTestPlanProjectRelationPutModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation updateCustomAttributeTestPlanProjectRelations
*
* @param projectId Project internal (UUID) or global (integer) identifier
* @param customAttributeTestPlanProjectRelationPutModel (optional)
* @return RequestConfig
*/
fun updateCustomAttributeTestPlanProjectRelationsRequestConfig(projectId: kotlin.String, customAttributeTestPlanProjectRelationPutModel: CustomAttributeTestPlanProjectRelationPutModel?) : RequestConfig {
val localVariableBody = customAttributeTestPlanProjectRelationPutModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.PUT,
path = "/api/v2/projects/{projectId}/testPlans/attributes".replace("{"+"projectId"+"}", encodeURIComponent(projectId.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
private fun encodeURIComponent(uriComponent: kotlin.String): kotlin.String =
HttpUrl.Builder().scheme("http").host("localhost").addPathSegment(uriComponent).build().encodedPathSegments[0]
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy