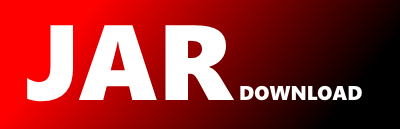
ru.testit.kotlin.client.apis.ProjectsApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of testit-api-client-kotlin Show documentation
Show all versions of testit-api-client-kotlin Show documentation
Kotlin API client for TestIT.
/**
*
* Please note:
* This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit this file manually.
*
*/
@file:Suppress(
"ArrayInDataClass",
"EnumEntryName",
"RemoveRedundantQualifierName",
"UnusedImport"
)
package ru.testit.kotlin.client.apis
import java.io.IOException
import okhttp3.OkHttpClient
import okhttp3.HttpUrl
import ru.testit.kotlin.client.models.AutoTestNamespaceModel
import ru.testit.kotlin.client.models.CustomAttributeTestPlanProjectRelationPutModel
import ru.testit.kotlin.client.models.FailureClassModel
import ru.testit.kotlin.client.models.FilterModel
import ru.testit.kotlin.client.models.Operation
import ru.testit.kotlin.client.models.ProblemDetails
import ru.testit.kotlin.client.models.ProjectModel
import ru.testit.kotlin.client.models.ProjectPostModel
import ru.testit.kotlin.client.models.ProjectPutModel
import ru.testit.kotlin.client.models.ProjectSelectModel
import ru.testit.kotlin.client.models.ProjectsFilterModel
import ru.testit.kotlin.client.models.PublicTestRunModel
import ru.testit.kotlin.client.models.TestPlanModel
import ru.testit.kotlin.client.models.TestRunModel
import ru.testit.kotlin.client.models.TestRunV2GetModel
import ru.testit.kotlin.client.models.ValidationProblemDetails
import com.squareup.moshi.Json
import ru.testit.kotlin.client.infrastructure.ApiClient
import ru.testit.kotlin.client.infrastructure.ApiResponse
import ru.testit.kotlin.client.infrastructure.ClientException
import ru.testit.kotlin.client.infrastructure.ClientError
import ru.testit.kotlin.client.infrastructure.ServerException
import ru.testit.kotlin.client.infrastructure.ServerError
import ru.testit.kotlin.client.infrastructure.MultiValueMap
import ru.testit.kotlin.client.infrastructure.PartConfig
import ru.testit.kotlin.client.infrastructure.RequestConfig
import ru.testit.kotlin.client.infrastructure.RequestMethod
import ru.testit.kotlin.client.infrastructure.ResponseType
import ru.testit.kotlin.client.infrastructure.Success
import ru.testit.kotlin.client.infrastructure.toMultiValue
class ProjectsApi(basePath: kotlin.String = defaultBasePath, client: OkHttpClient = ApiClient.defaultClient) : ApiClient(basePath, client) {
companion object {
@JvmStatic
val defaultBasePath: String by lazy {
System.getProperties().getProperty(ApiClient.baseUrlKey, "http://localhost")
}
}
/**
* Add global attributes to project
* Use case User sets project internal or global identifier and attributes identifiers System search project System relates global attributes with project System returns no content response
* @param id Project internal (UUID) or global (integer) identifier
* @param javaUtilUUID (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun addGlobaAttributesToProject(id: kotlin.String, javaUtilUUID: kotlin.collections.Set? = null) : Unit {
val localVarResponse = addGlobaAttributesToProjectWithHttpInfo(id = id, javaUtilUUID = javaUtilUUID)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Add global attributes to project
* Use case User sets project internal or global identifier and attributes identifiers System search project System relates global attributes with project System returns no content response
* @param id Project internal (UUID) or global (integer) identifier
* @param javaUtilUUID (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun addGlobaAttributesToProjectWithHttpInfo(id: kotlin.String, javaUtilUUID: kotlin.collections.Set?) : ApiResponse {
val localVariableConfig = addGlobaAttributesToProjectRequestConfig(id = id, javaUtilUUID = javaUtilUUID)
return request, Unit>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation addGlobaAttributesToProject
*
* @param id Project internal (UUID) or global (integer) identifier
* @param javaUtilUUID (optional)
* @return RequestConfig
*/
fun addGlobaAttributesToProjectRequestConfig(id: kotlin.String, javaUtilUUID: kotlin.collections.Set?) : RequestConfig> {
val localVariableBody = javaUtilUUID
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/projects/{id}/globalAttributes".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
*
*
* @param projectPostModel (optional)
* @return ProjectModel
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2ProjectsDemoPost(projectPostModel: ProjectPostModel? = null) : ProjectModel {
val localVarResponse = apiV2ProjectsDemoPostWithHttpInfo(projectPostModel = projectPostModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as ProjectModel
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
*
*
* @param projectPostModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2ProjectsDemoPostWithHttpInfo(projectPostModel: ProjectPostModel?) : ApiResponse {
val localVariableConfig = apiV2ProjectsDemoPostRequestConfig(projectPostModel = projectPostModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ProjectsDemoPost
*
* @param projectPostModel (optional)
* @return RequestConfig
*/
fun apiV2ProjectsDemoPostRequestConfig(projectPostModel: ProjectPostModel?) : RequestConfig {
val localVariableBody = projectPostModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/projects/demo",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Archive project
*
* @param id Unique or global ID of the project
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2ProjectsIdDelete(id: kotlin.String) : Unit {
val localVarResponse = apiV2ProjectsIdDeleteWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Archive project
*
* @param id Unique or global ID of the project
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2ProjectsIdDeleteWithHttpInfo(id: kotlin.String) : ApiResponse {
val localVariableConfig = apiV2ProjectsIdDeleteRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ProjectsIdDelete
*
* @param id Unique or global ID of the project
* @return RequestConfig
*/
fun apiV2ProjectsIdDeleteRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.DELETE,
path = "/api/v2/projects/{id}".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get failure classes
*
* @param id Unique or global ID of the project
* @param isDeleted (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2ProjectsIdFailureClassesGet(id: kotlin.String, isDeleted: kotlin.Boolean? = null) : kotlin.collections.List {
val localVarResponse = apiV2ProjectsIdFailureClassesGetWithHttpInfo(id = id, isDeleted = isDeleted)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get failure classes
*
* @param id Unique or global ID of the project
* @param isDeleted (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2ProjectsIdFailureClassesGetWithHttpInfo(id: kotlin.String, isDeleted: kotlin.Boolean?) : ApiResponse?> {
val localVariableConfig = apiV2ProjectsIdFailureClassesGetRequestConfig(id = id, isDeleted = isDeleted)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ProjectsIdFailureClassesGet
*
* @param id Unique or global ID of the project
* @param isDeleted (optional)
* @return RequestConfig
*/
fun apiV2ProjectsIdFailureClassesGetRequestConfig(id: kotlin.String, isDeleted: kotlin.Boolean?) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (isDeleted != null) {
put("isDeleted", listOf(isDeleted.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/projects/{id}/failureClasses".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Mark Project as favorite
*
* @param id Project internal (UUID) or global (integer) identifier
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2ProjectsIdFavoritePut(id: kotlin.String) : Unit {
val localVarResponse = apiV2ProjectsIdFavoritePutWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Mark Project as favorite
*
* @param id Project internal (UUID) or global (integer) identifier
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2ProjectsIdFavoritePutWithHttpInfo(id: kotlin.String) : ApiResponse {
val localVariableConfig = apiV2ProjectsIdFavoritePutRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ProjectsIdFavoritePut
*
* @param id Project internal (UUID) or global (integer) identifier
* @return RequestConfig
*/
fun apiV2ProjectsIdFavoritePutRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.PUT,
path = "/api/v2/projects/{id}/favorite".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get Project filters
* Use case User sets project internal or global identifier User runs method execution System returns project filters
* @param id Project internal (UUID) or global (integer) identifier
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2ProjectsIdFiltersGet(id: kotlin.String) : kotlin.collections.List {
val localVarResponse = apiV2ProjectsIdFiltersGetWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get Project filters
* Use case User sets project internal or global identifier User runs method execution System returns project filters
* @param id Project internal (UUID) or global (integer) identifier
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2ProjectsIdFiltersGetWithHttpInfo(id: kotlin.String) : ApiResponse?> {
val localVariableConfig = apiV2ProjectsIdFiltersGetRequestConfig(id = id)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ProjectsIdFiltersGet
*
* @param id Project internal (UUID) or global (integer) identifier
* @return RequestConfig
*/
fun apiV2ProjectsIdFiltersGetRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/projects/{id}/filters".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Patch project
* See <a href=\"https://www.rfc-editor.org/rfc/rfc6902\" target=\"_blank\">RFC 6902: JavaScript Object Notation (JSON) Patch</a> for details
* @param id Unique or global Id of project
* @param operation (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2ProjectsIdPatch(id: java.util.UUID, operation: kotlin.collections.List? = null) : Unit {
val localVarResponse = apiV2ProjectsIdPatchWithHttpInfo(id = id, operation = operation)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Patch project
* See <a href=\"https://www.rfc-editor.org/rfc/rfc6902\" target=\"_blank\">RFC 6902: JavaScript Object Notation (JSON) Patch</a> for details
* @param id Unique or global Id of project
* @param operation (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2ProjectsIdPatchWithHttpInfo(id: java.util.UUID, operation: kotlin.collections.List?) : ApiResponse {
val localVariableConfig = apiV2ProjectsIdPatchRequestConfig(id = id, operation = operation)
return request, Unit>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ProjectsIdPatch
*
* @param id Unique or global Id of project
* @param operation (optional)
* @return RequestConfig
*/
fun apiV2ProjectsIdPatchRequestConfig(id: java.util.UUID, operation: kotlin.collections.List?) : RequestConfig> {
val localVariableBody = operation
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.PATCH,
path = "/api/v2/projects/{id}".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Purge the project
*
* @param id Unique or global ID of the project
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2ProjectsIdPurgePost(id: kotlin.String) : Unit {
val localVarResponse = apiV2ProjectsIdPurgePostWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Purge the project
*
* @param id Unique or global ID of the project
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2ProjectsIdPurgePostWithHttpInfo(id: kotlin.String) : ApiResponse {
val localVariableConfig = apiV2ProjectsIdPurgePostRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ProjectsIdPurgePost
*
* @param id Unique or global ID of the project
* @return RequestConfig
*/
fun apiV2ProjectsIdPurgePostRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/projects/{id}/purge".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Restore archived project
*
* @param id Unique or global ID of the project
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2ProjectsIdRestorePost(id: kotlin.String) : Unit {
val localVarResponse = apiV2ProjectsIdRestorePostWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Restore archived project
*
* @param id Unique or global ID of the project
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2ProjectsIdRestorePostWithHttpInfo(id: kotlin.String) : ApiResponse {
val localVariableConfig = apiV2ProjectsIdRestorePostRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ProjectsIdRestorePost
*
* @param id Unique or global ID of the project
* @return RequestConfig
*/
fun apiV2ProjectsIdRestorePostRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/projects/{id}/restore".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Delete attribute from project's test plans
* Use case User sets project internal or global identifier and attribute identifier User runs method execution System updates project and delete attribute from project for test plans System returns no content response
* @param id Project internal (UUID) or global (integer) identifier
* @param attributeId
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
@Deprecated(message = "This operation is deprecated.")
fun apiV2ProjectsIdTestPlansAttributeAttributeIdDelete(id: kotlin.String, attributeId: java.util.UUID) : Unit {
@Suppress("DEPRECATION")
val localVarResponse = apiV2ProjectsIdTestPlansAttributeAttributeIdDeleteWithHttpInfo(id = id, attributeId = attributeId)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Delete attribute from project's test plans
* Use case User sets project internal or global identifier and attribute identifier User runs method execution System updates project and delete attribute from project for test plans System returns no content response
* @param id Project internal (UUID) or global (integer) identifier
* @param attributeId
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
@Deprecated(message = "This operation is deprecated.")
fun apiV2ProjectsIdTestPlansAttributeAttributeIdDeleteWithHttpInfo(id: kotlin.String, attributeId: java.util.UUID) : ApiResponse {
@Suppress("DEPRECATION")
val localVariableConfig = apiV2ProjectsIdTestPlansAttributeAttributeIdDeleteRequestConfig(id = id, attributeId = attributeId)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ProjectsIdTestPlansAttributeAttributeIdDelete
*
* @param id Project internal (UUID) or global (integer) identifier
* @param attributeId
* @return RequestConfig
*/
@Deprecated(message = "This operation is deprecated.")
fun apiV2ProjectsIdTestPlansAttributeAttributeIdDeleteRequestConfig(id: kotlin.String, attributeId: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.DELETE,
path = "/api/v2/projects/{id}/testPlans/attribute/{attributeId}".replace("{"+"id"+"}", encodeURIComponent(id.toString())).replace("{"+"attributeId"+"}", encodeURIComponent(attributeId.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Update attribute of project's test plans
* Use case User sets project internal or global identifier and attribute model User runs method execution System updates project and project attribute for test plan System returns no content response
* @param id Project internal (UUID) or global (integer) identifier
* @param customAttributeTestPlanProjectRelationPutModel (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
@Deprecated(message = "This operation is deprecated.")
fun apiV2ProjectsIdTestPlansAttributePut(id: kotlin.String, customAttributeTestPlanProjectRelationPutModel: CustomAttributeTestPlanProjectRelationPutModel? = null) : Unit {
@Suppress("DEPRECATION")
val localVarResponse = apiV2ProjectsIdTestPlansAttributePutWithHttpInfo(id = id, customAttributeTestPlanProjectRelationPutModel = customAttributeTestPlanProjectRelationPutModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Update attribute of project's test plans
* Use case User sets project internal or global identifier and attribute model User runs method execution System updates project and project attribute for test plan System returns no content response
* @param id Project internal (UUID) or global (integer) identifier
* @param customAttributeTestPlanProjectRelationPutModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
@Deprecated(message = "This operation is deprecated.")
fun apiV2ProjectsIdTestPlansAttributePutWithHttpInfo(id: kotlin.String, customAttributeTestPlanProjectRelationPutModel: CustomAttributeTestPlanProjectRelationPutModel?) : ApiResponse {
@Suppress("DEPRECATION")
val localVariableConfig = apiV2ProjectsIdTestPlansAttributePutRequestConfig(id = id, customAttributeTestPlanProjectRelationPutModel = customAttributeTestPlanProjectRelationPutModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ProjectsIdTestPlansAttributePut
*
* @param id Project internal (UUID) or global (integer) identifier
* @param customAttributeTestPlanProjectRelationPutModel (optional)
* @return RequestConfig
*/
@Deprecated(message = "This operation is deprecated.")
fun apiV2ProjectsIdTestPlansAttributePutRequestConfig(id: kotlin.String, customAttributeTestPlanProjectRelationPutModel: CustomAttributeTestPlanProjectRelationPutModel?) : RequestConfig {
val localVariableBody = customAttributeTestPlanProjectRelationPutModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.PUT,
path = "/api/v2/projects/{id}/testPlans/attribute".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get active Project TestRuns
* Use case User sets project internal or global identifier User runs method execution System returns active testruns
* @param id Project internal (UUID) or global (integer) identifier
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2ProjectsIdTestRunsActiveGet(id: kotlin.String) : kotlin.collections.List {
val localVarResponse = apiV2ProjectsIdTestRunsActiveGetWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get active Project TestRuns
* Use case User sets project internal or global identifier User runs method execution System returns active testruns
* @param id Project internal (UUID) or global (integer) identifier
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2ProjectsIdTestRunsActiveGetWithHttpInfo(id: kotlin.String) : ApiResponse?> {
val localVariableConfig = apiV2ProjectsIdTestRunsActiveGetRequestConfig(id = id)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ProjectsIdTestRunsActiveGet
*
* @param id Project internal (UUID) or global (integer) identifier
* @return RequestConfig
*/
fun apiV2ProjectsIdTestRunsActiveGetRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/projects/{id}/testRuns/active".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get Project TestRuns full models
* Use case User sets project internal or global identifier User sets query params User runs method execution System returns project test runs full models
* @param id Project internal (UUID) or global (integer) identifier
* @param includeTestResults (optional, default to false)
* @param mustAggregateTestResults (optional, default to true)
* @param notStarted (optional)
* @param inProgress (optional)
* @param stopped (optional)
* @param completed (optional)
* @param createdDateFrom (optional)
* @param createdDateTo (optional)
* @param testPlanId (optional)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2ProjectsIdTestRunsFullGet(id: kotlin.String, includeTestResults: kotlin.Boolean? = false, mustAggregateTestResults: kotlin.Boolean? = true, notStarted: kotlin.Boolean? = null, inProgress: kotlin.Boolean? = null, stopped: kotlin.Boolean? = null, completed: kotlin.Boolean? = null, createdDateFrom: java.time.OffsetDateTime? = null, createdDateTo: java.time.OffsetDateTime? = null, testPlanId: java.util.UUID? = null, skip: kotlin.Int? = null, take: kotlin.Int? = null, orderBy: kotlin.String? = null, searchField: kotlin.String? = null, searchValue: kotlin.String? = null) : kotlin.collections.List {
val localVarResponse = apiV2ProjectsIdTestRunsFullGetWithHttpInfo(id = id, includeTestResults = includeTestResults, mustAggregateTestResults = mustAggregateTestResults, notStarted = notStarted, inProgress = inProgress, stopped = stopped, completed = completed, createdDateFrom = createdDateFrom, createdDateTo = createdDateTo, testPlanId = testPlanId, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get Project TestRuns full models
* Use case User sets project internal or global identifier User sets query params User runs method execution System returns project test runs full models
* @param id Project internal (UUID) or global (integer) identifier
* @param includeTestResults (optional, default to false)
* @param mustAggregateTestResults (optional, default to true)
* @param notStarted (optional)
* @param inProgress (optional)
* @param stopped (optional)
* @param completed (optional)
* @param createdDateFrom (optional)
* @param createdDateTo (optional)
* @param testPlanId (optional)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2ProjectsIdTestRunsFullGetWithHttpInfo(id: kotlin.String, includeTestResults: kotlin.Boolean?, mustAggregateTestResults: kotlin.Boolean?, notStarted: kotlin.Boolean?, inProgress: kotlin.Boolean?, stopped: kotlin.Boolean?, completed: kotlin.Boolean?, createdDateFrom: java.time.OffsetDateTime?, createdDateTo: java.time.OffsetDateTime?, testPlanId: java.util.UUID?, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?) : ApiResponse?> {
val localVariableConfig = apiV2ProjectsIdTestRunsFullGetRequestConfig(id = id, includeTestResults = includeTestResults, mustAggregateTestResults = mustAggregateTestResults, notStarted = notStarted, inProgress = inProgress, stopped = stopped, completed = completed, createdDateFrom = createdDateFrom, createdDateTo = createdDateTo, testPlanId = testPlanId, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ProjectsIdTestRunsFullGet
*
* @param id Project internal (UUID) or global (integer) identifier
* @param includeTestResults (optional, default to false)
* @param mustAggregateTestResults (optional, default to true)
* @param notStarted (optional)
* @param inProgress (optional)
* @param stopped (optional)
* @param completed (optional)
* @param createdDateFrom (optional)
* @param createdDateTo (optional)
* @param testPlanId (optional)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return RequestConfig
*/
fun apiV2ProjectsIdTestRunsFullGetRequestConfig(id: kotlin.String, includeTestResults: kotlin.Boolean?, mustAggregateTestResults: kotlin.Boolean?, notStarted: kotlin.Boolean?, inProgress: kotlin.Boolean?, stopped: kotlin.Boolean?, completed: kotlin.Boolean?, createdDateFrom: java.time.OffsetDateTime?, createdDateTo: java.time.OffsetDateTime?, testPlanId: java.util.UUID?, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (includeTestResults != null) {
put("includeTestResults", listOf(includeTestResults.toString()))
}
if (mustAggregateTestResults != null) {
put("mustAggregateTestResults", listOf(mustAggregateTestResults.toString()))
}
if (notStarted != null) {
put("notStarted", listOf(notStarted.toString()))
}
if (inProgress != null) {
put("inProgress", listOf(inProgress.toString()))
}
if (stopped != null) {
put("stopped", listOf(stopped.toString()))
}
if (completed != null) {
put("completed", listOf(completed.toString()))
}
if (createdDateFrom != null) {
put("createdDateFrom", listOf(parseDateToQueryString(createdDateFrom)))
}
if (createdDateTo != null) {
put("createdDateTo", listOf(parseDateToQueryString(createdDateTo)))
}
if (testPlanId != null) {
put("testPlanId", listOf(testPlanId.toString()))
}
if (skip != null) {
put("Skip", listOf(skip.toString()))
}
if (take != null) {
put("Take", listOf(take.toString()))
}
if (orderBy != null) {
put("OrderBy", listOf(orderBy.toString()))
}
if (searchField != null) {
put("SearchField", listOf(searchField.toString()))
}
if (searchValue != null) {
put("SearchValue", listOf(searchValue.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/projects/{id}/testRuns/full".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
*
*
* @param name
* @return kotlin.Boolean
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2ProjectsNameNameExistsGet(name: kotlin.String) : kotlin.Boolean {
val localVarResponse = apiV2ProjectsNameNameExistsGetWithHttpInfo(name = name)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.Boolean
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
*
*
* @param name
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2ProjectsNameNameExistsGetWithHttpInfo(name: kotlin.String) : ApiResponse {
val localVariableConfig = apiV2ProjectsNameNameExistsGetRequestConfig(name = name)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ProjectsNameNameExistsGet
*
* @param name
* @return RequestConfig
*/
fun apiV2ProjectsNameNameExistsGetRequestConfig(name: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/projects/name/{name}/exists".replace("{"+"name"+"}", encodeURIComponent(name.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Purge multiple projects
*
* @param projectSelectModel (optional)
* @return kotlin.Long
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2ProjectsPurgeBulkPost(projectSelectModel: ProjectSelectModel? = null) : kotlin.Long {
val localVarResponse = apiV2ProjectsPurgeBulkPostWithHttpInfo(projectSelectModel = projectSelectModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.Long
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Purge multiple projects
*
* @param projectSelectModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2ProjectsPurgeBulkPostWithHttpInfo(projectSelectModel: ProjectSelectModel?) : ApiResponse {
val localVariableConfig = apiV2ProjectsPurgeBulkPostRequestConfig(projectSelectModel = projectSelectModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ProjectsPurgeBulkPost
*
* @param projectSelectModel (optional)
* @return RequestConfig
*/
fun apiV2ProjectsPurgeBulkPostRequestConfig(projectSelectModel: ProjectSelectModel?) : RequestConfig {
val localVariableBody = projectSelectModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/projects/purge/bulk",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Restore multiple projects
*
* @param projectSelectModel (optional)
* @return kotlin.Long
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2ProjectsRestoreBulkPost(projectSelectModel: ProjectSelectModel? = null) : kotlin.Long {
val localVarResponse = apiV2ProjectsRestoreBulkPostWithHttpInfo(projectSelectModel = projectSelectModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.Long
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Restore multiple projects
*
* @param projectSelectModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2ProjectsRestoreBulkPostWithHttpInfo(projectSelectModel: ProjectSelectModel?) : ApiResponse {
val localVariableConfig = apiV2ProjectsRestoreBulkPostRequestConfig(projectSelectModel = projectSelectModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ProjectsRestoreBulkPost
*
* @param projectSelectModel (optional)
* @return RequestConfig
*/
fun apiV2ProjectsRestoreBulkPostRequestConfig(projectSelectModel: ProjectSelectModel?) : RequestConfig {
val localVariableBody = projectSelectModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/projects/restore/bulk",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Search for projects
*
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param projectsFilterModel (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2ProjectsSearchPost(skip: kotlin.Int? = null, take: kotlin.Int? = null, orderBy: kotlin.String? = null, searchField: kotlin.String? = null, searchValue: kotlin.String? = null, projectsFilterModel: ProjectsFilterModel? = null) : kotlin.collections.List {
val localVarResponse = apiV2ProjectsSearchPostWithHttpInfo(skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue, projectsFilterModel = projectsFilterModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Search for projects
*
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param projectsFilterModel (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2ProjectsSearchPostWithHttpInfo(skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?, projectsFilterModel: ProjectsFilterModel?) : ApiResponse?> {
val localVariableConfig = apiV2ProjectsSearchPostRequestConfig(skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue, projectsFilterModel = projectsFilterModel)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2ProjectsSearchPost
*
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param projectsFilterModel (optional)
* @return RequestConfig
*/
fun apiV2ProjectsSearchPostRequestConfig(skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?, projectsFilterModel: ProjectsFilterModel?) : RequestConfig {
val localVariableBody = projectsFilterModel
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (skip != null) {
put("Skip", listOf(skip.toString()))
}
if (take != null) {
put("Take", listOf(take.toString()))
}
if (orderBy != null) {
put("OrderBy", listOf(orderBy.toString()))
}
if (searchField != null) {
put("SearchField", listOf(searchField.toString()))
}
if (searchValue != null) {
put("SearchValue", listOf(searchValue.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/projects/search",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Import project from JSON file in background job
*
* @param file (optional)
* @return java.util.UUID
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun backgroundImportProject(file: java.io.File? = null) : java.util.UUID {
val localVarResponse = backgroundImportProjectWithHttpInfo(file = file)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as java.util.UUID
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Import project from JSON file in background job
*
* @param file (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun backgroundImportProjectWithHttpInfo(file: java.io.File?) : ApiResponse {
val localVariableConfig = backgroundImportProjectRequestConfig(file = file)
return request
© 2015 - 2024 Weber Informatics LLC | Privacy Policy