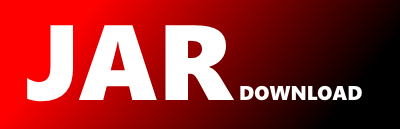
ru.testit.kotlin.client.apis.SectionsApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of testit-api-client-kotlin Show documentation
Show all versions of testit-api-client-kotlin Show documentation
Kotlin API client for TestIT.
/**
*
* Please note:
* This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit this file manually.
*
*/
@file:Suppress(
"ArrayInDataClass",
"EnumEntryName",
"RemoveRedundantQualifierName",
"UnusedImport"
)
package ru.testit.kotlin.client.apis
import java.io.IOException
import okhttp3.OkHttpClient
import okhttp3.HttpUrl
import ru.testit.kotlin.client.models.DeletionState
import ru.testit.kotlin.client.models.Operation
import ru.testit.kotlin.client.models.ProblemDetails
import ru.testit.kotlin.client.models.SectionMoveModel
import ru.testit.kotlin.client.models.SectionPostModel
import ru.testit.kotlin.client.models.SectionPutModel
import ru.testit.kotlin.client.models.SectionRenameModel
import ru.testit.kotlin.client.models.SectionWithStepsModel
import ru.testit.kotlin.client.models.ValidationProblemDetails
import ru.testit.kotlin.client.models.WorkItemShortModel
import com.squareup.moshi.Json
import ru.testit.kotlin.client.infrastructure.ApiClient
import ru.testit.kotlin.client.infrastructure.ApiResponse
import ru.testit.kotlin.client.infrastructure.ClientException
import ru.testit.kotlin.client.infrastructure.ClientError
import ru.testit.kotlin.client.infrastructure.ServerException
import ru.testit.kotlin.client.infrastructure.ServerError
import ru.testit.kotlin.client.infrastructure.MultiValueMap
import ru.testit.kotlin.client.infrastructure.PartConfig
import ru.testit.kotlin.client.infrastructure.RequestConfig
import ru.testit.kotlin.client.infrastructure.RequestMethod
import ru.testit.kotlin.client.infrastructure.ResponseType
import ru.testit.kotlin.client.infrastructure.Success
import ru.testit.kotlin.client.infrastructure.toMultiValue
class SectionsApi(basePath: kotlin.String = defaultBasePath, client: OkHttpClient = ApiClient.defaultClient) : ApiClient(basePath, client) {
companion object {
@JvmStatic
val defaultBasePath: String by lazy {
System.getProperties().getProperty(ApiClient.baseUrlKey, "http://localhost")
}
}
/**
* Patch section
* See <a href=\"https://www.rfc-editor.org/rfc/rfc6902\" target=\"_blank\">RFC 6902: JavaScript Object Notation (JSON) Patch</a> for details
* @param id Section internal (UUID) identifier
* @param operation (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2SectionsIdPatch(id: java.util.UUID, operation: kotlin.collections.List? = null) : Unit {
val localVarResponse = apiV2SectionsIdPatchWithHttpInfo(id = id, operation = operation)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Patch section
* See <a href=\"https://www.rfc-editor.org/rfc/rfc6902\" target=\"_blank\">RFC 6902: JavaScript Object Notation (JSON) Patch</a> for details
* @param id Section internal (UUID) identifier
* @param operation (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2SectionsIdPatchWithHttpInfo(id: java.util.UUID, operation: kotlin.collections.List?) : ApiResponse {
val localVariableConfig = apiV2SectionsIdPatchRequestConfig(id = id, operation = operation)
return request, Unit>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2SectionsIdPatch
*
* @param id Section internal (UUID) identifier
* @param operation (optional)
* @return RequestConfig
*/
fun apiV2SectionsIdPatchRequestConfig(id: java.util.UUID, operation: kotlin.collections.List?) : RequestConfig> {
val localVariableBody = operation
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.PATCH,
path = "/api/v2/sections/{id}".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Create section
* Use case User sets section properties (listed in request example) User runs method execution System creates section property values System returns section (listed in response example)
* @param sectionPostModel (optional)
* @return SectionWithStepsModel
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun createSection(sectionPostModel: SectionPostModel? = null) : SectionWithStepsModel {
val localVarResponse = createSectionWithHttpInfo(sectionPostModel = sectionPostModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as SectionWithStepsModel
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Create section
* Use case User sets section properties (listed in request example) User runs method execution System creates section property values System returns section (listed in response example)
* @param sectionPostModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun createSectionWithHttpInfo(sectionPostModel: SectionPostModel?) : ApiResponse {
val localVariableConfig = createSectionRequestConfig(sectionPostModel = sectionPostModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation createSection
*
* @param sectionPostModel (optional)
* @return RequestConfig
*/
fun createSectionRequestConfig(sectionPostModel: SectionPostModel?) : RequestConfig {
val localVariableBody = sectionPostModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/sections",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Delete section
* Use case User sets section identifier User runs method execution System search section by the identifier System search and delete nested sections of the found section System search and delete workitems related to the found nested sections System deletes initial section and related workitem System returns no content response
* @param id Section internal (UUID) identifier
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun deleteSection(id: java.util.UUID) : Unit {
val localVarResponse = deleteSectionWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Delete section
* Use case User sets section identifier User runs method execution System search section by the identifier System search and delete nested sections of the found section System search and delete workitems related to the found nested sections System deletes initial section and related workitem System returns no content response
* @param id Section internal (UUID) identifier
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun deleteSectionWithHttpInfo(id: java.util.UUID) : ApiResponse {
val localVariableConfig = deleteSectionRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation deleteSection
*
* @param id Section internal (UUID) identifier
* @return RequestConfig
*/
fun deleteSectionRequestConfig(id: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.DELETE,
path = "/api/v2/sections/{id}".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get section
* Use case User sets section internal (guid format) identifier User runs method execution System search section by the section identifier [Optional] If isDeleted flag equals false, deleted work items are not being searched. If true, deleted work items are also being searched, null for all work items. System returns section
* @param id Section internal (UUID) identifier
* @param isDeleted (optional)
* @return SectionWithStepsModel
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun getSectionById(id: java.util.UUID, isDeleted: DeletionState? = null) : SectionWithStepsModel {
val localVarResponse = getSectionByIdWithHttpInfo(id = id, isDeleted = isDeleted)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as SectionWithStepsModel
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get section
* Use case User sets section internal (guid format) identifier User runs method execution System search section by the section identifier [Optional] If isDeleted flag equals false, deleted work items are not being searched. If true, deleted work items are also being searched, null for all work items. System returns section
* @param id Section internal (UUID) identifier
* @param isDeleted (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun getSectionByIdWithHttpInfo(id: java.util.UUID, isDeleted: DeletionState?) : ApiResponse {
val localVariableConfig = getSectionByIdRequestConfig(id = id, isDeleted = isDeleted)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getSectionById
*
* @param id Section internal (UUID) identifier
* @param isDeleted (optional)
* @return RequestConfig
*/
fun getSectionByIdRequestConfig(id: java.util.UUID, isDeleted: DeletionState?) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (isDeleted != null) {
put("isDeleted", listOf(isDeleted.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/sections/{id}".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get section work items
* Use case User sets section identifier User runs method execution System search section by the identifier System search work items related to the section [Optional] If isDeleted flag equals false, deleted work items are not being searched. If true, deleted work items are also being searched, null for all work items. System returns work item collection
* @param id Section internal (UUID) identifier
* @param isDeleted Requested section is deleted (optional, default to false)
* @param tagNames List of work item tags (optional)
* @param includeIterations (optional, default to true)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
@Deprecated(message = "This operation is deprecated.")
fun getWorkItemsBySectionId(id: java.util.UUID, isDeleted: kotlin.Boolean? = false, tagNames: kotlin.collections.List? = null, includeIterations: kotlin.Boolean? = true, skip: kotlin.Int? = null, take: kotlin.Int? = null, orderBy: kotlin.String? = null, searchField: kotlin.String? = null, searchValue: kotlin.String? = null) : kotlin.collections.List {
@Suppress("DEPRECATION")
val localVarResponse = getWorkItemsBySectionIdWithHttpInfo(id = id, isDeleted = isDeleted, tagNames = tagNames, includeIterations = includeIterations, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get section work items
* Use case User sets section identifier User runs method execution System search section by the identifier System search work items related to the section [Optional] If isDeleted flag equals false, deleted work items are not being searched. If true, deleted work items are also being searched, null for all work items. System returns work item collection
* @param id Section internal (UUID) identifier
* @param isDeleted Requested section is deleted (optional, default to false)
* @param tagNames List of work item tags (optional)
* @param includeIterations (optional, default to true)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
@Deprecated(message = "This operation is deprecated.")
fun getWorkItemsBySectionIdWithHttpInfo(id: java.util.UUID, isDeleted: kotlin.Boolean?, tagNames: kotlin.collections.List?, includeIterations: kotlin.Boolean?, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?) : ApiResponse?> {
@Suppress("DEPRECATION")
val localVariableConfig = getWorkItemsBySectionIdRequestConfig(id = id, isDeleted = isDeleted, tagNames = tagNames, includeIterations = includeIterations, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getWorkItemsBySectionId
*
* @param id Section internal (UUID) identifier
* @param isDeleted Requested section is deleted (optional, default to false)
* @param tagNames List of work item tags (optional)
* @param includeIterations (optional, default to true)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return RequestConfig
*/
@Deprecated(message = "This operation is deprecated.")
fun getWorkItemsBySectionIdRequestConfig(id: java.util.UUID, isDeleted: kotlin.Boolean?, tagNames: kotlin.collections.List?, includeIterations: kotlin.Boolean?, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (isDeleted != null) {
put("isDeleted", listOf(isDeleted.toString()))
}
if (tagNames != null) {
put("tagNames", toMultiValue(tagNames.toList(), "multi"))
}
if (includeIterations != null) {
put("includeIterations", listOf(includeIterations.toString()))
}
if (skip != null) {
put("Skip", listOf(skip.toString()))
}
if (take != null) {
put("Take", listOf(take.toString()))
}
if (orderBy != null) {
put("OrderBy", listOf(orderBy.toString()))
}
if (searchField != null) {
put("SearchField", listOf(searchField.toString()))
}
if (searchValue != null) {
put("SearchValue", listOf(searchValue.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/sections/{id}/workItems".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Move section with all work items into another section
*
* @param sectionMoveModel (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun move(sectionMoveModel: SectionMoveModel? = null) : Unit {
val localVarResponse = moveWithHttpInfo(sectionMoveModel = sectionMoveModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Move section with all work items into another section
*
* @param sectionMoveModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun moveWithHttpInfo(sectionMoveModel: SectionMoveModel?) : ApiResponse {
val localVariableConfig = moveRequestConfig(sectionMoveModel = sectionMoveModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation move
*
* @param sectionMoveModel (optional)
* @return RequestConfig
*/
fun moveRequestConfig(sectionMoveModel: SectionMoveModel?) : RequestConfig {
val localVariableBody = sectionMoveModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/sections/move",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Rename section
* Use case User sets section identifier and new name (listed in request example) User runs method execution System search section by the identifier System updates section name using the new name System returns no content response
* @param sectionRenameModel (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun rename(sectionRenameModel: SectionRenameModel? = null) : Unit {
val localVarResponse = renameWithHttpInfo(sectionRenameModel = sectionRenameModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Rename section
* Use case User sets section identifier and new name (listed in request example) User runs method execution System search section by the identifier System updates section name using the new name System returns no content response
* @param sectionRenameModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun renameWithHttpInfo(sectionRenameModel: SectionRenameModel?) : ApiResponse {
val localVariableConfig = renameRequestConfig(sectionRenameModel = sectionRenameModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation rename
*
* @param sectionRenameModel (optional)
* @return RequestConfig
*/
fun renameRequestConfig(sectionRenameModel: SectionRenameModel?) : RequestConfig {
val localVariableBody = sectionRenameModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/sections/rename",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Update section
* Use case User sets section properties (listed in request example) User runs method execution System search section by the identifier System updates section using the property values System returns no content response
* @param sectionPutModel (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun updateSection(sectionPutModel: SectionPutModel? = null) : Unit {
val localVarResponse = updateSectionWithHttpInfo(sectionPutModel = sectionPutModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Update section
* Use case User sets section properties (listed in request example) User runs method execution System search section by the identifier System updates section using the property values System returns no content response
* @param sectionPutModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun updateSectionWithHttpInfo(sectionPutModel: SectionPutModel?) : ApiResponse {
val localVariableConfig = updateSectionRequestConfig(sectionPutModel = sectionPutModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation updateSection
*
* @param sectionPutModel (optional)
* @return RequestConfig
*/
fun updateSectionRequestConfig(sectionPutModel: SectionPutModel?) : RequestConfig {
val localVariableBody = sectionPutModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.PUT,
path = "/api/v2/sections",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
private fun encodeURIComponent(uriComponent: kotlin.String): kotlin.String =
HttpUrl.Builder().scheme("http").host("localhost").addPathSegment(uriComponent).build().encodedPathSegments[0]
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy