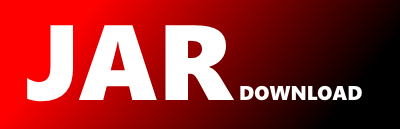
ru.testit.kotlin.client.apis.TestPlansApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of testit-api-client-kotlin Show documentation
Show all versions of testit-api-client-kotlin Show documentation
Kotlin API client for TestIT.
/**
*
* Please note:
* This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit this file manually.
*
*/
@file:Suppress(
"ArrayInDataClass",
"EnumEntryName",
"RemoveRedundantQualifierName",
"UnusedImport"
)
package ru.testit.kotlin.client.apis
import java.io.IOException
import okhttp3.OkHttpClient
import okhttp3.HttpUrl
import ru.testit.kotlin.client.models.ConfigurationModel
import ru.testit.kotlin.client.models.GetXlsxTestPointsByTestPlanModel
import ru.testit.kotlin.client.models.Operation
import ru.testit.kotlin.client.models.ProblemDetails
import ru.testit.kotlin.client.models.TestPlanChangeModel
import ru.testit.kotlin.client.models.TestPlanLink
import ru.testit.kotlin.client.models.TestPlanModel
import ru.testit.kotlin.client.models.TestPlanPostModel
import ru.testit.kotlin.client.models.TestPlanPutModel
import ru.testit.kotlin.client.models.TestPlanShortModel
import ru.testit.kotlin.client.models.TestPlanWithTestSuiteTreeModel
import ru.testit.kotlin.client.models.TestPointAnalyticResult
import ru.testit.kotlin.client.models.TestPointSelectModel
import ru.testit.kotlin.client.models.TestPointWithLastResultModel
import ru.testit.kotlin.client.models.TestRunModel
import ru.testit.kotlin.client.models.TestRunSearchQueryModel
import ru.testit.kotlin.client.models.TestSuiteV2TreeModel
import ru.testit.kotlin.client.models.ValidationProblemDetails
import ru.testit.kotlin.client.models.WorkItemSelectModel
import com.squareup.moshi.Json
import ru.testit.kotlin.client.infrastructure.ApiClient
import ru.testit.kotlin.client.infrastructure.ApiResponse
import ru.testit.kotlin.client.infrastructure.ClientException
import ru.testit.kotlin.client.infrastructure.ClientError
import ru.testit.kotlin.client.infrastructure.ServerException
import ru.testit.kotlin.client.infrastructure.ServerError
import ru.testit.kotlin.client.infrastructure.MultiValueMap
import ru.testit.kotlin.client.infrastructure.PartConfig
import ru.testit.kotlin.client.infrastructure.RequestConfig
import ru.testit.kotlin.client.infrastructure.RequestMethod
import ru.testit.kotlin.client.infrastructure.ResponseType
import ru.testit.kotlin.client.infrastructure.Success
import ru.testit.kotlin.client.infrastructure.toMultiValue
class TestPlansApi(basePath: kotlin.String = defaultBasePath, client: OkHttpClient = ApiClient.defaultClient) : ApiClient(basePath, client) {
companion object {
@JvmStatic
val defaultBasePath: String by lazy {
System.getProperties().getProperty(ApiClient.baseUrlKey, "http://localhost")
}
}
/**
* Add test-points to TestPlan with sections
*
* @param id Test plan internal (guid format) or global (int format) identifier
* @param workItemSelectModel Filter object to retrieve work items for test-suite's project (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun addTestPointsWithSections(id: kotlin.String, workItemSelectModel: WorkItemSelectModel? = null) : Unit {
val localVarResponse = addTestPointsWithSectionsWithHttpInfo(id = id, workItemSelectModel = workItemSelectModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Add test-points to TestPlan with sections
*
* @param id Test plan internal (guid format) or global (int format) identifier
* @param workItemSelectModel Filter object to retrieve work items for test-suite's project (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun addTestPointsWithSectionsWithHttpInfo(id: kotlin.String, workItemSelectModel: WorkItemSelectModel?) : ApiResponse {
val localVariableConfig = addTestPointsWithSectionsRequestConfig(id = id, workItemSelectModel = workItemSelectModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation addTestPointsWithSections
*
* @param id Test plan internal (guid format) or global (int format) identifier
* @param workItemSelectModel Filter object to retrieve work items for test-suite's project (optional)
* @return RequestConfig
*/
fun addTestPointsWithSectionsRequestConfig(id: kotlin.String, workItemSelectModel: WorkItemSelectModel?) : RequestConfig {
val localVariableBody = workItemSelectModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testPlans/{id}/test-points/withSections".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Add WorkItems to TestPlan with Sections as TestSuites
* Use case User sets TestPlan identifier User sets WorkItem identifiers (listed in request example) User runs method execution System added WorkItems and Sections to TestPlan System returns no content response
* @param id Test plan internal (guid format) or global (int format) identifier
* @param javaUtilUUID (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun addWorkItemsWithSections(id: kotlin.String, javaUtilUUID: kotlin.collections.Set? = null) : Unit {
val localVarResponse = addWorkItemsWithSectionsWithHttpInfo(id = id, javaUtilUUID = javaUtilUUID)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Add WorkItems to TestPlan with Sections as TestSuites
* Use case User sets TestPlan identifier User sets WorkItem identifiers (listed in request example) User runs method execution System added WorkItems and Sections to TestPlan System returns no content response
* @param id Test plan internal (guid format) or global (int format) identifier
* @param javaUtilUUID (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun addWorkItemsWithSectionsWithHttpInfo(id: kotlin.String, javaUtilUUID: kotlin.collections.Set?) : ApiResponse {
val localVariableConfig = addWorkItemsWithSectionsRequestConfig(id = id, javaUtilUUID = javaUtilUUID)
return request, Unit>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation addWorkItemsWithSections
*
* @param id Test plan internal (guid format) or global (int format) identifier
* @param javaUtilUUID (optional)
* @return RequestConfig
*/
fun addWorkItemsWithSectionsRequestConfig(id: kotlin.String, javaUtilUUID: kotlin.collections.Set?) : RequestConfig> {
val localVariableBody = javaUtilUUID
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testPlans/{id}/workItems/withSections".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get analytics by TestPlan
* Use case User sets test plan identifier User runs method execution System returns analytics by test plan
* @param id Test plan internal (guid format) or global (int format) identifier
* @return TestPointAnalyticResult
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestPlansIdAnalyticsGet(id: kotlin.String) : TestPointAnalyticResult {
val localVarResponse = apiV2TestPlansIdAnalyticsGetWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as TestPointAnalyticResult
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get analytics by TestPlan
* Use case User sets test plan identifier User runs method execution System returns analytics by test plan
* @param id Test plan internal (guid format) or global (int format) identifier
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestPlansIdAnalyticsGetWithHttpInfo(id: kotlin.String) : ApiResponse {
val localVariableConfig = apiV2TestPlansIdAnalyticsGetRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestPlansIdAnalyticsGet
*
* @param id Test plan internal (guid format) or global (int format) identifier
* @return RequestConfig
*/
fun apiV2TestPlansIdAnalyticsGetRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/testPlans/{id}/analytics".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Distribute test points between the users
*
* @param id Test plan unique or global ID
* @param testers Specifies a project user IDs to distribute (optional)
* @return TestPlanWithTestSuiteTreeModel
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestPlansIdAutobalancePost(id: kotlin.String, testers: kotlin.collections.Set? = null) : TestPlanWithTestSuiteTreeModel {
val localVarResponse = apiV2TestPlansIdAutobalancePostWithHttpInfo(id = id, testers = testers)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as TestPlanWithTestSuiteTreeModel
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Distribute test points between the users
*
* @param id Test plan unique or global ID
* @param testers Specifies a project user IDs to distribute (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestPlansIdAutobalancePostWithHttpInfo(id: kotlin.String, testers: kotlin.collections.Set?) : ApiResponse {
val localVariableConfig = apiV2TestPlansIdAutobalancePostRequestConfig(id = id, testers = testers)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestPlansIdAutobalancePost
*
* @param id Test plan unique or global ID
* @param testers Specifies a project user IDs to distribute (optional)
* @return RequestConfig
*/
fun apiV2TestPlansIdAutobalancePostRequestConfig(id: kotlin.String, testers: kotlin.collections.Set?) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (testers != null) {
put("testers", toMultiValue(testers.toList(), "multi"))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testPlans/{id}/autobalance".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get TestPlan configurations
* Use case User sets test plan identifier User runs method execution System return test plan configurations
* @param id Test plan internal (guid format) or global (int format) identifier
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestPlansIdConfigurationsGet(id: kotlin.String) : kotlin.collections.List {
val localVarResponse = apiV2TestPlansIdConfigurationsGetWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get TestPlan configurations
* Use case User sets test plan identifier User runs method execution System return test plan configurations
* @param id Test plan internal (guid format) or global (int format) identifier
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestPlansIdConfigurationsGetWithHttpInfo(id: kotlin.String) : ApiResponse?> {
val localVariableConfig = apiV2TestPlansIdConfigurationsGetRequestConfig(id = id)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestPlansIdConfigurationsGet
*
* @param id Test plan internal (guid format) or global (int format) identifier
* @return RequestConfig
*/
fun apiV2TestPlansIdConfigurationsGetRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/testPlans/{id}/configurations".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Export TestPoints from TestPlan in xls format
* Use case User sets test plan identifier User sets filter model (listed in request example) User runs method execution System return export xlsx file
* @param id Test plan internal (guid format) or global (int format) identifier
* @param timeZoneOffsetInMinutes (optional)
* @param getXlsxTestPointsByTestPlanModel (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestPlansIdExportTestPointsXlsxPost(id: kotlin.String, timeZoneOffsetInMinutes: kotlin.Long? = null, getXlsxTestPointsByTestPlanModel: GetXlsxTestPointsByTestPlanModel? = null) : Unit {
val localVarResponse = apiV2TestPlansIdExportTestPointsXlsxPostWithHttpInfo(id = id, timeZoneOffsetInMinutes = timeZoneOffsetInMinutes, getXlsxTestPointsByTestPlanModel = getXlsxTestPointsByTestPlanModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Export TestPoints from TestPlan in xls format
* Use case User sets test plan identifier User sets filter model (listed in request example) User runs method execution System return export xlsx file
* @param id Test plan internal (guid format) or global (int format) identifier
* @param timeZoneOffsetInMinutes (optional)
* @param getXlsxTestPointsByTestPlanModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestPlansIdExportTestPointsXlsxPostWithHttpInfo(id: kotlin.String, timeZoneOffsetInMinutes: kotlin.Long?, getXlsxTestPointsByTestPlanModel: GetXlsxTestPointsByTestPlanModel?) : ApiResponse {
val localVariableConfig = apiV2TestPlansIdExportTestPointsXlsxPostRequestConfig(id = id, timeZoneOffsetInMinutes = timeZoneOffsetInMinutes, getXlsxTestPointsByTestPlanModel = getXlsxTestPointsByTestPlanModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestPlansIdExportTestPointsXlsxPost
*
* @param id Test plan internal (guid format) or global (int format) identifier
* @param timeZoneOffsetInMinutes (optional)
* @param getXlsxTestPointsByTestPlanModel (optional)
* @return RequestConfig
*/
fun apiV2TestPlansIdExportTestPointsXlsxPostRequestConfig(id: kotlin.String, timeZoneOffsetInMinutes: kotlin.Long?, getXlsxTestPointsByTestPlanModel: GetXlsxTestPointsByTestPlanModel?) : RequestConfig {
val localVariableBody = getXlsxTestPointsByTestPlanModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
timeZoneOffsetInMinutes?.apply { localVariableHeaders["time-Zone-Offset-In-Minutes"] = this.toString() }
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testPlans/{id}/export/testPoints/xlsx".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Export TestResults history from TestPlan in xls format
* Use case User sets test plan identifier User sets filter model (listed in request example) User runs method execution System return export xlsx file
* @param id Test plan internal (guid format) or global (int format) identifier
* @param mustReturnOnlyLastTestResult (optional)
* @param includeSteps (optional)
* @param includeDeletedTestSuites (optional)
* @param timeZoneOffsetInMinutes (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestPlansIdExportTestResultHistoryXlsxPost(id: kotlin.String, mustReturnOnlyLastTestResult: kotlin.Boolean? = null, includeSteps: kotlin.Boolean? = null, includeDeletedTestSuites: kotlin.Boolean? = null, timeZoneOffsetInMinutes: kotlin.Long? = null) : Unit {
val localVarResponse = apiV2TestPlansIdExportTestResultHistoryXlsxPostWithHttpInfo(id = id, mustReturnOnlyLastTestResult = mustReturnOnlyLastTestResult, includeSteps = includeSteps, includeDeletedTestSuites = includeDeletedTestSuites, timeZoneOffsetInMinutes = timeZoneOffsetInMinutes)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Export TestResults history from TestPlan in xls format
* Use case User sets test plan identifier User sets filter model (listed in request example) User runs method execution System return export xlsx file
* @param id Test plan internal (guid format) or global (int format) identifier
* @param mustReturnOnlyLastTestResult (optional)
* @param includeSteps (optional)
* @param includeDeletedTestSuites (optional)
* @param timeZoneOffsetInMinutes (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestPlansIdExportTestResultHistoryXlsxPostWithHttpInfo(id: kotlin.String, mustReturnOnlyLastTestResult: kotlin.Boolean?, includeSteps: kotlin.Boolean?, includeDeletedTestSuites: kotlin.Boolean?, timeZoneOffsetInMinutes: kotlin.Long?) : ApiResponse {
val localVariableConfig = apiV2TestPlansIdExportTestResultHistoryXlsxPostRequestConfig(id = id, mustReturnOnlyLastTestResult = mustReturnOnlyLastTestResult, includeSteps = includeSteps, includeDeletedTestSuites = includeDeletedTestSuites, timeZoneOffsetInMinutes = timeZoneOffsetInMinutes)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestPlansIdExportTestResultHistoryXlsxPost
*
* @param id Test plan internal (guid format) or global (int format) identifier
* @param mustReturnOnlyLastTestResult (optional)
* @param includeSteps (optional)
* @param includeDeletedTestSuites (optional)
* @param timeZoneOffsetInMinutes (optional)
* @return RequestConfig
*/
fun apiV2TestPlansIdExportTestResultHistoryXlsxPostRequestConfig(id: kotlin.String, mustReturnOnlyLastTestResult: kotlin.Boolean?, includeSteps: kotlin.Boolean?, includeDeletedTestSuites: kotlin.Boolean?, timeZoneOffsetInMinutes: kotlin.Long?) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (mustReturnOnlyLastTestResult != null) {
put("mustReturnOnlyLastTestResult", listOf(mustReturnOnlyLastTestResult.toString()))
}
if (includeSteps != null) {
put("includeSteps", listOf(includeSteps.toString()))
}
if (includeDeletedTestSuites != null) {
put("includeDeletedTestSuites", listOf(includeDeletedTestSuites.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
timeZoneOffsetInMinutes?.apply { localVariableHeaders["time-Zone-Offset-In-Minutes"] = this.toString() }
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testPlans/{id}/export/testResultHistory/xlsx".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get TestPlan history
* Use case User sets test plan identifier User runs method execution System return test plan history
* @param id Test plan internal (guid format) or global (int format) identifier
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestPlansIdHistoryGet(id: kotlin.String, skip: kotlin.Int? = null, take: kotlin.Int? = null, orderBy: kotlin.String? = null, searchField: kotlin.String? = null, searchValue: kotlin.String? = null) : kotlin.collections.List {
val localVarResponse = apiV2TestPlansIdHistoryGetWithHttpInfo(id = id, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get TestPlan history
* Use case User sets test plan identifier User runs method execution System return test plan history
* @param id Test plan internal (guid format) or global (int format) identifier
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestPlansIdHistoryGetWithHttpInfo(id: kotlin.String, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?) : ApiResponse?> {
val localVariableConfig = apiV2TestPlansIdHistoryGetRequestConfig(id = id, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestPlansIdHistoryGet
*
* @param id Test plan internal (guid format) or global (int format) identifier
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return RequestConfig
*/
fun apiV2TestPlansIdHistoryGetRequestConfig(id: kotlin.String, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (skip != null) {
put("Skip", listOf(skip.toString()))
}
if (take != null) {
put("Take", listOf(take.toString()))
}
if (orderBy != null) {
put("OrderBy", listOf(orderBy.toString()))
}
if (searchField != null) {
put("SearchField", listOf(searchField.toString()))
}
if (searchValue != null) {
put("SearchValue", listOf(searchValue.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/testPlans/{id}/history".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get Links of TestPlan
* Use case User sets test plan identifier User sets pagination filter (listed in request example) User runs method execution System returns links of TestPlan
* @param id Test plan internal (guid format) or global (int format) identifier
* @param skip (optional)
* @param take (optional)
* @param orderBy (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestPlansIdLinksGet(id: kotlin.String, skip: kotlin.Int? = null, take: kotlin.Int? = null, orderBy: kotlin.String? = null) : kotlin.collections.List {
val localVarResponse = apiV2TestPlansIdLinksGetWithHttpInfo(id = id, skip = skip, take = take, orderBy = orderBy)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get Links of TestPlan
* Use case User sets test plan identifier User sets pagination filter (listed in request example) User runs method execution System returns links of TestPlan
* @param id Test plan internal (guid format) or global (int format) identifier
* @param skip (optional)
* @param take (optional)
* @param orderBy (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestPlansIdLinksGetWithHttpInfo(id: kotlin.String, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?) : ApiResponse?> {
val localVariableConfig = apiV2TestPlansIdLinksGetRequestConfig(id = id, skip = skip, take = take, orderBy = orderBy)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestPlansIdLinksGet
*
* @param id Test plan internal (guid format) or global (int format) identifier
* @param skip (optional)
* @param take (optional)
* @param orderBy (optional)
* @return RequestConfig
*/
fun apiV2TestPlansIdLinksGetRequestConfig(id: kotlin.String, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (skip != null) {
put("skip", listOf(skip.toString()))
}
if (take != null) {
put("take", listOf(take.toString()))
}
if (orderBy != null) {
put("orderBy", listOf(orderBy.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/testPlans/{id}/links".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Patch test plan
* See <a href=\"https://www.rfc-editor.org/rfc/rfc6902\" target=\"_blank\">RFC 6902: JavaScript Object Notation (JSON) Patch</a> for details
* @param id Unique ID of the test plan
* @param operation (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestPlansIdPatch(id: java.util.UUID, operation: kotlin.collections.List? = null) : Unit {
val localVarResponse = apiV2TestPlansIdPatchWithHttpInfo(id = id, operation = operation)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Patch test plan
* See <a href=\"https://www.rfc-editor.org/rfc/rfc6902\" target=\"_blank\">RFC 6902: JavaScript Object Notation (JSON) Patch</a> for details
* @param id Unique ID of the test plan
* @param operation (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestPlansIdPatchWithHttpInfo(id: java.util.UUID, operation: kotlin.collections.List?) : ApiResponse {
val localVariableConfig = apiV2TestPlansIdPatchRequestConfig(id = id, operation = operation)
return request, Unit>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestPlansIdPatch
*
* @param id Unique ID of the test plan
* @param operation (optional)
* @return RequestConfig
*/
fun apiV2TestPlansIdPatchRequestConfig(id: java.util.UUID, operation: kotlin.collections.List?) : RequestConfig> {
val localVariableBody = operation
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.PATCH,
path = "/api/v2/testPlans/{id}".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get TestPoints with last result from TestPlan
* Use case User sets test plan identifier User sets filter (listed in request example) User runs method execution System return test points with last result from test plan
* @param id Test plan internal (guid format) or global (int format) identifier
* @param testerId (optional)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestPlansIdTestPointsLastResultsGet(id: kotlin.String, testerId: java.util.UUID? = null, skip: kotlin.Int? = null, take: kotlin.Int? = null, orderBy: kotlin.String? = null, searchField: kotlin.String? = null, searchValue: kotlin.String? = null) : kotlin.collections.List {
val localVarResponse = apiV2TestPlansIdTestPointsLastResultsGetWithHttpInfo(id = id, testerId = testerId, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get TestPoints with last result from TestPlan
* Use case User sets test plan identifier User sets filter (listed in request example) User runs method execution System return test points with last result from test plan
* @param id Test plan internal (guid format) or global (int format) identifier
* @param testerId (optional)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestPlansIdTestPointsLastResultsGetWithHttpInfo(id: kotlin.String, testerId: java.util.UUID?, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?) : ApiResponse?> {
val localVariableConfig = apiV2TestPlansIdTestPointsLastResultsGetRequestConfig(id = id, testerId = testerId, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestPlansIdTestPointsLastResultsGet
*
* @param id Test plan internal (guid format) or global (int format) identifier
* @param testerId (optional)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return RequestConfig
*/
fun apiV2TestPlansIdTestPointsLastResultsGetRequestConfig(id: kotlin.String, testerId: java.util.UUID?, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (testerId != null) {
put("testerId", listOf(testerId.toString()))
}
if (skip != null) {
put("Skip", listOf(skip.toString()))
}
if (take != null) {
put("Take", listOf(take.toString()))
}
if (orderBy != null) {
put("OrderBy", listOf(orderBy.toString()))
}
if (searchField != null) {
put("SearchField", listOf(searchField.toString()))
}
if (searchValue != null) {
put("SearchValue", listOf(searchValue.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/testPlans/{id}/testPoints/lastResults".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Reset TestPoints status of TestPlan
* Use case User sets test plan identifier User sets test points identifiers User runs method execution System reset test points statuses of test plan
* @param id Test plan internal (guid format) or global (int format) identifier
* @param javaUtilUUID (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestPlansIdTestPointsResetPost(id: kotlin.String, javaUtilUUID: kotlin.collections.Set? = null) : Unit {
val localVarResponse = apiV2TestPlansIdTestPointsResetPostWithHttpInfo(id = id, javaUtilUUID = javaUtilUUID)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Reset TestPoints status of TestPlan
* Use case User sets test plan identifier User sets test points identifiers User runs method execution System reset test points statuses of test plan
* @param id Test plan internal (guid format) or global (int format) identifier
* @param javaUtilUUID (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestPlansIdTestPointsResetPostWithHttpInfo(id: kotlin.String, javaUtilUUID: kotlin.collections.Set?) : ApiResponse {
val localVariableConfig = apiV2TestPlansIdTestPointsResetPostRequestConfig(id = id, javaUtilUUID = javaUtilUUID)
return request, Unit>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestPlansIdTestPointsResetPost
*
* @param id Test plan internal (guid format) or global (int format) identifier
* @param javaUtilUUID (optional)
* @return RequestConfig
*/
fun apiV2TestPlansIdTestPointsResetPostRequestConfig(id: kotlin.String, javaUtilUUID: kotlin.collections.Set?) : RequestConfig> {
val localVariableBody = javaUtilUUID
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testPlans/{id}/testPoints/reset".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Unassign users from multiple test points
*
* @param id Unique or global ID of the test plan
* @param testPointSelectModel (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestPlansIdTestPointsTesterDelete(id: kotlin.String, testPointSelectModel: TestPointSelectModel? = null) : kotlin.collections.List {
val localVarResponse = apiV2TestPlansIdTestPointsTesterDeleteWithHttpInfo(id = id, testPointSelectModel = testPointSelectModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Unassign users from multiple test points
*
* @param id Unique or global ID of the test plan
* @param testPointSelectModel (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestPlansIdTestPointsTesterDeleteWithHttpInfo(id: kotlin.String, testPointSelectModel: TestPointSelectModel?) : ApiResponse?> {
val localVariableConfig = apiV2TestPlansIdTestPointsTesterDeleteRequestConfig(id = id, testPointSelectModel = testPointSelectModel)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestPlansIdTestPointsTesterDelete
*
* @param id Unique or global ID of the test plan
* @param testPointSelectModel (optional)
* @return RequestConfig
*/
fun apiV2TestPlansIdTestPointsTesterDeleteRequestConfig(id: kotlin.String, testPointSelectModel: TestPointSelectModel?) : RequestConfig {
val localVariableBody = testPointSelectModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.DELETE,
path = "/api/v2/testPlans/{id}/testPoints/tester".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Assign user as a tester to multiple test points
*
* @param id Unique or global ID of the test plan
* @param userId Unique ID of the user
* @param testPointSelectModel (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestPlansIdTestPointsTesterUserIdPost(id: kotlin.String, userId: java.util.UUID, testPointSelectModel: TestPointSelectModel? = null) : kotlin.collections.List {
val localVarResponse = apiV2TestPlansIdTestPointsTesterUserIdPostWithHttpInfo(id = id, userId = userId, testPointSelectModel = testPointSelectModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Assign user as a tester to multiple test points
*
* @param id Unique or global ID of the test plan
* @param userId Unique ID of the user
* @param testPointSelectModel (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestPlansIdTestPointsTesterUserIdPostWithHttpInfo(id: kotlin.String, userId: java.util.UUID, testPointSelectModel: TestPointSelectModel?) : ApiResponse?> {
val localVariableConfig = apiV2TestPlansIdTestPointsTesterUserIdPostRequestConfig(id = id, userId = userId, testPointSelectModel = testPointSelectModel)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestPlansIdTestPointsTesterUserIdPost
*
* @param id Unique or global ID of the test plan
* @param userId Unique ID of the user
* @param testPointSelectModel (optional)
* @return RequestConfig
*/
fun apiV2TestPlansIdTestPointsTesterUserIdPostRequestConfig(id: kotlin.String, userId: java.util.UUID, testPointSelectModel: TestPointSelectModel?) : RequestConfig {
val localVariableBody = testPointSelectModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testPlans/{id}/testPoints/tester/{userId}".replace("{"+"id"+"}", encodeURIComponent(id.toString())).replace("{"+"userId"+"}", encodeURIComponent(userId.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get TestRuns of TestPlan
* Use case User sets test plan identifier User sets TestRun status filter (listed in request example) User runs method execution System returns TestRuns for TestPlan
* @param id Test plan internal (guid format) or global (int format) identifier
* @param notStarted (optional)
* @param inProgress (optional)
* @param stopped (optional)
* @param completed (optional)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestPlansIdTestRunsGet(id: kotlin.String, notStarted: kotlin.Boolean? = null, inProgress: kotlin.Boolean? = null, stopped: kotlin.Boolean? = null, completed: kotlin.Boolean? = null, skip: kotlin.Int? = null, take: kotlin.Int? = null, orderBy: kotlin.String? = null, searchField: kotlin.String? = null, searchValue: kotlin.String? = null) : kotlin.collections.List {
val localVarResponse = apiV2TestPlansIdTestRunsGetWithHttpInfo(id = id, notStarted = notStarted, inProgress = inProgress, stopped = stopped, completed = completed, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get TestRuns of TestPlan
* Use case User sets test plan identifier User sets TestRun status filter (listed in request example) User runs method execution System returns TestRuns for TestPlan
* @param id Test plan internal (guid format) or global (int format) identifier
* @param notStarted (optional)
* @param inProgress (optional)
* @param stopped (optional)
* @param completed (optional)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestPlansIdTestRunsGetWithHttpInfo(id: kotlin.String, notStarted: kotlin.Boolean?, inProgress: kotlin.Boolean?, stopped: kotlin.Boolean?, completed: kotlin.Boolean?, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?) : ApiResponse?> {
val localVariableConfig = apiV2TestPlansIdTestRunsGetRequestConfig(id = id, notStarted = notStarted, inProgress = inProgress, stopped = stopped, completed = completed, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestPlansIdTestRunsGet
*
* @param id Test plan internal (guid format) or global (int format) identifier
* @param notStarted (optional)
* @param inProgress (optional)
* @param stopped (optional)
* @param completed (optional)
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @return RequestConfig
*/
fun apiV2TestPlansIdTestRunsGetRequestConfig(id: kotlin.String, notStarted: kotlin.Boolean?, inProgress: kotlin.Boolean?, stopped: kotlin.Boolean?, completed: kotlin.Boolean?, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (notStarted != null) {
put("notStarted", listOf(notStarted.toString()))
}
if (inProgress != null) {
put("inProgress", listOf(inProgress.toString()))
}
if (stopped != null) {
put("stopped", listOf(stopped.toString()))
}
if (completed != null) {
put("completed", listOf(completed.toString()))
}
if (skip != null) {
put("Skip", listOf(skip.toString()))
}
if (take != null) {
put("Take", listOf(take.toString()))
}
if (orderBy != null) {
put("OrderBy", listOf(orderBy.toString()))
}
if (searchField != null) {
put("SearchField", listOf(searchField.toString()))
}
if (searchValue != null) {
put("SearchValue", listOf(searchValue.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/testPlans/{id}/testRuns".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Search TestRuns of TestPlan
* Use case User sets test plan identifier User sets TestRuns filter (listed in request example) User runs method execution System returns TestRuns for TestPlan
* @param id Test plan internal (guid format) or global (int format) identifier
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param testRunSearchQueryModel (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestPlansIdTestRunsSearchPost(id: kotlin.String, skip: kotlin.Int? = null, take: kotlin.Int? = null, orderBy: kotlin.String? = null, searchField: kotlin.String? = null, searchValue: kotlin.String? = null, testRunSearchQueryModel: TestRunSearchQueryModel? = null) : kotlin.collections.List {
val localVarResponse = apiV2TestPlansIdTestRunsSearchPostWithHttpInfo(id = id, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue, testRunSearchQueryModel = testRunSearchQueryModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Search TestRuns of TestPlan
* Use case User sets test plan identifier User sets TestRuns filter (listed in request example) User runs method execution System returns TestRuns for TestPlan
* @param id Test plan internal (guid format) or global (int format) identifier
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param testRunSearchQueryModel (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestPlansIdTestRunsSearchPostWithHttpInfo(id: kotlin.String, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?, testRunSearchQueryModel: TestRunSearchQueryModel?) : ApiResponse?> {
val localVariableConfig = apiV2TestPlansIdTestRunsSearchPostRequestConfig(id = id, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue, testRunSearchQueryModel = testRunSearchQueryModel)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestPlansIdTestRunsSearchPost
*
* @param id Test plan internal (guid format) or global (int format) identifier
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param testRunSearchQueryModel (optional)
* @return RequestConfig
*/
fun apiV2TestPlansIdTestRunsSearchPostRequestConfig(id: kotlin.String, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?, testRunSearchQueryModel: TestRunSearchQueryModel?) : RequestConfig {
val localVariableBody = testRunSearchQueryModel
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (skip != null) {
put("Skip", listOf(skip.toString()))
}
if (take != null) {
put("Take", listOf(take.toString()))
}
if (orderBy != null) {
put("OrderBy", listOf(orderBy.toString()))
}
if (searchField != null) {
put("SearchField", listOf(searchField.toString()))
}
if (searchValue != null) {
put("SearchValue", listOf(searchValue.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testPlans/{id}/testRuns/search".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get last modification date of test plan's test results
*
* @param id Test plan unique or global ID
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestPlansIdTestRunsTestResultsLastModifiedModifiedDateGet(id: kotlin.String) : Unit {
val localVarResponse = apiV2TestPlansIdTestRunsTestResultsLastModifiedModifiedDateGetWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get last modification date of test plan's test results
*
* @param id Test plan unique or global ID
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestPlansIdTestRunsTestResultsLastModifiedModifiedDateGetWithHttpInfo(id: kotlin.String) : ApiResponse {
val localVariableConfig = apiV2TestPlansIdTestRunsTestResultsLastModifiedModifiedDateGetRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestPlansIdTestRunsTestResultsLastModifiedModifiedDateGet
*
* @param id Test plan unique or global ID
* @return RequestConfig
*/
fun apiV2TestPlansIdTestRunsTestResultsLastModifiedModifiedDateGetRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/testPlans/{id}/testRuns/testResults/lastModified/modifiedDate".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Send unlock TestPlan notification
* Use case User sets test plan identifier User runs method execution System send unlock test plan notification
* @param id Test plan internal (guid format) or global (int format) identifier
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestPlansIdUnlockRequestPost(id: kotlin.String) : Unit {
val localVarResponse = apiV2TestPlansIdUnlockRequestPostWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Send unlock TestPlan notification
* Use case User sets test plan identifier User runs method execution System send unlock test plan notification
* @param id Test plan internal (guid format) or global (int format) identifier
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestPlansIdUnlockRequestPostWithHttpInfo(id: kotlin.String) : ApiResponse {
val localVariableConfig = apiV2TestPlansIdUnlockRequestPostRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestPlansIdUnlockRequestPost
*
* @param id Test plan internal (guid format) or global (int format) identifier
* @return RequestConfig
*/
fun apiV2TestPlansIdUnlockRequestPostRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testPlans/{id}/unlock/request".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get TestPlans short models by Project identifiers
* Use case User sets projects identifiers User runs method execution System return test plans short models (listed in response example)
* @param isDeleted (optional)
* @param javaUtilUUID (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestPlansShortsPost(isDeleted: kotlin.Boolean? = null, javaUtilUUID: kotlin.collections.Set? = null) : kotlin.collections.List {
val localVarResponse = apiV2TestPlansShortsPostWithHttpInfo(isDeleted = isDeleted, javaUtilUUID = javaUtilUUID)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get TestPlans short models by Project identifiers
* Use case User sets projects identifiers User runs method execution System return test plans short models (listed in response example)
* @param isDeleted (optional)
* @param javaUtilUUID (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestPlansShortsPostWithHttpInfo(isDeleted: kotlin.Boolean?, javaUtilUUID: kotlin.collections.Set?) : ApiResponse?> {
val localVariableConfig = apiV2TestPlansShortsPostRequestConfig(isDeleted = isDeleted, javaUtilUUID = javaUtilUUID)
return request, kotlin.collections.List>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestPlansShortsPost
*
* @param isDeleted (optional)
* @param javaUtilUUID (optional)
* @return RequestConfig
*/
fun apiV2TestPlansShortsPostRequestConfig(isDeleted: kotlin.Boolean?, javaUtilUUID: kotlin.collections.Set?) : RequestConfig> {
val localVariableBody = javaUtilUUID
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (isDeleted != null) {
put("isDeleted", listOf(isDeleted.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testPlans/shorts",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Clone TestPlan
* Use case User sets test plan identifier User runs method execution System clones test plan System returns test plan (listed in response example)
* @param id Test plan internal (guid format) or global (int format) identifier
* @return TestPlanModel
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun clone(id: kotlin.String) : TestPlanModel {
val localVarResponse = cloneWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as TestPlanModel
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Clone TestPlan
* Use case User sets test plan identifier User runs method execution System clones test plan System returns test plan (listed in response example)
* @param id Test plan internal (guid format) or global (int format) identifier
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun cloneWithHttpInfo(id: kotlin.String) : ApiResponse {
val localVariableConfig = cloneRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation clone
*
* @param id Test plan internal (guid format) or global (int format) identifier
* @return RequestConfig
*/
fun cloneRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testPlans/{id}/clone".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Complete TestPlan
* Use case User sets test plan identifier User runs method execution System completes the test plan and updates test plan status System returns no content response
* @param id Test plan internal (guid format) or global (int format) identifier
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun complete(id: kotlin.String) : Unit {
val localVarResponse = completeWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Complete TestPlan
* Use case User sets test plan identifier User runs method execution System completes the test plan and updates test plan status System returns no content response
* @param id Test plan internal (guid format) or global (int format) identifier
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun completeWithHttpInfo(id: kotlin.String) : ApiResponse {
val localVariableConfig = completeRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation complete
*
* @param id Test plan internal (guid format) or global (int format) identifier
* @return RequestConfig
*/
fun completeRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testPlans/{id}/complete".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Create TestPlan
* Use case User sets test plan properties (listed in request example) User runs method execution System creates test plan System returns test plan (listed in response example)
* @param testPlanPostModel (optional)
* @return TestPlanModel
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun createTestPlan(testPlanPostModel: TestPlanPostModel? = null) : TestPlanModel {
val localVarResponse = createTestPlanWithHttpInfo(testPlanPostModel = testPlanPostModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as TestPlanModel
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Create TestPlan
* Use case User sets test plan properties (listed in request example) User runs method execution System creates test plan System returns test plan (listed in response example)
* @param testPlanPostModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun createTestPlanWithHttpInfo(testPlanPostModel: TestPlanPostModel?) : ApiResponse {
val localVariableConfig = createTestPlanRequestConfig(testPlanPostModel = testPlanPostModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation createTestPlan
*
* @param testPlanPostModel (optional)
* @return RequestConfig
*/
fun createTestPlanRequestConfig(testPlanPostModel: TestPlanPostModel?) : RequestConfig {
val localVariableBody = testPlanPostModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testPlans",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Delete TestPlan
* Use case User sets test plan identifier User runs method execution System delete test plan System returns no content response
* @param id Test plan internal (guid format) or global (int format) identifier
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun deleteTestPlan(id: kotlin.String) : Unit {
val localVarResponse = deleteTestPlanWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Delete TestPlan
* Use case User sets test plan identifier User runs method execution System delete test plan System returns no content response
* @param id Test plan internal (guid format) or global (int format) identifier
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun deleteTestPlanWithHttpInfo(id: kotlin.String) : ApiResponse {
val localVariableConfig = deleteTestPlanRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation deleteTestPlan
*
* @param id Test plan internal (guid format) or global (int format) identifier
* @return RequestConfig
*/
fun deleteTestPlanRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.DELETE,
path = "/api/v2/testPlans/{id}".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get TestPlan by Id
* Use case User sets test plan identifier User runs method execution System search test plan by the identifier System returns test plan
* @param id Test plan internal (guid format) or global (int format) identifier
* @return TestPlanModel
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun getTestPlanById(id: kotlin.String) : TestPlanModel {
val localVarResponse = getTestPlanByIdWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as TestPlanModel
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get TestPlan by Id
* Use case User sets test plan identifier User runs method execution System search test plan by the identifier System returns test plan
* @param id Test plan internal (guid format) or global (int format) identifier
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun getTestPlanByIdWithHttpInfo(id: kotlin.String) : ApiResponse {
val localVariableConfig = getTestPlanByIdRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getTestPlanById
*
* @param id Test plan internal (guid format) or global (int format) identifier
* @return RequestConfig
*/
fun getTestPlanByIdRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/testPlans/{id}".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get TestSuites Tree By Id
* Use case User sets test plan identifier User runs method execution System finds test suites related to the test plan System returns test suites as a tree model (listed in response example)
* @param id Test plan internal (guid format) or global (int format) identifier
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun getTestSuitesById(id: kotlin.String) : kotlin.collections.List {
val localVarResponse = getTestSuitesByIdWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get TestSuites Tree By Id
* Use case User sets test plan identifier User runs method execution System finds test suites related to the test plan System returns test suites as a tree model (listed in response example)
* @param id Test plan internal (guid format) or global (int format) identifier
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun getTestSuitesByIdWithHttpInfo(id: kotlin.String) : ApiResponse?> {
val localVariableConfig = getTestSuitesByIdRequestConfig(id = id)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getTestSuitesById
*
* @param id Test plan internal (guid format) or global (int format) identifier
* @return RequestConfig
*/
fun getTestSuitesByIdRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/testPlans/{id}/testSuites".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Pause TestPlan
* Use case User sets test plan identifier User runs method execution System pauses the test plan and updates test plan status System returns no content response
* @param id Test plan internal (guid format) or global (int format) identifier
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun pause(id: kotlin.String) : Unit {
val localVarResponse = pauseWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Pause TestPlan
* Use case User sets test plan identifier User runs method execution System pauses the test plan and updates test plan status System returns no content response
* @param id Test plan internal (guid format) or global (int format) identifier
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun pauseWithHttpInfo(id: kotlin.String) : ApiResponse {
val localVariableConfig = pauseRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation pause
*
* @param id Test plan internal (guid format) or global (int format) identifier
* @return RequestConfig
*/
fun pauseRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testPlans/{id}/pause".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Permanently delete test plan from archive
*
* @param id Unique or global ID of the test plan
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun purgeTestPlan(id: kotlin.String) : Unit {
val localVarResponse = purgeTestPlanWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Permanently delete test plan from archive
*
* @param id Unique or global ID of the test plan
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun purgeTestPlanWithHttpInfo(id: kotlin.String) : ApiResponse {
val localVariableConfig = purgeTestPlanRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation purgeTestPlan
*
* @param id Unique or global ID of the test plan
* @return RequestConfig
*/
fun purgeTestPlanRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testPlans/{id}/purge".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Restore TestPlan
* Use case User sets test plan identifier User runs method execution System restores test plan System returns no content response
* @param id Test plan internal (guid format) or global (int format) identifier
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun restoreTestPlan(id: kotlin.String) : Unit {
val localVarResponse = restoreTestPlanWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Restore TestPlan
* Use case User sets test plan identifier User runs method execution System restores test plan System returns no content response
* @param id Test plan internal (guid format) or global (int format) identifier
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun restoreTestPlanWithHttpInfo(id: kotlin.String) : ApiResponse {
val localVariableConfig = restoreTestPlanRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation restoreTestPlan
*
* @param id Test plan internal (guid format) or global (int format) identifier
* @return RequestConfig
*/
fun restoreTestPlanRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testPlans/{id}/restore".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Start TestPlan
* Use case User sets test plan identifier User runs method execution System starts the test plan and updates test plan status System returns no content response
* @param id Test plan internal (guid format) or global (int format) identifier
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun start(id: kotlin.String) : Unit {
val localVarResponse = startWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Start TestPlan
* Use case User sets test plan identifier User runs method execution System starts the test plan and updates test plan status System returns no content response
* @param id Test plan internal (guid format) or global (int format) identifier
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun startWithHttpInfo(id: kotlin.String) : ApiResponse {
val localVariableConfig = startRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation start
*
* @param id Test plan internal (guid format) or global (int format) identifier
* @return RequestConfig
*/
fun startRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testPlans/{id}/start".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Update TestPlan
* Use case User sets test plan properties(listed in request example) User runs method execution System updates test plan System returns no content response
* @param testPlanPutModel (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun updateTestPlan(testPlanPutModel: TestPlanPutModel? = null) : Unit {
val localVarResponse = updateTestPlanWithHttpInfo(testPlanPutModel = testPlanPutModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Update TestPlan
* Use case User sets test plan properties(listed in request example) User runs method execution System updates test plan System returns no content response
* @param testPlanPutModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun updateTestPlanWithHttpInfo(testPlanPutModel: TestPlanPutModel?) : ApiResponse {
val localVariableConfig = updateTestPlanRequestConfig(testPlanPutModel = testPlanPutModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation updateTestPlan
*
* @param testPlanPutModel (optional)
* @return RequestConfig
*/
fun updateTestPlanRequestConfig(testPlanPutModel: TestPlanPutModel?) : RequestConfig {
val localVariableBody = testPlanPutModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.PUT,
path = "/api/v2/testPlans",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
private fun encodeURIComponent(uriComponent: kotlin.String): kotlin.String =
HttpUrl.Builder().scheme("http").host("localhost").addPathSegment(uriComponent).build().encodedPathSegments[0]
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy