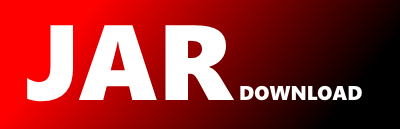
ru.testit.kotlin.client.apis.TestRunsApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of testit-api-client-kotlin Show documentation
Show all versions of testit-api-client-kotlin Show documentation
Kotlin API client for TestIT.
/**
*
* Please note:
* This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit this file manually.
*
*/
@file:Suppress(
"ArrayInDataClass",
"EnumEntryName",
"RemoveRedundantQualifierName",
"UnusedImport"
)
package ru.testit.kotlin.client.apis
import java.io.IOException
import okhttp3.OkHttpClient
import okhttp3.HttpUrl
import ru.testit.kotlin.client.models.AutoTestResultsForTestRunModel
import ru.testit.kotlin.client.models.ProblemDetails
import ru.testit.kotlin.client.models.TestPointResultModel
import ru.testit.kotlin.client.models.TestResultsLocalFilterModel
import ru.testit.kotlin.client.models.TestResultsStatisticsGetModel
import ru.testit.kotlin.client.models.TestRunFillByAutoTestsPostModel
import ru.testit.kotlin.client.models.TestRunFillByConfigurationsPostModel
import ru.testit.kotlin.client.models.TestRunFillByWorkItemsPostModel
import ru.testit.kotlin.client.models.TestRunFilterModel
import ru.testit.kotlin.client.models.TestRunSelectModel
import ru.testit.kotlin.client.models.TestRunShortGetModel
import ru.testit.kotlin.client.models.TestRunTestResultsPartialBulkSetModel
import ru.testit.kotlin.client.models.TestRunUpdateMultipleModel
import ru.testit.kotlin.client.models.TestRunV2GetModel
import ru.testit.kotlin.client.models.TestRunV2PostShortModel
import ru.testit.kotlin.client.models.TestRunV2PutModel
import ru.testit.kotlin.client.models.ValidationProblemDetails
import com.squareup.moshi.Json
import ru.testit.kotlin.client.infrastructure.ApiClient
import ru.testit.kotlin.client.infrastructure.ApiResponse
import ru.testit.kotlin.client.infrastructure.ClientException
import ru.testit.kotlin.client.infrastructure.ClientError
import ru.testit.kotlin.client.infrastructure.ServerException
import ru.testit.kotlin.client.infrastructure.ServerError
import ru.testit.kotlin.client.infrastructure.MultiValueMap
import ru.testit.kotlin.client.infrastructure.PartConfig
import ru.testit.kotlin.client.infrastructure.RequestConfig
import ru.testit.kotlin.client.infrastructure.RequestMethod
import ru.testit.kotlin.client.infrastructure.ResponseType
import ru.testit.kotlin.client.infrastructure.Success
import ru.testit.kotlin.client.infrastructure.toMultiValue
class TestRunsApi(basePath: kotlin.String = defaultBasePath, client: OkHttpClient = ApiClient.defaultClient) : ApiClient(basePath, client) {
companion object {
@JvmStatic
val defaultBasePath: String by lazy {
System.getProperties().getProperty(ApiClient.baseUrlKey, "http://localhost")
}
}
/**
* Delete multiple test runs
* Use case User sets selection parameters of test runs System search and delete collection of test runs System returns the number of deleted test runs
* @param testRunSelectModel (optional)
* @return kotlin.Int
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestRunsDelete(testRunSelectModel: TestRunSelectModel? = null) : kotlin.Int {
val localVarResponse = apiV2TestRunsDeleteWithHttpInfo(testRunSelectModel = testRunSelectModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.Int
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Delete multiple test runs
* Use case User sets selection parameters of test runs System search and delete collection of test runs System returns the number of deleted test runs
* @param testRunSelectModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestRunsDeleteWithHttpInfo(testRunSelectModel: TestRunSelectModel?) : ApiResponse {
val localVariableConfig = apiV2TestRunsDeleteRequestConfig(testRunSelectModel = testRunSelectModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestRunsDelete
*
* @param testRunSelectModel (optional)
* @return RequestConfig
*/
fun apiV2TestRunsDeleteRequestConfig(testRunSelectModel: TestRunSelectModel?) : RequestConfig {
val localVariableBody = testRunSelectModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.DELETE,
path = "/api/v2/testRuns",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Delete test run
* Use case User sets test run internal (guid format) identifier System search and delete test run
* @param id Test run internal (UUID) identifier
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestRunsIdDelete(id: java.util.UUID) : Unit {
val localVarResponse = apiV2TestRunsIdDeleteWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Delete test run
* Use case User sets test run internal (guid format) identifier System search and delete test run
* @param id Test run internal (UUID) identifier
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestRunsIdDeleteWithHttpInfo(id: java.util.UUID) : ApiResponse {
val localVariableConfig = apiV2TestRunsIdDeleteRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestRunsIdDelete
*
* @param id Test run internal (UUID) identifier
* @return RequestConfig
*/
fun apiV2TestRunsIdDeleteRequestConfig(id: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.DELETE,
path = "/api/v2/testRuns/{id}".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Permanently delete test run from archive
* Use case User sets archived test run internal (guid format) identifier System search and purge archived test run
* @param id Test run internal (UUID) identifier
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestRunsIdPurgePost(id: java.util.UUID) : Unit {
val localVarResponse = apiV2TestRunsIdPurgePostWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Permanently delete test run from archive
* Use case User sets archived test run internal (guid format) identifier System search and purge archived test run
* @param id Test run internal (UUID) identifier
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestRunsIdPurgePostWithHttpInfo(id: java.util.UUID) : ApiResponse {
val localVariableConfig = apiV2TestRunsIdPurgePostRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestRunsIdPurgePost
*
* @param id Test run internal (UUID) identifier
* @return RequestConfig
*/
fun apiV2TestRunsIdPurgePostRequestConfig(id: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testRuns/{id}/purge".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Restore test run from the archive
* Use case User sets archived test run internal (guid format) identifier System search and restore test run
* @param id Unique ID of the test run
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestRunsIdRestorePost(id: java.util.UUID) : Unit {
val localVarResponse = apiV2TestRunsIdRestorePostWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Restore test run from the archive
* Use case User sets archived test run internal (guid format) identifier System search and restore test run
* @param id Unique ID of the test run
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestRunsIdRestorePostWithHttpInfo(id: java.util.UUID) : ApiResponse {
val localVariableConfig = apiV2TestRunsIdRestorePostRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestRunsIdRestorePost
*
* @param id Unique ID of the test run
* @return RequestConfig
*/
fun apiV2TestRunsIdRestorePostRequestConfig(id: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testRuns/{id}/restore".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Search for the test run test results and build statistics
*
* @param id Test run unique ID
* @param testResultsLocalFilterModel (optional)
* @return TestResultsStatisticsGetModel
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestRunsIdStatisticsFilterPost(id: java.util.UUID, testResultsLocalFilterModel: TestResultsLocalFilterModel? = null) : TestResultsStatisticsGetModel {
val localVarResponse = apiV2TestRunsIdStatisticsFilterPostWithHttpInfo(id = id, testResultsLocalFilterModel = testResultsLocalFilterModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as TestResultsStatisticsGetModel
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Search for the test run test results and build statistics
*
* @param id Test run unique ID
* @param testResultsLocalFilterModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestRunsIdStatisticsFilterPostWithHttpInfo(id: java.util.UUID, testResultsLocalFilterModel: TestResultsLocalFilterModel?) : ApiResponse {
val localVariableConfig = apiV2TestRunsIdStatisticsFilterPostRequestConfig(id = id, testResultsLocalFilterModel = testResultsLocalFilterModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestRunsIdStatisticsFilterPost
*
* @param id Test run unique ID
* @param testResultsLocalFilterModel (optional)
* @return RequestConfig
*/
fun apiV2TestRunsIdStatisticsFilterPostRequestConfig(id: java.util.UUID, testResultsLocalFilterModel: TestResultsLocalFilterModel?) : RequestConfig {
val localVariableBody = testResultsLocalFilterModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testRuns/{id}/statistics/filter".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get test results from the test run grouped by test points
*
* @param id Test run unique ID
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestRunsIdTestPointsResultsGet(id: java.util.UUID) : kotlin.collections.List {
val localVarResponse = apiV2TestRunsIdTestPointsResultsGetWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get test results from the test run grouped by test points
*
* @param id Test run unique ID
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestRunsIdTestPointsResultsGetWithHttpInfo(id: java.util.UUID) : ApiResponse?> {
val localVariableConfig = apiV2TestRunsIdTestPointsResultsGetRequestConfig(id = id)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestRunsIdTestPointsResultsGet
*
* @param id Test run unique ID
* @return RequestConfig
*/
fun apiV2TestRunsIdTestPointsResultsGetRequestConfig(id: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/testRuns/{id}/testPoints/results".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Partial edit of multiple test results in the test run
*
* @param id Test run unique ID
* @param testRunTestResultsPartialBulkSetModel (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestRunsIdTestResultsBulkPut(id: java.util.UUID, testRunTestResultsPartialBulkSetModel: TestRunTestResultsPartialBulkSetModel? = null) : Unit {
val localVarResponse = apiV2TestRunsIdTestResultsBulkPutWithHttpInfo(id = id, testRunTestResultsPartialBulkSetModel = testRunTestResultsPartialBulkSetModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Partial edit of multiple test results in the test run
*
* @param id Test run unique ID
* @param testRunTestResultsPartialBulkSetModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestRunsIdTestResultsBulkPutWithHttpInfo(id: java.util.UUID, testRunTestResultsPartialBulkSetModel: TestRunTestResultsPartialBulkSetModel?) : ApiResponse {
val localVariableConfig = apiV2TestRunsIdTestResultsBulkPutRequestConfig(id = id, testRunTestResultsPartialBulkSetModel = testRunTestResultsPartialBulkSetModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestRunsIdTestResultsBulkPut
*
* @param id Test run unique ID
* @param testRunTestResultsPartialBulkSetModel (optional)
* @return RequestConfig
*/
fun apiV2TestRunsIdTestResultsBulkPutRequestConfig(id: java.util.UUID, testRunTestResultsPartialBulkSetModel: TestRunTestResultsPartialBulkSetModel?) : RequestConfig {
val localVariableBody = testRunTestResultsPartialBulkSetModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.PUT,
path = "/api/v2/testRuns/{id}/testResults/bulk".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get modification date of last test result of the test run
*
* @param id Test run unique ID
* @return java.time.OffsetDateTime
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestRunsIdTestResultsLastModifiedModificationDateGet(id: java.util.UUID) : java.time.OffsetDateTime {
val localVarResponse = apiV2TestRunsIdTestResultsLastModifiedModificationDateGetWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as java.time.OffsetDateTime
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get modification date of last test result of the test run
*
* @param id Test run unique ID
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestRunsIdTestResultsLastModifiedModificationDateGetWithHttpInfo(id: java.util.UUID) : ApiResponse {
val localVariableConfig = apiV2TestRunsIdTestResultsLastModifiedModificationDateGetRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestRunsIdTestResultsLastModifiedModificationDateGet
*
* @param id Test run unique ID
* @return RequestConfig
*/
fun apiV2TestRunsIdTestResultsLastModifiedModificationDateGetRequestConfig(id: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/testRuns/{id}/testResults/lastModified/modificationDate".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Permanently delete multiple test runs from archive
* Use case User sets selection parameters of archived test runs System search and delete collection of archived test runs System returns the number of deleted archived test runs
* @param testRunSelectModel (optional)
* @return kotlin.Int
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestRunsPurgeBulkPost(testRunSelectModel: TestRunSelectModel? = null) : kotlin.Int {
val localVarResponse = apiV2TestRunsPurgeBulkPostWithHttpInfo(testRunSelectModel = testRunSelectModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.Int
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Permanently delete multiple test runs from archive
* Use case User sets selection parameters of archived test runs System search and delete collection of archived test runs System returns the number of deleted archived test runs
* @param testRunSelectModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestRunsPurgeBulkPostWithHttpInfo(testRunSelectModel: TestRunSelectModel?) : ApiResponse {
val localVariableConfig = apiV2TestRunsPurgeBulkPostRequestConfig(testRunSelectModel = testRunSelectModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestRunsPurgeBulkPost
*
* @param testRunSelectModel (optional)
* @return RequestConfig
*/
fun apiV2TestRunsPurgeBulkPostRequestConfig(testRunSelectModel: TestRunSelectModel?) : RequestConfig {
val localVariableBody = testRunSelectModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testRuns/purge/bulk",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Restore multiple test runs from the archive
* Use case User sets selection parameters of archived test runs System search and restore collection of archived test runs System returns the number of restored test runs
* @param testRunSelectModel (optional)
* @return kotlin.Int
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestRunsRestoreBulkPost(testRunSelectModel: TestRunSelectModel? = null) : kotlin.Int {
val localVarResponse = apiV2TestRunsRestoreBulkPostWithHttpInfo(testRunSelectModel = testRunSelectModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.Int
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Restore multiple test runs from the archive
* Use case User sets selection parameters of archived test runs System search and restore collection of archived test runs System returns the number of restored test runs
* @param testRunSelectModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestRunsRestoreBulkPostWithHttpInfo(testRunSelectModel: TestRunSelectModel?) : ApiResponse {
val localVariableConfig = apiV2TestRunsRestoreBulkPostRequestConfig(testRunSelectModel = testRunSelectModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestRunsRestoreBulkPost
*
* @param testRunSelectModel (optional)
* @return RequestConfig
*/
fun apiV2TestRunsRestoreBulkPostRequestConfig(testRunSelectModel: TestRunSelectModel?) : RequestConfig {
val localVariableBody = testRunSelectModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testRuns/restore/bulk",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Search for test runs
*
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param testRunFilterModel (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestRunsSearchPost(skip: kotlin.Int? = null, take: kotlin.Int? = null, orderBy: kotlin.String? = null, searchField: kotlin.String? = null, searchValue: kotlin.String? = null, testRunFilterModel: TestRunFilterModel? = null) : kotlin.collections.List {
val localVarResponse = apiV2TestRunsSearchPostWithHttpInfo(skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue, testRunFilterModel = testRunFilterModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Search for test runs
*
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param testRunFilterModel (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestRunsSearchPostWithHttpInfo(skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?, testRunFilterModel: TestRunFilterModel?) : ApiResponse?> {
val localVariableConfig = apiV2TestRunsSearchPostRequestConfig(skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue, testRunFilterModel = testRunFilterModel)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestRunsSearchPost
*
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param testRunFilterModel (optional)
* @return RequestConfig
*/
fun apiV2TestRunsSearchPostRequestConfig(skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?, testRunFilterModel: TestRunFilterModel?) : RequestConfig {
val localVariableBody = testRunFilterModel
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (skip != null) {
put("Skip", listOf(skip.toString()))
}
if (take != null) {
put("Take", listOf(take.toString()))
}
if (orderBy != null) {
put("OrderBy", listOf(orderBy.toString()))
}
if (searchField != null) {
put("SearchField", listOf(searchField.toString()))
}
if (searchValue != null) {
put("SearchValue", listOf(searchValue.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testRuns/search",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Update multiple test runs
*
* @param testRunUpdateMultipleModel (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestRunsUpdateMultiplePost(testRunUpdateMultipleModel: TestRunUpdateMultipleModel? = null) : Unit {
val localVarResponse = apiV2TestRunsUpdateMultiplePostWithHttpInfo(testRunUpdateMultipleModel = testRunUpdateMultipleModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Update multiple test runs
*
* @param testRunUpdateMultipleModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestRunsUpdateMultiplePostWithHttpInfo(testRunUpdateMultipleModel: TestRunUpdateMultipleModel?) : ApiResponse {
val localVariableConfig = apiV2TestRunsUpdateMultiplePostRequestConfig(testRunUpdateMultipleModel = testRunUpdateMultipleModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestRunsUpdateMultiplePost
*
* @param testRunUpdateMultipleModel (optional)
* @return RequestConfig
*/
fun apiV2TestRunsUpdateMultiplePostRequestConfig(testRunUpdateMultipleModel: TestRunUpdateMultipleModel?) : RequestConfig {
val localVariableBody = testRunUpdateMultipleModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testRuns/updateMultiple",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Complete TestRun
* Use case User sets test run identifier User runs method execution System completes test run System returns no content response
* @param id Test Run internal identifier (GUID format)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun completeTestRun(id: java.util.UUID) : Unit {
val localVarResponse = completeTestRunWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Complete TestRun
* Use case User sets test run identifier User runs method execution System completes test run System returns no content response
* @param id Test Run internal identifier (GUID format)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun completeTestRunWithHttpInfo(id: java.util.UUID) : ApiResponse {
val localVariableConfig = completeTestRunRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation completeTestRun
*
* @param id Test Run internal identifier (GUID format)
* @return RequestConfig
*/
fun completeTestRunRequestConfig(id: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testRuns/{id}/complete".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Create test runs based on autotests and configurations
* This method creates a test run based on an autotest and a configuration. The difference between the `POST /api/v2/testRuns/byWorkItems` and `POST /api/v2/testRuns/byConfigurations` methods is that in this method there is no need to create a test plan and work items (test cases and checklists).
* @param testRunFillByAutoTestsPostModel (optional)
* @return TestRunV2GetModel
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun createAndFillByAutoTests(testRunFillByAutoTestsPostModel: TestRunFillByAutoTestsPostModel? = null) : TestRunV2GetModel {
val localVarResponse = createAndFillByAutoTestsWithHttpInfo(testRunFillByAutoTestsPostModel = testRunFillByAutoTestsPostModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as TestRunV2GetModel
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Create test runs based on autotests and configurations
* This method creates a test run based on an autotest and a configuration. The difference between the `POST /api/v2/testRuns/byWorkItems` and `POST /api/v2/testRuns/byConfigurations` methods is that in this method there is no need to create a test plan and work items (test cases and checklists).
* @param testRunFillByAutoTestsPostModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun createAndFillByAutoTestsWithHttpInfo(testRunFillByAutoTestsPostModel: TestRunFillByAutoTestsPostModel?) : ApiResponse {
val localVariableConfig = createAndFillByAutoTestsRequestConfig(testRunFillByAutoTestsPostModel = testRunFillByAutoTestsPostModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation createAndFillByAutoTests
*
* @param testRunFillByAutoTestsPostModel (optional)
* @return RequestConfig
*/
fun createAndFillByAutoTestsRequestConfig(testRunFillByAutoTestsPostModel: TestRunFillByAutoTestsPostModel?) : RequestConfig {
val localVariableBody = testRunFillByAutoTestsPostModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testRuns/byAutoTests",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Create test runs picking the needed test points
* This method creates a test run based on a combination of a configuration and a work item(test case or checklist). Before you create a test run using this method, make sure to create a test plan. Work items must be automated. This method is different from the `POST /api/v2/testRuns/byWorkItems` method because of the ability to send a jagged array within the \"<b>testPointSelectors</b>\" parameter.
* @param testRunFillByConfigurationsPostModel (optional)
* @return TestRunV2GetModel
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun createAndFillByConfigurations(testRunFillByConfigurationsPostModel: TestRunFillByConfigurationsPostModel? = null) : TestRunV2GetModel {
val localVarResponse = createAndFillByConfigurationsWithHttpInfo(testRunFillByConfigurationsPostModel = testRunFillByConfigurationsPostModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as TestRunV2GetModel
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Create test runs picking the needed test points
* This method creates a test run based on a combination of a configuration and a work item(test case or checklist). Before you create a test run using this method, make sure to create a test plan. Work items must be automated. This method is different from the `POST /api/v2/testRuns/byWorkItems` method because of the ability to send a jagged array within the \"<b>testPointSelectors</b>\" parameter.
* @param testRunFillByConfigurationsPostModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun createAndFillByConfigurationsWithHttpInfo(testRunFillByConfigurationsPostModel: TestRunFillByConfigurationsPostModel?) : ApiResponse {
val localVariableConfig = createAndFillByConfigurationsRequestConfig(testRunFillByConfigurationsPostModel = testRunFillByConfigurationsPostModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation createAndFillByConfigurations
*
* @param testRunFillByConfigurationsPostModel (optional)
* @return RequestConfig
*/
fun createAndFillByConfigurationsRequestConfig(testRunFillByConfigurationsPostModel: TestRunFillByConfigurationsPostModel?) : RequestConfig {
val localVariableBody = testRunFillByConfigurationsPostModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testRuns/byConfigurations",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Create test run based on configurations and work items
* This method creates a test run based on a combination of configuration and work item (test case or checklist). Before you create a test run using this method, make sure to create a test plan. Work items must be automated.
* @param testRunFillByWorkItemsPostModel (optional)
* @return TestRunV2GetModel
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun createAndFillByWorkItems(testRunFillByWorkItemsPostModel: TestRunFillByWorkItemsPostModel? = null) : TestRunV2GetModel {
val localVarResponse = createAndFillByWorkItemsWithHttpInfo(testRunFillByWorkItemsPostModel = testRunFillByWorkItemsPostModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as TestRunV2GetModel
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Create test run based on configurations and work items
* This method creates a test run based on a combination of configuration and work item (test case or checklist). Before you create a test run using this method, make sure to create a test plan. Work items must be automated.
* @param testRunFillByWorkItemsPostModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun createAndFillByWorkItemsWithHttpInfo(testRunFillByWorkItemsPostModel: TestRunFillByWorkItemsPostModel?) : ApiResponse {
val localVariableConfig = createAndFillByWorkItemsRequestConfig(testRunFillByWorkItemsPostModel = testRunFillByWorkItemsPostModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation createAndFillByWorkItems
*
* @param testRunFillByWorkItemsPostModel (optional)
* @return RequestConfig
*/
fun createAndFillByWorkItemsRequestConfig(testRunFillByWorkItemsPostModel: TestRunFillByWorkItemsPostModel?) : RequestConfig {
val localVariableBody = testRunFillByWorkItemsPostModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testRuns/byWorkItems",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Create empty TestRun
* Use case User sets test run model (listed in the request example) User runs method execution System creates test run System returns test run model
* @param testRunV2PostShortModel (optional)
* @return TestRunV2GetModel
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun createEmpty(testRunV2PostShortModel: TestRunV2PostShortModel? = null) : TestRunV2GetModel {
val localVarResponse = createEmptyWithHttpInfo(testRunV2PostShortModel = testRunV2PostShortModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as TestRunV2GetModel
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Create empty TestRun
* Use case User sets test run model (listed in the request example) User runs method execution System creates test run System returns test run model
* @param testRunV2PostShortModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun createEmptyWithHttpInfo(testRunV2PostShortModel: TestRunV2PostShortModel?) : ApiResponse {
val localVariableConfig = createEmptyRequestConfig(testRunV2PostShortModel = testRunV2PostShortModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation createEmpty
*
* @param testRunV2PostShortModel (optional)
* @return RequestConfig
*/
fun createEmptyRequestConfig(testRunV2PostShortModel: TestRunV2PostShortModel?) : RequestConfig {
val localVariableBody = testRunV2PostShortModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testRuns",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get TestRun by Id
* Use case User sets test run identifier User runs method execution System finds test run System returns test run
* @param id Test Run internal identifier (GUID format)
* @return TestRunV2GetModel
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun getTestRunById(id: java.util.UUID) : TestRunV2GetModel {
val localVarResponse = getTestRunByIdWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as TestRunV2GetModel
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get TestRun by Id
* Use case User sets test run identifier User runs method execution System finds test run System returns test run
* @param id Test Run internal identifier (GUID format)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun getTestRunByIdWithHttpInfo(id: java.util.UUID) : ApiResponse {
val localVariableConfig = getTestRunByIdRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getTestRunById
*
* @param id Test Run internal identifier (GUID format)
* @return RequestConfig
*/
fun getTestRunByIdRequestConfig(id: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/testRuns/{id}".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Send test results to the test runs in the system
* This method sends test results to the test management system.
* @param id Test Run internal identifier (GUID format)
* @param autoTestResultsForTestRunModel (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun setAutoTestResultsForTestRun(id: java.util.UUID, autoTestResultsForTestRunModel: kotlin.collections.List? = null) : kotlin.collections.List {
val localVarResponse = setAutoTestResultsForTestRunWithHttpInfo(id = id, autoTestResultsForTestRunModel = autoTestResultsForTestRunModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Send test results to the test runs in the system
* This method sends test results to the test management system.
* @param id Test Run internal identifier (GUID format)
* @param autoTestResultsForTestRunModel (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun setAutoTestResultsForTestRunWithHttpInfo(id: java.util.UUID, autoTestResultsForTestRunModel: kotlin.collections.List?) : ApiResponse?> {
val localVariableConfig = setAutoTestResultsForTestRunRequestConfig(id = id, autoTestResultsForTestRunModel = autoTestResultsForTestRunModel)
return request, kotlin.collections.List>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation setAutoTestResultsForTestRun
*
* @param id Test Run internal identifier (GUID format)
* @param autoTestResultsForTestRunModel (optional)
* @return RequestConfig
*/
fun setAutoTestResultsForTestRunRequestConfig(id: java.util.UUID, autoTestResultsForTestRunModel: kotlin.collections.List?) : RequestConfig> {
val localVariableBody = autoTestResultsForTestRunModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testRuns/{id}/testResults".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Start TestRun
* Use case User sets test run identifier User runs method execution System starts test run System returns no content response
* @param id Test Run internal identifier (GUID format)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun startTestRun(id: java.util.UUID) : Unit {
val localVarResponse = startTestRunWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Start TestRun
* Use case User sets test run identifier User runs method execution System starts test run System returns no content response
* @param id Test Run internal identifier (GUID format)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun startTestRunWithHttpInfo(id: java.util.UUID) : ApiResponse {
val localVariableConfig = startTestRunRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation startTestRun
*
* @param id Test Run internal identifier (GUID format)
* @return RequestConfig
*/
fun startTestRunRequestConfig(id: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testRuns/{id}/start".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Stop TestRun
* Use case User sets test run identifier User runs method execution System stops test run System returns no content response
* @param id Test Run internal identifier (GUID format)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun stopTestRun(id: java.util.UUID) : Unit {
val localVarResponse = stopTestRunWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Stop TestRun
* Use case User sets test run identifier User runs method execution System stops test run System returns no content response
* @param id Test Run internal identifier (GUID format)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun stopTestRunWithHttpInfo(id: java.util.UUID) : ApiResponse {
val localVariableConfig = stopTestRunRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation stopTestRun
*
* @param id Test Run internal identifier (GUID format)
* @return RequestConfig
*/
fun stopTestRunRequestConfig(id: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testRuns/{id}/stop".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Update empty TestRun
* Use case User sets test run properties (listed in the request example) User runs method execution System updates test run System returns returns no content response
* @param testRunV2PutModel (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun updateEmpty(testRunV2PutModel: TestRunV2PutModel? = null) : Unit {
val localVarResponse = updateEmptyWithHttpInfo(testRunV2PutModel = testRunV2PutModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Update empty TestRun
* Use case User sets test run properties (listed in the request example) User runs method execution System updates test run System returns returns no content response
* @param testRunV2PutModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun updateEmptyWithHttpInfo(testRunV2PutModel: TestRunV2PutModel?) : ApiResponse {
val localVariableConfig = updateEmptyRequestConfig(testRunV2PutModel = testRunV2PutModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation updateEmpty
*
* @param testRunV2PutModel (optional)
* @return RequestConfig
*/
fun updateEmptyRequestConfig(testRunV2PutModel: TestRunV2PutModel?) : RequestConfig {
val localVariableBody = testRunV2PutModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.PUT,
path = "/api/v2/testRuns",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
private fun encodeURIComponent(uriComponent: kotlin.String): kotlin.String =
HttpUrl.Builder().scheme("http").host("localhost").addPathSegment(uriComponent).build().encodedPathSegments[0]
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy