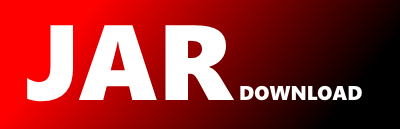
ru.testit.kotlin.client.apis.TestSuitesApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of testit-api-client-kotlin Show documentation
Show all versions of testit-api-client-kotlin Show documentation
Kotlin API client for TestIT.
/**
*
* Please note:
* This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit this file manually.
*
*/
@file:Suppress(
"ArrayInDataClass",
"EnumEntryName",
"RemoveRedundantQualifierName",
"UnusedImport"
)
package ru.testit.kotlin.client.apis
import java.io.IOException
import okhttp3.OkHttpClient
import okhttp3.HttpUrl
import ru.testit.kotlin.client.models.ConfigurationModel
import ru.testit.kotlin.client.models.Operation
import ru.testit.kotlin.client.models.ProblemDetails
import ru.testit.kotlin.client.models.TestPointByTestSuiteModel
import ru.testit.kotlin.client.models.TestResultV2ShortModel
import ru.testit.kotlin.client.models.TestSuiteV2GetModel
import ru.testit.kotlin.client.models.TestSuiteV2PostModel
import ru.testit.kotlin.client.models.TestSuiteV2PutModel
import ru.testit.kotlin.client.models.TestSuiteWorkItemsSearchModel
import ru.testit.kotlin.client.models.ValidationProblemDetails
import ru.testit.kotlin.client.models.WorkItemSelectModel
import ru.testit.kotlin.client.models.WorkItemShortModel
import com.squareup.moshi.Json
import ru.testit.kotlin.client.infrastructure.ApiClient
import ru.testit.kotlin.client.infrastructure.ApiResponse
import ru.testit.kotlin.client.infrastructure.ClientException
import ru.testit.kotlin.client.infrastructure.ClientError
import ru.testit.kotlin.client.infrastructure.ServerException
import ru.testit.kotlin.client.infrastructure.ServerError
import ru.testit.kotlin.client.infrastructure.MultiValueMap
import ru.testit.kotlin.client.infrastructure.PartConfig
import ru.testit.kotlin.client.infrastructure.RequestConfig
import ru.testit.kotlin.client.infrastructure.RequestMethod
import ru.testit.kotlin.client.infrastructure.ResponseType
import ru.testit.kotlin.client.infrastructure.Success
import ru.testit.kotlin.client.infrastructure.toMultiValue
class TestSuitesApi(basePath: kotlin.String = defaultBasePath, client: OkHttpClient = ApiClient.defaultClient) : ApiClient(basePath, client) {
companion object {
@JvmStatic
val defaultBasePath: String by lazy {
System.getProperties().getProperty(ApiClient.baseUrlKey, "http://localhost")
}
}
/**
* Add test-points to test suite
*
* @param id Test suite internal identifier
* @param workItemSelectModel Filter object to retrieve work items for test-suite's project (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun addTestPointsToTestSuite(id: java.util.UUID, workItemSelectModel: WorkItemSelectModel? = null) : Unit {
val localVarResponse = addTestPointsToTestSuiteWithHttpInfo(id = id, workItemSelectModel = workItemSelectModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Add test-points to test suite
*
* @param id Test suite internal identifier
* @param workItemSelectModel Filter object to retrieve work items for test-suite's project (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun addTestPointsToTestSuiteWithHttpInfo(id: java.util.UUID, workItemSelectModel: WorkItemSelectModel?) : ApiResponse {
val localVariableConfig = addTestPointsToTestSuiteRequestConfig(id = id, workItemSelectModel = workItemSelectModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation addTestPointsToTestSuite
*
* @param id Test suite internal identifier
* @param workItemSelectModel Filter object to retrieve work items for test-suite's project (optional)
* @return RequestConfig
*/
fun addTestPointsToTestSuiteRequestConfig(id: java.util.UUID, workItemSelectModel: WorkItemSelectModel?) : RequestConfig {
val localVariableBody = workItemSelectModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testSuites/{id}/test-points".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Patch test suite
* See <a href=\"https://www.rfc-editor.org/rfc/rfc6902\" target=\"_blank\">RFC 6902: JavaScript Object Notation (JSON) Patch</a> for details
* @param id Test Suite internal (UUID) identifier
* @param operation (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestSuitesIdPatch(id: java.util.UUID, operation: kotlin.collections.List? = null) : Unit {
val localVarResponse = apiV2TestSuitesIdPatchWithHttpInfo(id = id, operation = operation)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Patch test suite
* See <a href=\"https://www.rfc-editor.org/rfc/rfc6902\" target=\"_blank\">RFC 6902: JavaScript Object Notation (JSON) Patch</a> for details
* @param id Test Suite internal (UUID) identifier
* @param operation (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestSuitesIdPatchWithHttpInfo(id: java.util.UUID, operation: kotlin.collections.List?) : ApiResponse {
val localVariableConfig = apiV2TestSuitesIdPatchRequestConfig(id = id, operation = operation)
return request, Unit>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestSuitesIdPatch
*
* @param id Test Suite internal (UUID) identifier
* @param operation (optional)
* @return RequestConfig
*/
fun apiV2TestSuitesIdPatchRequestConfig(id: java.util.UUID, operation: kotlin.collections.List?) : RequestConfig> {
val localVariableBody = operation
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.PATCH,
path = "/api/v2/testSuites/{id}".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Refresh test suite. Only dynamic test suites are supported by this method
*
* @param id Test Suite internal (UUID) identifier
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestSuitesIdRefreshPost(id: java.util.UUID) : Unit {
val localVarResponse = apiV2TestSuitesIdRefreshPostWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Refresh test suite. Only dynamic test suites are supported by this method
*
* @param id Test Suite internal (UUID) identifier
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestSuitesIdRefreshPostWithHttpInfo(id: java.util.UUID) : ApiResponse {
val localVariableConfig = apiV2TestSuitesIdRefreshPostRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestSuitesIdRefreshPost
*
* @param id Test Suite internal (UUID) identifier
* @return RequestConfig
*/
fun apiV2TestSuitesIdRefreshPostRequestConfig(id: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testSuites/{id}/refresh".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Set work items for test suite
*
* @param id Unique ID of the test suite
* @param javaUtilUUID (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestSuitesIdWorkItemsPost(id: java.util.UUID, javaUtilUUID: kotlin.collections.Set? = null) : Unit {
val localVarResponse = apiV2TestSuitesIdWorkItemsPostWithHttpInfo(id = id, javaUtilUUID = javaUtilUUID)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Set work items for test suite
*
* @param id Unique ID of the test suite
* @param javaUtilUUID (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestSuitesIdWorkItemsPostWithHttpInfo(id: java.util.UUID, javaUtilUUID: kotlin.collections.Set?) : ApiResponse {
val localVariableConfig = apiV2TestSuitesIdWorkItemsPostRequestConfig(id = id, javaUtilUUID = javaUtilUUID)
return request, Unit>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestSuitesIdWorkItemsPost
*
* @param id Unique ID of the test suite
* @param javaUtilUUID (optional)
* @return RequestConfig
*/
fun apiV2TestSuitesIdWorkItemsPostRequestConfig(id: java.util.UUID, javaUtilUUID: kotlin.collections.Set?) : RequestConfig> {
val localVariableBody = javaUtilUUID
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testSuites/{id}/workItems".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Create test suite
*
* @param testSuiteV2PostModel (optional)
* @return TestSuiteV2GetModel
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestSuitesPost(testSuiteV2PostModel: TestSuiteV2PostModel? = null) : TestSuiteV2GetModel {
val localVarResponse = apiV2TestSuitesPostWithHttpInfo(testSuiteV2PostModel = testSuiteV2PostModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as TestSuiteV2GetModel
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Create test suite
*
* @param testSuiteV2PostModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestSuitesPostWithHttpInfo(testSuiteV2PostModel: TestSuiteV2PostModel?) : ApiResponse {
val localVariableConfig = apiV2TestSuitesPostRequestConfig(testSuiteV2PostModel = testSuiteV2PostModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestSuitesPost
*
* @param testSuiteV2PostModel (optional)
* @return RequestConfig
*/
fun apiV2TestSuitesPostRequestConfig(testSuiteV2PostModel: TestSuiteV2PostModel?) : RequestConfig {
val localVariableBody = testSuiteV2PostModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testSuites",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Edit test suite
*
* @param testSuiteV2PutModel (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2TestSuitesPut(testSuiteV2PutModel: TestSuiteV2PutModel? = null) : Unit {
val localVarResponse = apiV2TestSuitesPutWithHttpInfo(testSuiteV2PutModel = testSuiteV2PutModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Edit test suite
*
* @param testSuiteV2PutModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2TestSuitesPutWithHttpInfo(testSuiteV2PutModel: TestSuiteV2PutModel?) : ApiResponse {
val localVariableConfig = apiV2TestSuitesPutRequestConfig(testSuiteV2PutModel = testSuiteV2PutModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2TestSuitesPut
*
* @param testSuiteV2PutModel (optional)
* @return RequestConfig
*/
fun apiV2TestSuitesPutRequestConfig(testSuiteV2PutModel: TestSuiteV2PutModel?) : RequestConfig {
val localVariableBody = testSuiteV2PutModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.PUT,
path = "/api/v2/testSuites",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Delete TestSuite
* Use case User sets test suite identifier User runs method execution System search test suite by identifier System deletes test suite System returns no content response
* @param id Test suite internal (guid format) identifier\"
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun deleteTestSuite(id: java.util.UUID) : Unit {
val localVarResponse = deleteTestSuiteWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Delete TestSuite
* Use case User sets test suite identifier User runs method execution System search test suite by identifier System deletes test suite System returns no content response
* @param id Test suite internal (guid format) identifier\"
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun deleteTestSuiteWithHttpInfo(id: java.util.UUID) : ApiResponse {
val localVariableConfig = deleteTestSuiteRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation deleteTestSuite
*
* @param id Test suite internal (guid format) identifier\"
* @return RequestConfig
*/
fun deleteTestSuiteRequestConfig(id: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.DELETE,
path = "/api/v2/testSuites/{id}".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get Configurations By Id
* Use case User sets test suite identifier User runs method execution System search test suite by identifier System search test points related to the test suite System search configurations related to the test points System returns configurations array
* @param id Test suite internal (guid format) identifier\"
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun getConfigurationsByTestSuiteId(id: java.util.UUID) : kotlin.collections.List {
val localVarResponse = getConfigurationsByTestSuiteIdWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get Configurations By Id
* Use case User sets test suite identifier User runs method execution System search test suite by identifier System search test points related to the test suite System search configurations related to the test points System returns configurations array
* @param id Test suite internal (guid format) identifier\"
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun getConfigurationsByTestSuiteIdWithHttpInfo(id: java.util.UUID) : ApiResponse?> {
val localVariableConfig = getConfigurationsByTestSuiteIdRequestConfig(id = id)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getConfigurationsByTestSuiteId
*
* @param id Test suite internal (guid format) identifier\"
* @return RequestConfig
*/
fun getConfigurationsByTestSuiteIdRequestConfig(id: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/testSuites/{id}/configurations".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get TestPoints By Id
* Use case User sets test suite identifier User runs method execution System search test suite by identifier System search test points related to the test suite System returns test points array
* @param id Test suite internal (guid format) identifier\"
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun getTestPointsById(id: java.util.UUID) : kotlin.collections.List {
val localVarResponse = getTestPointsByIdWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get TestPoints By Id
* Use case User sets test suite identifier User runs method execution System search test suite by identifier System search test points related to the test suite System returns test points array
* @param id Test suite internal (guid format) identifier\"
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun getTestPointsByIdWithHttpInfo(id: java.util.UUID) : ApiResponse?> {
val localVariableConfig = getTestPointsByIdRequestConfig(id = id)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getTestPointsById
*
* @param id Test suite internal (guid format) identifier\"
* @return RequestConfig
*/
fun getTestPointsByIdRequestConfig(id: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/testSuites/{id}/testPoints".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get TestResults By Id
* Use case User sets test suite identifier User runs method execution System search test suite by identifier System search test points related to the test suite System search test results related to the test points System returns test results array
* @param id Test suite internal (guid format) identifier\"
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun getTestResultsById(id: java.util.UUID) : kotlin.collections.List {
val localVarResponse = getTestResultsByIdWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get TestResults By Id
* Use case User sets test suite identifier User runs method execution System search test suite by identifier System search test points related to the test suite System search test results related to the test points System returns test results array
* @param id Test suite internal (guid format) identifier\"
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun getTestResultsByIdWithHttpInfo(id: java.util.UUID) : ApiResponse?> {
val localVariableConfig = getTestResultsByIdRequestConfig(id = id)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getTestResultsById
*
* @param id Test suite internal (guid format) identifier\"
* @return RequestConfig
*/
fun getTestResultsByIdRequestConfig(id: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/testSuites/{id}/testResults".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get TestSuite by Id
* Use case User sets test suite identifier User runs method execution System search test suite by identifier System returns test suite
* @param id Test suite internal (guid format) identifier\"
* @return TestSuiteV2GetModel
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun getTestSuiteById(id: java.util.UUID) : TestSuiteV2GetModel {
val localVarResponse = getTestSuiteByIdWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as TestSuiteV2GetModel
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get TestSuite by Id
* Use case User sets test suite identifier User runs method execution System search test suite by identifier System returns test suite
* @param id Test suite internal (guid format) identifier\"
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun getTestSuiteByIdWithHttpInfo(id: java.util.UUID) : ApiResponse {
val localVariableConfig = getTestSuiteByIdRequestConfig(id = id)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getTestSuiteById
*
* @param id Test suite internal (guid format) identifier\"
* @return RequestConfig
*/
fun getTestSuiteByIdRequestConfig(id: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/testSuites/{id}".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Search WorkItems
* Use case User sets test suite identifier [Optional] User sets filter User runs method execution System search test suite by identifier System search test points related to the test suite System search work items related to the test points [Optional] User sets filter, system applies filter System returns work items array
* @param id Test suite internal (guid format) identifier\"
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param testSuiteWorkItemsSearchModel (optional)
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
@Deprecated(message = "This operation is deprecated.")
fun searchWorkItems(id: java.util.UUID, skip: kotlin.Int? = null, take: kotlin.Int? = null, orderBy: kotlin.String? = null, searchField: kotlin.String? = null, searchValue: kotlin.String? = null, testSuiteWorkItemsSearchModel: TestSuiteWorkItemsSearchModel? = null) : kotlin.collections.List {
@Suppress("DEPRECATION")
val localVarResponse = searchWorkItemsWithHttpInfo(id = id, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue, testSuiteWorkItemsSearchModel = testSuiteWorkItemsSearchModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Search WorkItems
* Use case User sets test suite identifier [Optional] User sets filter User runs method execution System search test suite by identifier System search test points related to the test suite System search work items related to the test points [Optional] User sets filter, system applies filter System returns work items array
* @param id Test suite internal (guid format) identifier\"
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param testSuiteWorkItemsSearchModel (optional)
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
@Deprecated(message = "This operation is deprecated.")
fun searchWorkItemsWithHttpInfo(id: java.util.UUID, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?, testSuiteWorkItemsSearchModel: TestSuiteWorkItemsSearchModel?) : ApiResponse?> {
@Suppress("DEPRECATION")
val localVariableConfig = searchWorkItemsRequestConfig(id = id, skip = skip, take = take, orderBy = orderBy, searchField = searchField, searchValue = searchValue, testSuiteWorkItemsSearchModel = testSuiteWorkItemsSearchModel)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation searchWorkItems
*
* @param id Test suite internal (guid format) identifier\"
* @param skip Amount of items to be skipped (offset) (optional)
* @param take Amount of items to be taken (limit) (optional)
* @param orderBy SQL-like ORDER BY statement (column1 ASC|DESC , column2 ASC|DESC) (optional)
* @param searchField Property name for searching (optional)
* @param searchValue Value for searching (optional)
* @param testSuiteWorkItemsSearchModel (optional)
* @return RequestConfig
*/
@Deprecated(message = "This operation is deprecated.")
fun searchWorkItemsRequestConfig(id: java.util.UUID, skip: kotlin.Int?, take: kotlin.Int?, orderBy: kotlin.String?, searchField: kotlin.String?, searchValue: kotlin.String?, testSuiteWorkItemsSearchModel: TestSuiteWorkItemsSearchModel?) : RequestConfig {
val localVariableBody = testSuiteWorkItemsSearchModel
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (skip != null) {
put("Skip", listOf(skip.toString()))
}
if (take != null) {
put("Take", listOf(take.toString()))
}
if (orderBy != null) {
put("OrderBy", listOf(orderBy.toString()))
}
if (searchField != null) {
put("SearchField", listOf(searchField.toString()))
}
if (searchValue != null) {
put("SearchValue", listOf(searchValue.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testSuites/{id}/workItems/search".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Set Configurations By TestSuite Id
* Use case User sets test suite identifier User sets collection of configuration identifiers User runs method execution System search test suite by identifier System search test points related to the test suite System search configuration System restores(if exist) or creates test points with listed configuration System returns no content response
* @param id Test suite internal (guid format) identifier\"
* @param javaUtilUUID Collection of configuration identifiers\" (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun setConfigurationsByTestSuiteId(id: java.util.UUID, javaUtilUUID: kotlin.collections.Set? = null) : Unit {
val localVarResponse = setConfigurationsByTestSuiteIdWithHttpInfo(id = id, javaUtilUUID = javaUtilUUID)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Set Configurations By TestSuite Id
* Use case User sets test suite identifier User sets collection of configuration identifiers User runs method execution System search test suite by identifier System search test points related to the test suite System search configuration System restores(if exist) or creates test points with listed configuration System returns no content response
* @param id Test suite internal (guid format) identifier\"
* @param javaUtilUUID Collection of configuration identifiers\" (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun setConfigurationsByTestSuiteIdWithHttpInfo(id: java.util.UUID, javaUtilUUID: kotlin.collections.Set?) : ApiResponse {
val localVariableConfig = setConfigurationsByTestSuiteIdRequestConfig(id = id, javaUtilUUID = javaUtilUUID)
return request, Unit>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation setConfigurationsByTestSuiteId
*
* @param id Test suite internal (guid format) identifier\"
* @param javaUtilUUID Collection of configuration identifiers\" (optional)
* @return RequestConfig
*/
fun setConfigurationsByTestSuiteIdRequestConfig(id: java.util.UUID, javaUtilUUID: kotlin.collections.Set?) : RequestConfig> {
val localVariableBody = javaUtilUUID
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/testSuites/{id}/configurations".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
private fun encodeURIComponent(uriComponent: kotlin.String): kotlin.String =
HttpUrl.Builder().scheme("http").host("localhost").addPathSegment(uriComponent).build().encodedPathSegments[0]
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy