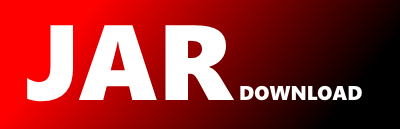
ru.testit.kotlin.client.apis.WorkItemsCommentsApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of testit-api-client-kotlin Show documentation
Show all versions of testit-api-client-kotlin Show documentation
Kotlin API client for TestIT.
/**
*
* Please note:
* This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit this file manually.
*
*/
@file:Suppress(
"ArrayInDataClass",
"EnumEntryName",
"RemoveRedundantQualifierName",
"UnusedImport"
)
package ru.testit.kotlin.client.apis
import java.io.IOException
import okhttp3.OkHttpClient
import okhttp3.HttpUrl
import ru.testit.kotlin.client.models.ProblemDetails
import ru.testit.kotlin.client.models.ValidationProblemDetails
import ru.testit.kotlin.client.models.WorkItemCommentModel
import ru.testit.kotlin.client.models.WorkItemCommentPostModel
import ru.testit.kotlin.client.models.WorkItemCommentPutModel
import com.squareup.moshi.Json
import ru.testit.kotlin.client.infrastructure.ApiClient
import ru.testit.kotlin.client.infrastructure.ApiResponse
import ru.testit.kotlin.client.infrastructure.ClientException
import ru.testit.kotlin.client.infrastructure.ClientError
import ru.testit.kotlin.client.infrastructure.ServerException
import ru.testit.kotlin.client.infrastructure.ServerError
import ru.testit.kotlin.client.infrastructure.MultiValueMap
import ru.testit.kotlin.client.infrastructure.PartConfig
import ru.testit.kotlin.client.infrastructure.RequestConfig
import ru.testit.kotlin.client.infrastructure.RequestMethod
import ru.testit.kotlin.client.infrastructure.ResponseType
import ru.testit.kotlin.client.infrastructure.Success
import ru.testit.kotlin.client.infrastructure.toMultiValue
class WorkItemsCommentsApi(basePath: kotlin.String = defaultBasePath, client: OkHttpClient = ApiClient.defaultClient) : ApiClient(basePath, client) {
companion object {
@JvmStatic
val defaultBasePath: String by lazy {
System.getProperties().getProperty(ApiClient.baseUrlKey, "http://localhost")
}
}
/**
* Delete WorkItem comment
* Use case User sets comment identifier User runs method execution System delete comment System returns success status code
* @param commentId Comment internal (guid format) identifier
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2WorkItemsCommentsCommentIdDelete(commentId: java.util.UUID) : Unit {
val localVarResponse = apiV2WorkItemsCommentsCommentIdDeleteWithHttpInfo(commentId = commentId)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Delete WorkItem comment
* Use case User sets comment identifier User runs method execution System delete comment System returns success status code
* @param commentId Comment internal (guid format) identifier
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2WorkItemsCommentsCommentIdDeleteWithHttpInfo(commentId: java.util.UUID) : ApiResponse {
val localVariableConfig = apiV2WorkItemsCommentsCommentIdDeleteRequestConfig(commentId = commentId)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2WorkItemsCommentsCommentIdDelete
*
* @param commentId Comment internal (guid format) identifier
* @return RequestConfig
*/
fun apiV2WorkItemsCommentsCommentIdDeleteRequestConfig(commentId: java.util.UUID) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.DELETE,
path = "/api/v2/workItems/comments/{commentId}".replace("{"+"commentId"+"}", encodeURIComponent(commentId.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Create WorkItem comment
* Use case User sets comment properties (listed in request parameters) User runs method execution System creates comment System returns comment model (listed in response parameters)
* @param workItemCommentPostModel (optional)
* @return WorkItemCommentModel
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2WorkItemsCommentsPost(workItemCommentPostModel: WorkItemCommentPostModel? = null) : WorkItemCommentModel {
val localVarResponse = apiV2WorkItemsCommentsPostWithHttpInfo(workItemCommentPostModel = workItemCommentPostModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as WorkItemCommentModel
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Create WorkItem comment
* Use case User sets comment properties (listed in request parameters) User runs method execution System creates comment System returns comment model (listed in response parameters)
* @param workItemCommentPostModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2WorkItemsCommentsPostWithHttpInfo(workItemCommentPostModel: WorkItemCommentPostModel?) : ApiResponse {
val localVariableConfig = apiV2WorkItemsCommentsPostRequestConfig(workItemCommentPostModel = workItemCommentPostModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2WorkItemsCommentsPost
*
* @param workItemCommentPostModel (optional)
* @return RequestConfig
*/
fun apiV2WorkItemsCommentsPostRequestConfig(workItemCommentPostModel: WorkItemCommentPostModel?) : RequestConfig {
val localVariableBody = workItemCommentPostModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/api/v2/workItems/comments",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Update work item comment
*
* @param workItemCommentPutModel (optional)
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2WorkItemsCommentsPut(workItemCommentPutModel: WorkItemCommentPutModel? = null) : Unit {
val localVarResponse = apiV2WorkItemsCommentsPutWithHttpInfo(workItemCommentPutModel = workItemCommentPutModel)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Update work item comment
*
* @param workItemCommentPutModel (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun apiV2WorkItemsCommentsPutWithHttpInfo(workItemCommentPutModel: WorkItemCommentPutModel?) : ApiResponse {
val localVariableConfig = apiV2WorkItemsCommentsPutRequestConfig(workItemCommentPutModel = workItemCommentPutModel)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2WorkItemsCommentsPut
*
* @param workItemCommentPutModel (optional)
* @return RequestConfig
*/
fun apiV2WorkItemsCommentsPutRequestConfig(workItemCommentPutModel: WorkItemCommentPutModel?) : RequestConfig {
val localVariableBody = workItemCommentPutModel
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.PUT,
path = "/api/v2/workItems/comments",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Get work item comments
*
* @param id Unique or global ID of the work item
* @return kotlin.collections.List
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun apiV2WorkItemsIdCommentsGet(id: kotlin.String) : kotlin.collections.List {
val localVarResponse = apiV2WorkItemsIdCommentsGetWithHttpInfo(id = id)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as kotlin.collections.List
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Get work item comments
*
* @param id Unique or global ID of the work item
* @return ApiResponse?>
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun apiV2WorkItemsIdCommentsGetWithHttpInfo(id: kotlin.String) : ApiResponse?> {
val localVariableConfig = apiV2WorkItemsIdCommentsGetRequestConfig(id = id)
return request>(
localVariableConfig
)
}
/**
* To obtain the request config of the operation apiV2WorkItemsIdCommentsGet
*
* @param id Unique or global ID of the work item
* @return RequestConfig
*/
fun apiV2WorkItemsIdCommentsGetRequestConfig(id: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/api/v2/workItems/{id}/comments".replace("{"+"id"+"}", encodeURIComponent(id.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
private fun encodeURIComponent(uriComponent: kotlin.String): kotlin.String =
HttpUrl.Builder().scheme("http").host("localhost").addPathSegment(uriComponent).build().encodedPathSegments[0]
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy