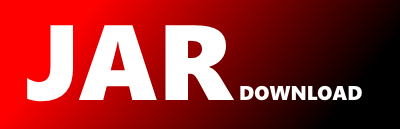
ru.testit.kotlin.client.models.WebHookModel.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of testit-api-client-kotlin Show documentation
Show all versions of testit-api-client-kotlin Show documentation
Kotlin API client for TestIT.
/**
*
* Please note:
* This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit this file manually.
*
*/
@file:Suppress(
"ArrayInDataClass",
"EnumEntryName",
"RemoveRedundantQualifierName",
"UnusedImport"
)
package ru.testit.kotlin.client.models
import ru.testit.kotlin.client.models.RequestTypeModel
import ru.testit.kotlin.client.models.WebHookEventTypeModel
import com.squareup.moshi.Json
import com.squareup.moshi.JsonClass
/**
*
*
* @param name Name of the webhook
* @param eventType Type of event which triggers the webhook
* @param url Url to which the webhook sends request
* @param requestType Method which the webhook uses
* @param shouldSendBody Indicates if the webhook sends body
* @param isEnabled Indicates if the webhook is active
* @param shouldSendCustomBody Indicates if the webhook sends custom body
* @param shouldReplaceParameters Indicates if the webhook injects parameters
* @param shouldEscapeParameters Indicates if the webhook escapes invalid characters in parameters
* @param createdDate Creation date of the webhook
* @param createdById Unique ID of user who created the webhook
* @param projectId Unique ID of project where the webhook is located
* @param id Unique ID of the entity
* @param isDeleted Indicates if the entity is deleted
* @param description Description of the webhook
* @param headers Collection of headers which the webhook sends
* @param queryParameters Collection of query parameters which the webhook sends
* @param customBody Custom body of the webhook
* @param customBodyMediaType MIME type of body of the webhook
* @param modifiedDate Last modification date of the webhook
* @param modifiedById Unique ID of user who modified the webhook last time
*/
data class WebHookModel (
/* Name of the webhook */
@Json(name = "name")
val name: kotlin.String,
/* Type of event which triggers the webhook */
@Json(name = "eventType")
val eventType: WebHookEventTypeModel,
/* Url to which the webhook sends request */
@Json(name = "url")
val url: kotlin.String,
/* Method which the webhook uses */
@Json(name = "requestType")
val requestType: RequestTypeModel,
/* Indicates if the webhook sends body */
@Json(name = "shouldSendBody")
val shouldSendBody: kotlin.Boolean,
/* Indicates if the webhook is active */
@Json(name = "isEnabled")
val isEnabled: kotlin.Boolean,
/* Indicates if the webhook sends custom body */
@Json(name = "shouldSendCustomBody")
val shouldSendCustomBody: kotlin.Boolean,
/* Indicates if the webhook injects parameters */
@Json(name = "shouldReplaceParameters")
val shouldReplaceParameters: kotlin.Boolean,
/* Indicates if the webhook escapes invalid characters in parameters */
@Json(name = "shouldEscapeParameters")
val shouldEscapeParameters: kotlin.Boolean,
/* Creation date of the webhook */
@Json(name = "createdDate")
val createdDate: java.time.OffsetDateTime,
/* Unique ID of user who created the webhook */
@Json(name = "createdById")
val createdById: java.util.UUID,
/* Unique ID of project where the webhook is located */
@Json(name = "projectId")
val projectId: java.util.UUID,
/* Unique ID of the entity */
@Json(name = "id")
val id: java.util.UUID,
/* Indicates if the entity is deleted */
@Json(name = "isDeleted")
val isDeleted: kotlin.Boolean,
/* Description of the webhook */
@Json(name = "description")
val description: kotlin.String? = null,
/* Collection of headers which the webhook sends */
@Json(name = "headers")
val headers: kotlin.collections.Map? = null,
/* Collection of query parameters which the webhook sends */
@Json(name = "queryParameters")
val queryParameters: kotlin.collections.Map? = null,
/* Custom body of the webhook */
@Json(name = "customBody")
val customBody: kotlin.String? = null,
/* MIME type of body of the webhook */
@Json(name = "customBodyMediaType")
val customBodyMediaType: kotlin.String? = null,
/* Last modification date of the webhook */
@Json(name = "modifiedDate")
val modifiedDate: java.time.OffsetDateTime? = null,
/* Unique ID of user who modified the webhook last time */
@Json(name = "modifiedById")
val modifiedById: java.util.UUID? = null
) {
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy