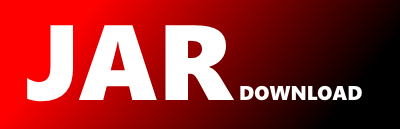
ru.testit.kotlin.client.models.WorkItemShortModel.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of testit-api-client-kotlin Show documentation
Show all versions of testit-api-client-kotlin Show documentation
Kotlin API client for TestIT.
/**
*
* Please note:
* This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit this file manually.
*
*/
@file:Suppress(
"ArrayInDataClass",
"EnumEntryName",
"RemoveRedundantQualifierName",
"UnusedImport"
)
package ru.testit.kotlin.client.models
import ru.testit.kotlin.client.models.IterationModel
import ru.testit.kotlin.client.models.LinkShortModel
import ru.testit.kotlin.client.models.WorkItemPriorityModel
import ru.testit.kotlin.client.models.WorkItemStates
import com.squareup.moshi.Json
import com.squareup.moshi.JsonClass
/**
*
*
* @param id Work Item internal unique identifier
* @param versionId Work Item version identifier
* @param versionNumber Work Item version number
* @param name Work Item name
* @param entityTypeName Work Item type. Possible values: CheckLists, SharedSteps, TestCases
* @param projectId Project unique identifier
* @param sectionId Identifier of Section where Work Item is located
* @param sectionName Section name of Work Item
* @param isAutomated Boolean flag determining whether Work Item is automated
* @param globalId Work Item global identifier
* @param duration Work Item duration
* @param createdById Unique identifier of user who created Work Item
* @param state The current state of Work Item
* @param priority Work Item priority level
* @param isDeleted Flag determining whether Work Item is deleted
* @param iterations Set of iterations related to Work Item
* @param links Set of links related to Work Item
* @param medianDuration Work Item median duration
* @param attributes Work Item attributes
* @param modifiedById Unique identifier of user who applied the latest modification of Work Item
* @param createdDate Date and time of Work Item creation
* @param modifiedDate Date and time of the latest modification of Work Item
* @param tagNames Array of tag names of Work Item
*/
data class WorkItemShortModel (
/* Work Item internal unique identifier */
@Json(name = "id")
val id: java.util.UUID,
/* Work Item version identifier */
@Json(name = "versionId")
val versionId: java.util.UUID,
/* Work Item version number */
@Json(name = "versionNumber")
val versionNumber: kotlin.Int,
/* Work Item name */
@Json(name = "name")
val name: kotlin.String,
/* Work Item type. Possible values: CheckLists, SharedSteps, TestCases */
@Json(name = "entityTypeName")
val entityTypeName: kotlin.String,
/* Project unique identifier */
@Json(name = "projectId")
val projectId: java.util.UUID,
/* Identifier of Section where Work Item is located */
@Json(name = "sectionId")
val sectionId: java.util.UUID,
/* Section name of Work Item */
@Json(name = "sectionName")
val sectionName: kotlin.String,
/* Boolean flag determining whether Work Item is automated */
@Json(name = "isAutomated")
val isAutomated: kotlin.Boolean,
/* Work Item global identifier */
@Json(name = "globalId")
val globalId: kotlin.Long,
/* Work Item duration */
@Json(name = "duration")
val duration: kotlin.Int,
/* Unique identifier of user who created Work Item */
@Json(name = "createdById")
val createdById: java.util.UUID,
/* The current state of Work Item */
@Json(name = "state")
val state: WorkItemStates,
/* Work Item priority level */
@Json(name = "priority")
val priority: WorkItemPriorityModel,
/* Flag determining whether Work Item is deleted */
@Json(name = "isDeleted")
val isDeleted: kotlin.Boolean,
/* Set of iterations related to Work Item */
@Json(name = "iterations")
val iterations: kotlin.collections.List,
/* Set of links related to Work Item */
@Json(name = "links")
val links: kotlin.collections.List,
/* Work Item median duration */
@Json(name = "medianDuration")
val medianDuration: kotlin.Long? = null,
/* Work Item attributes */
@Json(name = "attributes")
val attributes: kotlin.collections.Map? = null,
/* Unique identifier of user who applied the latest modification of Work Item */
@Json(name = "modifiedById")
val modifiedById: java.util.UUID? = null,
/* Date and time of Work Item creation */
@Json(name = "createdDate")
val createdDate: java.time.OffsetDateTime? = null,
/* Date and time of the latest modification of Work Item */
@Json(name = "modifiedDate")
val modifiedDate: java.time.OffsetDateTime? = null,
/* Array of tag names of Work Item */
@Json(name = "tagNames")
val tagNames: kotlin.collections.List? = null
) {
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy