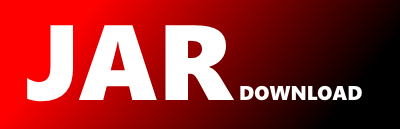
ru.tinkoff.allure.AllureStorage.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of allure-common Show documentation
Show all versions of allure-common Show documentation
Android library for generating instrumentation reports in Allure format
The newest version!
package ru.tinkoff.allure
import ru.tinkoff.allure.model.StepResult
import ru.tinkoff.allure.model.TestResult
import ru.tinkoff.allure.model.TestResultContainer
import ru.tinkoff.allure.model.WithSteps
import java.util.*
import java.util.concurrent.ConcurrentHashMap
/**
* @author Badya on 18.04.2017.
*/
internal object AllureStorage {
private val storage: MutableMap = ConcurrentHashMap()
private val currentStepContext: ThreadLocal> = object : InheritableThreadLocal>() {
public override fun initialValue() = LinkedList()
}
fun getCurrentStep(): String {
return requireNotNull(currentStepContext.get().firstOrNull()) { "Current Step doesn't Exist" }
}
fun getTest(): String {
return requireNotNull(currentStepContext.get().lastOrNull()) { "Root Step doesn't Exist" }
}
fun startTest(uuid: String) {
currentStepContext.get().push(uuid)
}
fun startStep(uuid: String) {
currentStepContext.get().push(uuid)
}
fun stopStep() {
currentStepContext.get().pop()
}
fun clearStepContext() {
currentStepContext.remove()
}
fun getStep(uuid: String?): StepResult {
return get(uuid, StepResult::class.java)
}
fun addStep(parentUUID: String?, stepResult: StepResult) {
put(stepResult.uuid, stepResult)
get(parentUUID, WithSteps::class.java).steps.add(stepResult)
}
fun removeStep(uuid: String?): StepResult? {
return remove(uuid, StepResult::class.java)
}
fun getContainer(uuid: String?): TestResultContainer? {
return get(uuid, TestResultContainer::class.java)
}
fun addContainer(container: TestResultContainer) {
put(container.uuid, container)
}
fun removeContainer(uuid: String?): TestResultContainer? {
return remove(uuid, TestResultContainer::class.java)
}
fun getTestResult(uuid: String?): TestResult? {
return get(uuid, TestResult::class.java)
}
fun addTestResult(testResult: TestResult) {
put(testResult.uuid, testResult)
}
fun removeTestResult(uuid: String?): TestResult? {
return remove(uuid, TestResult::class.java)
}
internal fun put(uuid: String?, item: T): T {
val key = requireNotNull(uuid, { "Failed to put item to storage: uuid can't be null" })
storage[key] = item
return item
}
internal fun get(uuid: String?, type: Class): T {
val key = requireNotNull(uuid) { "Failed to get item from storage: uuid can't be null" }
return type.cast(storage[key])
}
internal fun remove(uuid: String?, type: Class): T {
val key = requireNotNull(uuid, { "Failed to remove item from storage: uuid can't be null" })
return type.cast(storage.remove(key))
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy