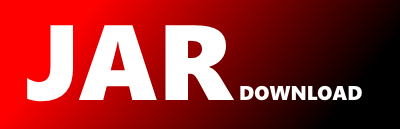
ru.tinkoff.kora.s3.client.minio.MinioS3Object Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of s3-client-minio Show documentation
Show all versions of s3-client-minio Show documentation
Kora s3-client-minio module
package ru.tinkoff.kora.s3.client.minio;
import io.minio.GetObjectResponse;
import okhttp3.Headers;
import org.jetbrains.annotations.ApiStatus;
import ru.tinkoff.kora.s3.client.model.S3Body;
import ru.tinkoff.kora.s3.client.model.S3Object;
import ru.tinkoff.kora.s3.client.model.S3ObjectMeta;
import java.io.IOException;
import java.io.UncheckedIOException;
import java.time.Instant;
import java.util.Objects;
@ApiStatus.Experimental
final class MinioS3Object implements S3Object, S3ObjectMeta {
private final S3Body body;
private final String key;
private final Instant modified;
private final long size;
public MinioS3Object(GetObjectResponse response) {
this.key = response.object();
this.modified = response.headers().getInstant("Modified");
try {
final Headers headers = response.headers();
this.size = headers.get("Content-Length") == null
? response.available()
: Long.valueOf(headers.get("Content-Length"));
this.body = new MinioS3Body(response, size, headers.get("Content-Encoding"), headers.get("Content-Type"));
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}
@Override
public String key() {
return key;
}
@Override
public Instant modified() {
return modified;
}
@Override
public long size() {
return size;
}
@Override
public S3Body body() {
return body;
}
@Override
public boolean equals(Object object) {
if (this == object) return true;
if (object == null || getClass() != object.getClass()) return false;
MinioS3Object that = (MinioS3Object) object;
return size == that.size && Objects.equals(key, that.key);
}
@Override
public int hashCode() {
return Objects.hash(key, size);
}
@Override
public String toString() {
return "MinioS3Object{key=" + key +
", size=" + size +
", modified=" + modified +
'}';
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy