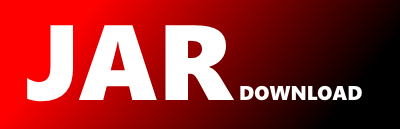
ru.tinkoff.kora.config.common.ConfigValue Maven / Gradle / Ivy
The newest version!
package ru.tinkoff.kora.config.common;
import jakarta.annotation.Nullable;
import ru.tinkoff.kora.config.common.extractor.ConfigValueExtractionException;
import java.math.BigDecimal;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.stream.Collectors;
/**
* Русский: Базовое предоставления значения в конфигурации в Kora.
*
* English: Basic value representation in the configuration in Kora.
*/
sealed public interface ConfigValue {
@Nullable
T value();
ConfigValueOrigin origin();
default String asString() {
if (this instanceof ConfigValue.StringValue str) {
return str.value();
} else if (this instanceof ConfigValue.NumberValue number) {
return number.value().toString();
} else if (this instanceof ConfigValue.BooleanValue booleanValue) {
return booleanValue.value() ? "true" : "false";
} else {
throw ConfigValueExtractionException.unexpectedValueType(this, ConfigValue.StringValue.class);
}
}
default Number asNumber() {
if (this instanceof ConfigValue.StringValue str) {
try {
return new BigDecimal(str.value());
} catch (NumberFormatException e) {
throw ConfigValueExtractionException.parsingError(this, e);
}
} else if (this instanceof ConfigValue.NumberValue number) {
return number.value();
} else {
throw ConfigValueExtractionException.unexpectedValueType(this, ConfigValue.NumberValue.class);
}
}
default ArrayValue asArray() {
if (this instanceof ArrayValue arrayValue) {
return arrayValue;
}
throw ConfigValueExtractionException.unexpectedValueType(this, ConfigValue.ArrayValue.class);
}
default ObjectValue asObject() {
if (this instanceof ObjectValue object) {
return object;
}
throw ConfigValueExtractionException.unexpectedValueType(this, ConfigValue.ObjectValue.class);
}
default boolean asBoolean() {
if (this instanceof ConfigValue.StringValue str) {
return Boolean.parseBoolean(str.value());
}
if (this instanceof BooleanValue bv) {
return bv.value;
}
throw ConfigValueExtractionException.unexpectedValueType(this, ConfigValue.BooleanValue.class);
}
default boolean isNull() {
return this instanceof NullValue;
}
record NullValue(ConfigValueOrigin origin) implements ConfigValue {
public NullValue {
Objects.requireNonNull(origin);
}
@Override
public String toString() {
return "null";
}
@Override
public Void value() {
return null;
}
}
record BooleanValue(ConfigValueOrigin origin, Boolean value) implements ConfigValue {
public BooleanValue {
Objects.requireNonNull(origin);
Objects.requireNonNull(value);
}
@Override
public String toString() {
return Objects.toString(value);
}
}
record StringValue(ConfigValueOrigin origin, String value) implements ConfigValue {
public StringValue {
Objects.requireNonNull(origin);
Objects.requireNonNull(value);
}
@Override
public String toString() {
return "\"" + this.value + "\"";
}
}
record NumberValue(ConfigValueOrigin origin, Number value) implements ConfigValue {
public NumberValue {
Objects.requireNonNull(origin);
Objects.requireNonNull(value);
}
@Override
public String toString() {
return value.toString();
}
}
record ArrayValue(ConfigValueOrigin origin, List> value) implements ConfigValue>>, Iterable> {
public ArrayValue {
Objects.requireNonNull(origin);
Objects.requireNonNull(value);
}
public ConfigValue> get(int i) {
return Objects.requireNonNull(this.value.get(i));
}
@Override
public Iterator> iterator() {
return this.value.iterator();
}
@Override
public String toString() {
return value.stream().map(Objects::toString).collect(Collectors.joining(", ", "[", "]"));
}
}
record ObjectValue(ConfigValueOrigin origin, Map> value) implements ConfigValue
© 2015 - 2024 Weber Informatics LLC | Privacy Policy