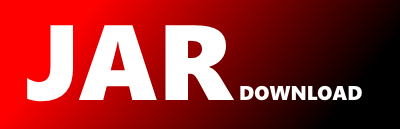
ru.vyarus.gradle.plugin.teavm.task.CompileParameters Maven / Gradle / Ivy
package ru.vyarus.gradle.plugin.teavm.task;
import org.gradle.api.file.Directory;
import org.gradle.api.file.DirectoryProperty;
import org.gradle.api.file.RegularFileProperty;
import org.gradle.api.provider.ListProperty;
import org.gradle.api.provider.MapProperty;
import org.gradle.api.provider.Property;
import org.gradle.workers.WorkParameters;
import org.teavm.backend.wasm.render.WasmBinaryVersion;
import org.teavm.tooling.TeaVMTargetType;
import org.teavm.vm.TeaVMOptimizationLevel;
import java.io.File;
/**
* Parameters for teavm compiler worker.
*
* @author Vyacheslav Rusakov
* @since 06.01.2023
*/
public interface CompileParameters extends WorkParameters {
/**
* @return true to print teavm compilation details
*/
Property getDebug();
/**
* Worker process can't directly return anything, so special file used as error indicator.
*
* @return error indication file
*/
RegularFileProperty getErrorFile();
/**
* @return all directories with compiled classes and classpath jar files
*/
ListProperty getClassPathEntries();
/**
* @return all directories with sources
*/
ListProperty getSourceDirectories();
/**
* @return list of source jar files
*/
ListProperty getSourceJars();
/**
* @return target directory
*/
DirectoryProperty getTargetDirectory();
/**
* @return teavm cache directory
*/
DirectoryProperty getCacheDirectory();
/**
* @return main class name (entry point)
*/
Property getMainClass();
/**
* @return entry point name (main by default)
*/
Property getEntryPointName();
/**
* @return target file name (by default depends on target: classes.js, classes.wasm)
*/
Property getTargetFileName();
/**
* @return teavm compilation target (js, wasm)
*/
Property getTargetType();
/**
* @return wasm version
*/
Property getWasmVersion();
/**
* @return true to minimize compiled js
*/
Property getObfuscated();
/**
* @return true for strict mode
*/
Property getStrict();
/**
* @return true to copy source files (required for browser debug with source maps)
*/
Property getSourceFilesCopied();
/**
* @return tue for incremental compilation
*/
Property getIncremental();
/**
* @return true to generate debug info file (required for debug server)
*/
Property getDebugInformationGenerated();
/**
* @return true for source maps generation
*/
Property getSourceMapsFileGenerated();
/**
* @return true for short file names (C target)
*/
Property getShortFileNames();
/**
* @return true for long jmp (C target)
*/
Property getLongjmpSupported();
/**
* @return true for heap dump (C target)
*/
Property getHeapDump();
/**
* ONLY for development because it affects optimization level (set to SIMPLE).
*
* @return true for fast dependencies analysis
*/
Property getFastDependencyAnalysis();
/**
* @return true to remove assertions during compilation
*/
Property getAssertionsRemoved();
/**
* @return max top level names (JS target only)
*/
Property getMaxTopLevelNames();
/**
* @return min heap size (WASM and C targets)
*/
Property getMinHeapSize();
/**
* @return max heap size (WASM and C targets)
*/
Property getMaxHeapSize();
/**
* @return optimization level (SIMPLE - minimal, ADVANCED - prod. FULL - for WASM)
*/
Property getOptimizationLevel();
/**
* @return list of transformer classes (transforming ClassHolders)
*/
ListProperty getTransformers();
/**
* @return properties for teavm plugins
*/
MapProperty getProperties();
/**
* @return classes to preserve
*/
ListProperty getClassesToPreserve();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy