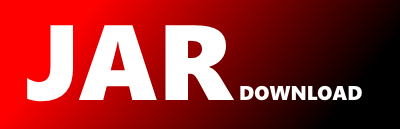
cocaine.Sessions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cocaine-core Show documentation
Show all versions of cocaine-core Show documentation
Core classes for Cocaine Application Engine.
package cocaine;
import java.util.Arrays;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.atomic.AtomicLong;
import org.apache.log4j.Logger;
import rx.Observable;
import rx.Observer;
import rx.subjects.ReplaySubject;
import rx.subjects.Subject;
/**
* @author Anton Bobukh
*/
public final class Sessions {
private static final Logger logger = Logger.getLogger(Sessions.class);
private final AtomicLong counter;
private final Map> sessions;
private final String service;
public Sessions(String service) {
this.service = service;
this.counter = new AtomicLong(0);
this.sessions = new ConcurrentHashMap<>();
}
public Session create() {
long id = counter.getAndIncrement();
Subject subject = ReplaySubject.create();
logger.debug("Creating new session: " + id);
sessions.put(id, subject);
return new Session(id, subject);
}
public void onChunk(long id, byte[] chunk) {
Observer session = sessions.get(id);
if (session != null) {
logger.debug("Pushing new chunk " + Arrays.toString(chunk) + " to session " + id);
session.onNext(chunk);
} else {
logger.warn("Session " + id + " does not exist");
}
}
public void onCompleted(long id) {
Observer session = sessions.remove(id);
if (session != null) {
logger.debug("Closing session " + id);
session.onCompleted();
} else {
logger.warn("Session " + id + " does not exist");
}
}
public void onError(long id, ServiceException exception) {
Observer session = sessions.remove(id);
if (session != null) {
logger.debug("Setting error " + exception.getMessage() + " for session " + id);
session.onError(exception);
} else {
logger.warn("Session " + id + " does not exist");
}
}
public void onCompleted() {
logger.debug("Closing all sessions of " + service);
for (long session : sessions.keySet()) {
onCompleted(session);
}
}
public void onError(ServiceException exception) {
logger.debug("Setting errors for all sessions of " + service);
for (long session : sessions.keySet()) {
onError(session, exception);
}
}
public static final class Session {
private final long id;
private final Observable observable;
private Session(long id, Observable observable) {
this.id = id;
this.observable = observable;
}
public long getId() {
return id;
}
public Observable getObservable() {
return observable;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy