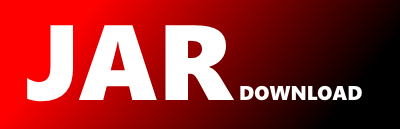
ru.yandex.qatools.allure.data.plugins.PluginManager.groovy Maven / Gradle / Ivy
package ru.yandex.qatools.allure.data.plugins
import com.google.common.hash.Hashing
import com.google.common.reflect.ClassPath
import com.google.inject.Inject
import groovy.transform.CompileStatic
import ru.yandex.qatools.allure.data.Widgets
import ru.yandex.qatools.allure.data.io.ReportWriter
import ru.yandex.qatools.allure.data.utils.PluginUtils
import java.nio.charset.StandardCharsets
/**
* Plugin manager helps you to find plugins and run
* only plugins you need.
*
* @author Dmitry Baev [email protected]
* Date: 17.02.15
*/
@CompileStatic
class PluginManager {
/**
* File with this name contains list of plugins with resources.
*/
public static final String PLUGINS_JSON = "plugins.json"
public static final String WIDGETS_JSON = "widgets.json"
protected final PluginStorage preparePlugins
protected final PluginStorage processPlugins
protected final List pluginsWithResources
protected final List pluginsWithWidgets
protected final List pluginsWithData
/**
* Create an instance of plugin manager.
*/
@Inject
PluginManager(PluginsIndex index) {
preparePlugins = new PluginStorage<>(index.findAll(PreparePlugin))
processPlugins = new PluginStorage<>(index.findAll(ProcessPlugin))
pluginsWithResources = index.findAll(WithResources)
pluginsWithWidgets = index.findAll(WithWidget)
pluginsWithData = index.findAll(WithData)
}
/**
* Get list of names of plugins with resources.
*/
List getPluginsNames() {
pluginsWithResources.collect { plugin ->
plugin.name
}
}
/**
* Get all data for plugins with data.
*/
List getPluginsData() {
pluginsWithData*.pluginData.flatten() as List
}
/**
* Get all plugin widgets.
*/
List getWidgets() {
pluginsWithWidgets*.widget
}
/**
* Find all prepare plugins an process given object for
* each of found plugins.
*/
public void prepare(T object) {
def plugins = (preparePlugins.get(object?.class) ?: []) as List
plugins*.prepare(object)
}
/**
* Find all process plugins an process given object for
* each of found plugins.
*/
public void process(T object) {
def plugins = (processPlugins.get(object?.class) ?: []) as List
for (def plugin : plugins) {
plugin.process(PluginUtils.clone(object))
}
}
/**
* Write list of plugins with resources to {@link #PLUGINS_JSON}
*
* @see ReportWriter
*/
void writePluginList(ReportWriter writer) {
writer.write(new PluginData(PLUGINS_JSON, pluginsNames))
}
/**
* Write plugins widgets to {@link #WIDGETS_JSON}.
*
* @see ReportWriter
*/
void writePluginWidgets(ReportWriter writer) {
def widgets = widgets
def widgetNames = widgets.collect { it.name }.sort().join("")
def hash = Hashing.sha1().hashString(widgetNames, StandardCharsets.UTF_8).toString()
writer.write(new PluginData(WIDGETS_JSON, new Widgets(hash: hash, data: widgets)))
}
/**
* Write plugin resources. For each plugin search resources using
* {@link #findPluginResources(WithResources)}
*
* @see ReportWriter
*/
void writePluginResources(ReportWriter writer) {
pluginsWithResources.each { plugin ->
def resources = findPluginResources(plugin)
resources.each { resource ->
writer.write(plugin.name, resource)
}
}
}
/**
* Write plugins data to data directory.
*
* @see ReportWriter
*/
void writePluginData(ReportWriter writer) {
writer.write(pluginsData)
}
/**
* Find all resources for plugin.
*/
protected static List findPluginResources(WithResources plugin) {
def path = plugin.class.canonicalName.replace('.', '/')
def pattern = ~"^$path/.+\$"
def result = []
for (def resource : ClassPath.from(plugin.class.classLoader).resources) {
if (resource.resourceName =~ pattern) {
result.add(resource.url())
}
}
result
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy