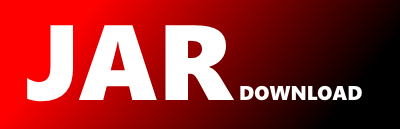
ru.yandex.qatools.allure.data.plugins.PluginManager.groovy Maven / Gradle / Ivy
package ru.yandex.qatools.allure.data.plugins
import com.google.inject.Inject
import com.google.inject.Injector
import ru.yandex.qatools.allure.data.io.ReportWriter
import ru.yandex.qatools.allure.data.utils.PluginUtils
/**
* @author Dmitry Baev [email protected]
* Date: 17.02.15
*/
class PluginManager {
protected Storage storage;
@Inject
PluginManager(PluginLoader loader, Injector injector = null) {
storage = new Storage(loader, injector)
}
public void prepare(T object) {
if (!object) {
return
}
def plugins = storage.get(object.class, PreparePlugin)
plugins.each {
it.prepare(object)
}
}
public void process(T object) {
if (!object) {
return
}
def plugins = storage.get(object.class, ProcessPlugin)
plugins.each {
it.process(PluginUtils.clone(object))
}
}
public void writePluginData(Class type, ReportWriter writer) {
writer.write(getData(type))
}
protected List getData(Class> type) {
if (!type) {
[]
}
storage.get(type, ProcessPlugin).collect {
it.pluginData
}.flatten()
}
protected class Storage {
private Map>> storage = new HashMap<>().withDefault {
new HashMap<>().withDefault {
new ArrayList<>()
}
}
Storage(PluginLoader loader, Injector injector) {
def plugins = loader.loadPlugins()
if (!plugins) {
return
}
plugins.each {
if (it) {
if (injector) {
injector.injectMembers(it)
}
put(it)
}
}
}
public > List get(Class objectType, Class pluginType) {
storage[objectType]?.get(pluginType) as List ?: []
}
public void put(Plugin plugin) {
def type = plugin.type
def classes = [ProcessPlugin, PreparePlugin]
classes.each {
if (it.isAssignableFrom(plugin.class)) {
storage[type][it].add(plugin)
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy