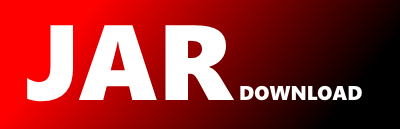
ru.yandex.qatools.htmlelements.element.Table Maven / Gradle / Ivy
package ru.yandex.qatools.htmlelements.element;
import ch.lambdaj.Lambda;
import ch.lambdaj.function.convert.Converter;
import org.openqa.selenium.By;
import org.openqa.selenium.WebElement;
import ru.yandex.qatools.htmlelements.exceptions.HtmlElementsException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import static ch.lambdaj.Lambda.convert;
import static ch.lambdaj.Lambda.convertMap;
import static ru.yandex.qatools.htmlelements.element.Table.ListConverter.toListsConvertingEachItem;
import static ru.yandex.qatools.htmlelements.element.Table.MapConverter.toMapsConvertingEachValue;
import static ru.yandex.qatools.htmlelements.element.Table.WebElementToTextConverter.toText;
import static ru.yandex.qatools.htmlelements.element.Table.WebElementToTextConverter.toTextValues;
/**
* Represents web page table element. Provides convenient ways of retrieving data stored in it.
*
* @author Alexander Tolmachev [email protected]
* Date: 11.03.13
*/
public class Table extends TypifiedElement {
/**
* Specifies {@link org.openqa.selenium.WebElement} representing table tag.
*
* @param wrappedElement {@code WebElement} to wrap.
*/
public Table(WebElement wrappedElement) {
super(wrappedElement);
}
/**
* Returns table heading elements (contained in "th" tags).
*
* @return List with table heading elements.
*/
public List getHeadings() {
return getWrappedElement().findElements(By.xpath(".//th"));
}
/**
* Returns text values of table heading elements (contained in "th" tags).
*
* @return List with text values of table heading elements.
*/
public List getHeadingsAsString() {
return convert(getHeadings(), toTextValues());
}
/**
* Returns table cell elements grouped by rows.
*
* @return List where each item is a table row.
*/
public List> getRows() {
List> rows = new ArrayList>();
List rowElements = getWrappedElement().findElements(By.xpath(".//tr"));
for (WebElement rowElement : rowElements) {
rows.add(rowElement.findElements(By.xpath(".//td")));
}
return rows;
}
/**
* Returns text values of table cell elements grouped by rows.
*
* @return List where each item is text values of a table row.
*/
public List> getRowsAsString() {
return convert(getRows(), toListsConvertingEachItem(toTextValues()));
}
/**
* Returns table cell elements grouped by columns.
*
* @return List where each item is a table column.
*/
public List> getColumns() {
List> columns = new ArrayList>();
List> rows = getRows();
if (rows.isEmpty()) {
return columns;
}
int columnsNumber = rows.get(0).size();
for (int i = 0; i < columnsNumber; i++) {
List column = new ArrayList();
for (List row : rows) {
column.add(row.get(i));
}
columns.add(column);
}
return columns;
}
/**
* Returns text values of table cell elements grouped by columns.
*
* @return List where each item is text values of a table column.
*/
public List> getColumnsAsString() {
return convert(getColumns(), toListsConvertingEachItem(toTextValues()));
}
/**
* Returns table cell element at i-th row and j-th column.
*
* @param i Row number
* @param j Column number
* @return Cell element at i-th row and j-th column.
*/
public WebElement getCellAt(int i, int j) {
return getRows().get(i).get(j);
}
/**
* Returns list of maps where keys are table headings and values are table row elements.
*/
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy