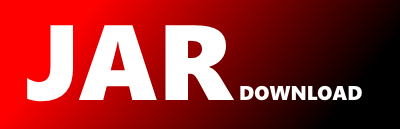
run.halo.app.extension.index.query.QueryIndexView Maven / Gradle / Ivy
Show all versions of api Show documentation
package run.halo.app.extension.index.query;
import java.util.List;
import java.util.NavigableSet;
import org.springframework.data.domain.Sort;
import run.halo.app.extension.Metadata;
import run.halo.app.extension.index.IndexEntry;
import run.halo.app.extension.index.IndexSpec;
/**
* A view of an index entries that can be queried.
* Explanation of naming:
*
* - fieldName: a field of an index, usually {@link IndexSpec#getName()}
* - fieldValue: a value of a field, e.g. a value of a field "name" could be "foo"
* - id: the id of an object pointing to object position, see {@link Metadata#getName()}
*
*
* @author guqing
* @since 2.12.0
*/
public interface QueryIndexView {
/**
* Gets all object ids for a given field name and field value.
*
* @param fieldName the field name
* @param fieldValue the field value
* @return all indexed object ids associated with the given field name and field value
* @throws IllegalArgumentException if the field name is not indexed
*/
NavigableSet findIds(String fieldName, String fieldValue);
/**
* Gets all object ids for a given field name without null cells.
*
* @param fieldName the field name
* @return all indexed object ids for the given field name
* @throws IllegalArgumentException if the field name is not indexed
*/
NavigableSet getIdsForField(String fieldName);
/**
* Gets all object ids in this view.
*
* @return all object ids in this view
*/
NavigableSet getAllIds();
/**
* Finds and returns a set of unique identifiers (metadata.name) for entries that have
* matching values across two fields and where the values are equal.
* For example:
*
* metadata.name | field1 | field2
* ------------- | ------ | ------
* foo | 1 | 1
* bar | 2 | 3
* baz | 3 | 3
*
* findMatchingIdsWithEqualValues("field1", "field2")
would return ["foo","baz"]
*
* @see #findMatchingIdsWithGreaterValues(String, String, boolean)
* @see #findMatchingIdsWithSmallerValues(String, String, boolean)
*/
NavigableSet findMatchingIdsWithEqualValues(String fieldName1, String fieldName2);
/**
* Finds and returns a set of unique identifiers (metadata.name) for entries that have
* matching values across two fields, but where the value associated with fieldName1 is
* greater than the value associated with fieldName2.
* For example:
*
* metadata.name | field1 | field2
* ------------- | ------ | ------
* foo | 1 | 1
* bar | 2 | 3
* baz | 3 | 3
* qux | 4 | 2
*
* findMatchingIdsWithGreaterValues("field1", "field2")
would return ["qux"]
* findMatchingIdsWithGreaterValues("field2", "field1")
would return ["bar"]
* findMatchingIdsWithGreaterValues("field1", "field2", true)
would return
* ["foo","baz","qux"]
*
* @param fieldName1 The field name whose values are compared as the larger values.
* @param fieldName2 The field name whose values are compared as the smaller values.
* @param orEqual whether to include equal values
* @return A result set of ids where the entries in fieldName1 have greater values than those
* in fieldName2 for entries that have the same id across both fields
*/
NavigableSet findMatchingIdsWithGreaterValues(String fieldName1, String fieldName2,
boolean orEqual);
NavigableSet findIdsGreaterThan(String fieldName, String fieldValue, boolean orEqual);
/**
* Finds and returns a set of unique identifiers (metadata.name) for entries that have
* matching values across two fields, but where the value associated with fieldName1 is
* less than the value associated with fieldName2.
* For example:
*
* metadata.name | field1 | field2
* ------------- | ------ | ------
* foo | 1 | 1
* bar | 2 | 3
* baz | 3 | 3
* qux | 4 | 2
*
* findMatchingIdsWithSmallerValues("field1", "field2")
would return ["bar"]
* findMatchingIdsWithSmallerValues("field2", "field1")
would return ["qux"]
* findMatchingIdsWithSmallerValues("field1", "field2", true)
would return
* ["foo","bar","baz"]
*
* @param fieldName1 The field name whose values are compared as the smaller values.
* @param fieldName2 The field name whose values are compared as the larger values.
* @param orEqual whether to include equal values
* @return A result set of ids where the entries in fieldName1 have smaller values than those
* in fieldName2 for entries that have the same id across both fields
*/
NavigableSet findMatchingIdsWithSmallerValues(String fieldName1, String fieldName2,
boolean orEqual);
NavigableSet findIdsLessThan(String fieldName, String fieldValue, boolean orEqual);
NavigableSet between(String fieldName, String lowerValue, boolean lowerInclusive,
String upperValue, boolean upperInclusive);
List sortBy(NavigableSet resultSet, Sort sort);
IndexEntry getIndexEntry(String fieldName);
/**
* Acquire a read lock on the indexer.
* if you need to operate on more than one {@code IndexEntry} at the same time, you need to
* lock first.
*
* @see #getIndexEntry(String)
*/
void acquireReadLock();
void releaseReadLock();
}