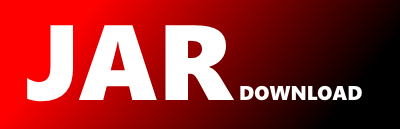
se.fortnox.reactivewizard.test.publisher.PublisherAssert Maven / Gradle / Ivy
package se.fortnox.reactivewizard.test.publisher;
import org.assertj.core.api.AbstractAssert;
import org.assertj.core.api.Assertions;
import org.assertj.core.api.ListAssert;
import org.assertj.core.api.ObjectAssert;
import org.reactivestreams.Publisher;
import reactor.core.publisher.Flux;
import reactor.test.StepVerifier;
import java.util.List;
/**
* Assertion for a Publisher.
*
* @param the type of the items emitted by the Publisher under test
*/
public class PublisherAssert extends AbstractAssert, Publisher> {
/**
* Default constructor.
*
* @param actual the Publisher under test
*/
public PublisherAssert(Publisher actual) {
super(actual, PublisherAssert.class);
isNotNull();
}
/**
* Verifies that the Publisher receives exactly the values given and nothing else in the same order.
*
* @param expected expected value
* @return a new assertion object whose object under test is a list of the expected values
*/
public ListAssert containsExactly(T... expected) {
StepVerifier.create(actual).expectNext(expected).verifyComplete();
return Assertions.assertThatList(List.of(expected));
}
/**
* Verifies that the Publisher receives a single event and no errors.
*
* @return a new assertion object whose object under test is the received event
*/
public ObjectAssert singleElement() {
List values = Flux.from(actual).collectList().block();
Assertions.assertThat(values)
.describedAs("Expected one value, but got %s.", values)
.hasSize(1);
return Assertions.assertThat(values.get(0));
}
/**
* Verifies that the Publisher emits no values.
*/
public void isEmpty() {
StepVerifier.create(actual).verifyComplete();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy