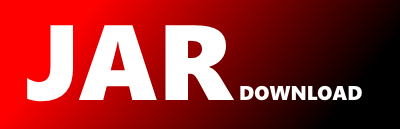
se.fortnox.reactivewizard.util.LambdaCompiler Maven / Gradle / Ivy
package se.fortnox.reactivewizard.util;
import java.lang.invoke.CallSite;
import java.lang.invoke.LambdaMetafactory;
import java.lang.invoke.MethodHandle;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.MethodType;
import java.util.function.BiConsumer;
import java.util.function.Function;
import java.util.function.Supplier;
public class LambdaCompiler {
static boolean useLambdas = "true".equals(System.getProperty("useLambdas", "true"));
public static Supplier compileLambdaSupplier(MethodHandles.Lookup lookup, MethodHandle methodHandle) throws Throwable {
if (!useLambdas) {
return () -> {
try {
return (T) methodHandle.invoke();
} catch (Throwable e) {
throw new RuntimeException(e);
}
};
}
CallSite callSite = LambdaMetafactory.metafactory(
lookup,
"get",
MethodType.methodType(Supplier.class),
MethodType.methodType(Object.class),
methodHandle,
methodHandle.type()
);
return (Supplier)callSite.getTarget().invoke();
}
public static BiConsumer compileLambdaBiConsumer(MethodHandles.Lookup lookup, MethodHandle methodHandle) throws Throwable {
if (!useLambdas) {
return (instance, arg) -> {
try {
methodHandle.bindTo(instance).invokeWithArguments(arg);
} catch (Throwable e) {
throw new RuntimeException(e);
}
};
}
CallSite callSite = LambdaMetafactory.metafactory(
lookup,
"accept",
MethodType.methodType(BiConsumer.class),
MethodType.methodType(void.class, Object.class, Object.class),
methodHandle,
methodHandle.type().wrap().changeReturnType(void.class)
);
return (BiConsumer) callSite.getTarget().invoke();
}
public static Function compileLambdaFunction(MethodHandles.Lookup lookup, MethodHandle methodHandle) throws Throwable {
if (!useLambdas) {
return (instance) -> {
try {
return (T)methodHandle.bindTo(instance).invoke();
} catch (Throwable e) {
throw new RuntimeException(e);
}
};
}
CallSite callSite = LambdaMetafactory.metafactory(
lookup,
"apply",
MethodType.methodType(Function.class),
MethodType.methodType(Object.class, Object.class),
methodHandle,
methodHandle.type()
);
return (Function) callSite.getTarget().invoke();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy