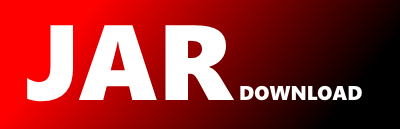
se.fortnox.reactivewizard.util.rx.FirstThen Maven / Gradle / Ivy
package se.fortnox.reactivewizard.util.rx;
import rx.Observable;
import rx.functions.Func0;
import static rx.Observable.just;
/**
* Helper class used to chain sequential work in Rx, or omit foo variables. Turns code like this:
*
*
* {@code
* doStuff().flatMap(foo->empty());
* }
*
* Into this:
*
*
* {@code
* first(doStuff()).thenReturnEmpty();
* }
*
*/
public class FirstThen {
private Observable> doFirst;
private FirstThen(Observable> doFirst) {
this.doFirst = doFirst;
}
public static FirstThen first(Observable> doFirst) {
return new FirstThen(doFirst);
}
@SuppressWarnings("unchecked")
private static Observable ignoreElements(Observable> toConsume) {
return (Observable)toConsume.ignoreElements();
}
public FirstThen then(Observable thenReturn) {
return new FirstThen(ignoreElements(doFirst).concatWith(thenReturn));
}
public FirstThen then(Func0> thenFn) {
return new FirstThen(ignoreElements(doFirst).concatWith(Observable.defer(thenFn)));
}
public Observable thenReturn(Func0> thenFn) {
return thenReturn(Observable.defer(thenFn));
}
public Observable thenReturn(T thenReturn) {
return thenReturn(just(thenReturn));
}
public Observable thenReturn(Observable thenReturn) {
return FirstThen.ignoreElements(doFirst).concatWith(thenReturn);
}
/**
* An empty observable, signalling success or error.
*/
public Observable thenReturnEmpty() {
return thenReturn(Observable.empty());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy