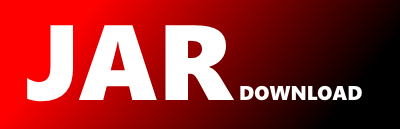
se.gawell.fakeriak.index.InMemoryIndexes Maven / Gradle / Ivy
The newest version!
package se.gawell.fakeriak.index;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentLinkedQueue;
import org.codehaus.jackson.map.ObjectMapper;
public class InMemoryIndexes implements Indexes {
private ConcurrentHashMap>>> indexBucketMap = new ConcurrentHashMap>>>();
@Override
public String getKeysAsJsonString(String bucketName, String indexName, String indexValueA, String indexValueB) {
Set bucketIndexValueList = new HashSet();
if (indexValueB == null) {
indexValueB = indexValueA;
}
for (String indexValue : getBucketIndex(bucketName, indexName).keySet()) {
if (indexValueA.compareTo(indexValue) <= 0 && indexValueB.compareTo(indexValue) >= 0) {
ConcurrentLinkedQueue valuesForKey = getBucketIndexValueSet(bucketName, indexName, indexValue);
bucketIndexValueList.addAll(valuesForKey);
}
}
List sortedBucketIndexValueList = new ArrayList(bucketIndexValueList.size());
sortedBucketIndexValueList.addAll(bucketIndexValueList);
Collections.sort(sortedBucketIndexValueList);
ObjectMapper objectMapper = new ObjectMapper();
try {
return objectMapper.writeValueAsString(new KeyList(sortedBucketIndexValueList));
} catch (Exception e) {
throw new RuntimeException(e);
}
}
@Override
public void put(String bucketName, String indexName, String indexValue, String key) {
ConcurrentLinkedQueue bucketIndexValueSet = getBucketIndexValueSet(bucketName, indexName, indexValue);
bucketIndexValueSet.add(key);
}
@Override
public void deleteKey(String bucketName, String key) {
ConcurrentHashMap>> bucket = getBucket(bucketName);
for (ConcurrentHashMap> indexValues: bucket.values()) {
for (String indexNames: indexValues.keySet()) {
ConcurrentLinkedQueue keysInIndex = indexValues.get(indexNames);
keysInIndex.remove(key);
}
}
}
private ConcurrentLinkedQueue getBucketIndexValueSet(String bucketName, String indexName, String indexValue) {
final ConcurrentHashMap> bucketIndex = getBucketIndex(bucketName,
indexName);
ConcurrentLinkedQueue indexSet = bucketIndex.get(indexValue);
if (indexSet == null) {
indexSet = new ConcurrentLinkedQueue();
bucketIndex.put(indexValue, indexSet);
}
return indexSet;
}
private ConcurrentHashMap> getBucketIndex(String bucketName, String indexName) {
final ConcurrentHashMap>> bucket = getBucket(bucketName);
ConcurrentHashMap> index = bucket.get(indexName);
if (index == null) {
index = new ConcurrentHashMap>();
bucket.put(indexName, index);
}
return index;
}
private ConcurrentHashMap>> getBucket(
String bucketName) {
ConcurrentHashMap>> indexBucket = indexBucketMap
.get(bucketName);
if (indexBucket == null) {
indexBucket = new ConcurrentHashMap>>();
indexBucketMap.put(bucketName, indexBucket);
}
return indexBucket;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy