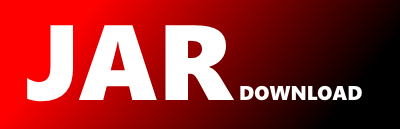
se.hiq.oss.json.schema.repo.JsonSchemaRepositoryImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of json-schema-discovery Show documentation
Show all versions of json-schema-discovery Show documentation
Auto-detected JSON schema classes and provide a repository for easy lookup
package se.hiq.oss.json.schema.repo;
import java.io.IOException;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import org.springframework.core.annotation.AnnotationUtils;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.github.fge.jackson.JsonLoader;
import com.github.fge.jsonschema.core.exceptions.ProcessingException;
import com.github.fge.jsonschema.main.JsonSchema;
import com.github.fge.jsonschema.main.JsonSchemaFactory;
import se.hiq.oss.json.schema.validation.impl.JsonSchemaNodeValidatorImpl;
import se.hiq.oss.json.schema.validation.impl.JsonSchemaStringValidatorImpl;
public class JsonSchemaRepositoryImpl implements JsonSchemaRepository {
private Map> schemas = new HashMap<>();
private ObjectMapper objectMapper;
public JsonSchemaRepositoryImpl(final ObjectMapper objectMapper) {
this.objectMapper = objectMapper;
}
@Override
public Optional getSchemaRegistration(String name, String version) {
Map schemasPerName = schemas.get(name);
if (schemasPerName != null) {
return Optional.ofNullable(schemasPerName.get(version));
}
return Optional.empty();
}
@Override
public boolean isSupported(String name, String version) {
Map schemasPerName = schemas.get(name);
if (schemasPerName != null) {
return schemasPerName.get(version) != null;
}
return false;
}
@Override
public Set getSupportedVersions(String name) {
Map schemasPerName = schemas.get(name);
if (schemasPerName != null) {
return schemasPerName.keySet();
}
return Collections.EMPTY_SET;
}
@Override
public void registerSchemaFor(Class> serDeClass) {
se.hiq.oss.json.schema.JsonSchema annotation =
AnnotationUtils.findAnnotation(serDeClass,
se.hiq.oss.json.schema.JsonSchema.class);
if (annotation != null) {
Map schemasPerName = schemas.get(annotation.name());
if (schemasPerName == null) {
schemasPerName = new HashMap<>();
schemas.put(annotation.name(), schemasPerName);
}
checkDuplicatedSchema(serDeClass, annotation, schemasPerName);
JsonSchemaRegistration registration = createRegistration(serDeClass, annotation);
schemasPerName.put(annotation.version(), registration);
}
}
private JsonSchemaRegistration createRegistration(
Class> serDeClass,
se.hiq.oss.json.schema.JsonSchema annotation) {
JsonSchema schema = loadSchema(annotation);
JsonSchemaStringValidatorImpl stringValidator = new JsonSchemaStringValidatorImpl(objectMapper);
stringValidator.initialize(serDeClass);
JsonSchemaNodeValidatorImpl nodeValidator = new JsonSchemaNodeValidatorImpl();
nodeValidator.initialize(serDeClass);
return new JsonSchemaRegistrationImpl(serDeClass, schema, stringValidator, nodeValidator);
}
private JsonSchema loadSchema(se.hiq.oss.json.schema.JsonSchema annotation) {
try {
return JsonSchemaFactory.byDefault().getJsonSchema(JsonLoader.fromResource(annotation.location()));
} catch (IOException | ProcessingException e) {
throw new IllegalStateException("Could not load JSON schema "
+ annotation.name() + " version " + annotation.version(), e);
}
}
private void checkDuplicatedSchema(Class> serDeClass,
se.hiq.oss.json.schema.JsonSchema annotation,
Map schemasPerName) {
if (schemasPerName.containsKey(annotation.version())) {
JsonSchemaRegistration otherSchema = schemasPerName.get(annotation.version());
throw new DuplicateJsonSchemaException("Duplicate classes are annotated as the same version for schema "
+ annotation.name() + " and version " + annotation.version() + " both "
+ otherSchema.getSerDeClass() + " and " + serDeClass);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy