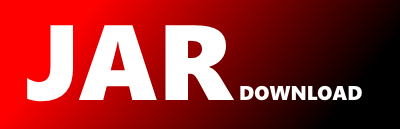
se.hiq.oss.json.schema.validation.impl.AbstractJsonValidator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of json-schema-discovery Show documentation
Show all versions of json-schema-discovery Show documentation
Auto-detected JSON schema classes and provide a repository for easy lookup
package se.hiq.oss.json.schema.validation.impl;
import java.io.IOException;
import javax.validation.ConstraintValidatorContext;
import org.springframework.core.annotation.AnnotationUtils;
import com.fasterxml.jackson.databind.JsonNode;
import com.github.fge.jackson.JsonLoader;
import com.github.fge.jsonschema.core.exceptions.ProcessingException;
import com.github.fge.jsonschema.core.report.ProcessingReport;
import com.github.fge.jsonschema.main.JsonSchema;
import com.github.fge.jsonschema.main.JsonSchemaFactory;
import se.hiq.oss.json.schema.validation.ValidJson;
public class AbstractJsonValidator {
private String name;
private String version;
private JsonSchema jsonSchema;
public void initialize(final Class> serDeClass) {
se.hiq.oss.json.schema.JsonSchema jsonSchemaAnnotation =
AnnotationUtils.findAnnotation(serDeClass,
se.hiq.oss.json.schema.JsonSchema.class);
if (jsonSchemaAnnotation != null) {
jsonSchema = loadSchema(jsonSchemaAnnotation);
name = jsonSchemaAnnotation.name();
version = jsonSchemaAnnotation.version();
} else {
throw new IllegalArgumentException("Class " + serDeClass.getName() + " must be annotated with @"
+ se.hiq.oss.json.schema.JsonSchema.class.getName());
}
}
public void initialize(final ValidJson annotation) {
initialize(annotation.jsonSchemaClass());
}
private JsonSchema loadSchema(se.hiq.oss.json.schema.JsonSchema annotation) {
try {
return JsonSchemaFactory.byDefault().getJsonSchema(JsonLoader.fromResource(annotation.location()));
} catch (IOException | ProcessingException e) {
throw new IllegalStateException("Could not load JSON schema for "
+ annotation.name() + " version " + annotation.version() + " from " + annotation.location(), e);
}
}
protected ProcessingReport isJsonValid(JsonNode jsonNode) {
try {
return jsonSchema.validate(jsonNode);
} catch (ProcessingException e) {
throw new IllegalStateException("Could not process JSON schema '" + name + "' version '" + version + "'", e);
}
}
private void addConstraints(ProcessingReport processingReport, ConstraintValidatorContext context) {
context.buildConstraintViolationWithTemplate("JSON does not comply to schema '" + name
+ "' version '" + version + "'")
.addConstraintViolation();
processingReport.forEach(m -> context.buildConstraintViolationWithTemplate(m.asJson().toString())
.addConstraintViolation());
}
protected String toErrorMessage(ProcessingReport processingReport) {
StringBuilder stringBuilder = new StringBuilder();
processingReport.forEach(m -> stringBuilder.append(m.getMessage() + ": " + m.asJson()).append('\n'));
return "JSON does not comply to schema '" + name + "' version '" + version + "': " + stringBuilder.toString();
}
protected void setErrorConstraint(ProcessingReport processingReport, ConstraintValidatorContext context) {
context.disableDefaultConstraintViolation();
addConstraints(processingReport, context);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy