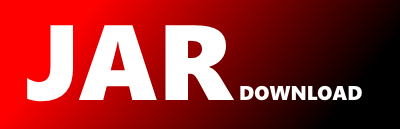
se.jbee.inject.Array Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of silk-di Show documentation
Show all versions of silk-di Show documentation
Silk Java dependency injection framework
/*
* Copyright (c) 2012, Jan Bernitt
*
* Licensed under the Apache License, Version 2.0, http://www.apache.org/licenses/LICENSE-2.0
*/
package se.jbee.inject;
import java.util.Arrays;
import java.util.Collection;
/**
* Silks array util.
*
* @author Jan Bernitt ([email protected])
*/
public final class Array {
public static T[] append( T[] array, T value ) {
T[] copy = Arrays.copyOf( array, array.length + 1 );
copy[array.length] = value;
return copy;
}
@SuppressWarnings ( "unchecked" )
public static T[] prepand( T value, T[] array ) {
T[] copy = (T[]) newInstance( array.getClass().getComponentType(), array.length + 1 );
System.arraycopy( array, 0, copy, 1, array.length );
copy[0] = value;
return copy;
}
@SuppressWarnings ( "unchecked" )
public static T[] newInstance( Class componentType, int length ) {
return (T[]) java.lang.reflect.Array.newInstance( componentType, length );
}
@SuppressWarnings ( "unchecked" )
public static T[] fill( T value, int length ) {
T[] res = (T[]) newInstance( value.getClass(), length );
for ( int i = 0; i < res.length; i++ ) {
res[i] = value;
}
return res;
}
public static T[] of( Collection extends T> list, Class type ) {
return list.toArray( newInstance( type, list.size() ) );
}
@SuppressWarnings ( "unchecked" )
public static T[] of( Collection extends T> list, T[] empty ) {
if ( list.isEmpty() ) {
return empty;
}
return of( list, (Class) empty.getClass().getComponentType() );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy