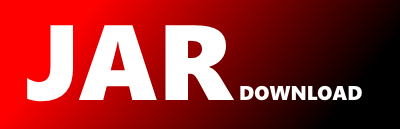
sorald.event.models.repair.RuleRepairStatistics Maven / Gradle / Ivy
package sorald.event.models.repair;
import java.nio.file.Path;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.stream.Collectors;
import sorald.event.collectors.RepairStatisticsCollector;
import sorald.event.models.RepairEvent;
import sorald.event.models.WarningLocation;
import sorald.event.models.miner.MinedViolationEvent;
import sorald.sonar.SonarRule;
/** Repair statistics for a single rule. */
public class RuleRepairStatistics {
private final String ruleKey;
private final String ruleName;
private final List violationsBefore;
private final List violationsAfter;
private final List performedRepairsLocations;
private final List crashedRepairsLocations;
public RuleRepairStatistics(
String ruleKey,
String ruleName,
List violationsBefore,
List violationsAfter,
List repairedViolations,
List failedRepairs,
Path projectPath) {
this.ruleKey = ruleKey;
this.ruleName = ruleName;
this.violationsBefore = new ArrayList<>(violationsBefore);
this.violationsAfter = new ArrayList<>(violationsAfter);
this.performedRepairsLocations = toWarningLocations(repairedViolations, projectPath);
this.crashedRepairsLocations = toWarningLocations(failedRepairs, projectPath);
}
private static List toWarningLocations(
List repairEvents, Path projectPath) {
return repairEvents.stream()
.map(
repairEvent ->
new WarningLocation(repairEvent.getRuleViolation(), projectPath))
.collect(Collectors.toList());
}
public String getRuleKey() {
return ruleKey;
}
public String getRuleName() {
return ruleName;
}
public List getPerformedRepairsLocations() {
return Collections.unmodifiableList(performedRepairsLocations);
}
public List getCrashedRepairsLocations() {
return Collections.unmodifiableList(crashedRepairsLocations);
}
public int getNbViolationsBefore() {
return violationsBefore.size();
}
public int getNbViolationsAfter() {
return violationsAfter.size();
}
public int getNbPerformedRepairs() {
return performedRepairsLocations.size();
}
public int getNbCrashedRepairs() {
return crashedRepairsLocations.size();
}
/**
* Convert a repair statistics collector into a list of repair statistics containers designed
* for pretty JSON output.
*
* @param statsCollector A statistics collector for the repair mode.
* @param projectPath Path to the project.
* @return A list of repair statistics container, one for each rule.
*/
public static List createRepairStatsList(
RepairStatisticsCollector statsCollector, Path projectPath) {
Map> keyToRepair = statsCollector.performedRepairs();
Map> keyToFailure = statsCollector.crashedRepairs();
Map> keyToViolationsBefore =
statsCollector.minedViolationsBefore();
Map> keyToViolationsAfter =
statsCollector.minedViolationsAfter();
Set distinctKeys = new HashSet<>(keyToRepair.keySet());
distinctKeys.addAll(keyToFailure.keySet());
return distinctKeys.stream()
.map(
key -> {
List repairs = keyToRepair.getOrDefault(key, List.of());
List failures = keyToFailure.getOrDefault(key, List.of());
List violationsBefore =
keyToViolationsBefore.getOrDefault(key, List.of());
List violationsAfter =
keyToViolationsAfter.getOrDefault(key, List.of());
String ruleName = new SonarRule(key).getName();
return new RuleRepairStatistics(
key,
ruleName,
violationsBefore,
violationsAfter,
repairs,
failures,
projectPath);
})
.collect(Collectors.toList());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy